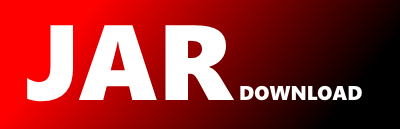
it.tidalwave.metadata.persistence.spi.MetadataPersistenceSupport Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* blueMarine Metadata - open source media workflow
* Copyright (C) 2007-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluemarine.tidalwave.it
* SCM: https://kenai.com/hg/bluemarine~metadata-src
*
**********************************************************************************************************************/
package it.tidalwave.metadata.persistence.spi;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.NoSuchElementException;
import it.tidalwave.util.logging.Logger;
import org.openide.util.Lookup;
import it.tidalwave.metadata.spi.ClassMap;
import it.tidalwave.metadata.persistence.MetadataPropertySet;
import it.tidalwave.metadata.persistence.event.MetadataPersistenceEvent;
import it.tidalwave.metadata.persistence.event.MetadataPersistenceListener;
/*******************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
******************************************************************************/
public abstract class MetadataPersistenceSupport implements MetadataPersistenceSpi
{
private final static String CLASS = MetadataPersistenceSupport.class.getName();
private final static Logger logger = Logger.getLogger(CLASS);
private final ClassMap accessorMapByClass = new ClassMap();
private final Map> classMapByGroupName = new HashMap>();
private final ClassMap propertySetNameMapByClass = new ClassMap();
/***************************************************************************
*
**************************************************************************/
public final void register (@Nonnull final Class beanClass,
@Nonnull final BeanAccessor beanAccessor)
{
final String propertySetName = beanAccessor.getPropertySetName();
logger.info("register(%s, %s, %s)", beanClass, propertySetName, beanAccessor);
classMapByGroupName.put(propertySetName, beanClass);
propertySetNameMapByClass.put(beanClass, propertySetName);
accessorMapByClass.put(beanClass, beanAccessor);
logger.info(">>>> classMapByGroupName: %s", classMapByGroupName);
logger.info(">>>> propertySetNameMapByClass: %s", propertySetNameMapByClass);
logger.info(">>>> accessorMapByClass: %s", accessorMapByClass);
}
/***************************************************************************
*
* {@inheritDoc}
*
**************************************************************************/
@Nonnull
public MetadataPropertySet findPropertySetByClass (@Nonnull final Class> beanClass)
{
for (final MetadataPropertySet set : getPropertySets())
{
if (set.getItemClass().equals(beanClass))
{
return set;
}
}
throw new NoSuchElementException(beanClass.getName());
}
/***************************************************************************
*
* {@inheritDoc}
*
**************************************************************************/
@CheckForNull
public final Class> findBeanClassByPropertySetName (@Nonnull final String groupName)
{
if (groupName == null)
{
throw new IllegalArgumentException("groupName is mandatory");
}
return classMapByGroupName.get(groupName);
}
/***************************************************************************
*
* {@inheritDoc}
*
**************************************************************************/
@CheckForNull
public final String findPropertySetNameByBeanClass (@Nonnull final Class> beanClass)
{
if (beanClass == null)
{
throw new IllegalArgumentException("beanClass is mandatory");
}
return propertySetNameMapByClass.get(beanClass);
}
/***************************************************************************
*
* {@inheritDoc}
*
**************************************************************************/
@CheckForNull
public final BeanAccessor findAccessorByClass (@Nonnull final Class> beanClass)
{
if (beanClass == null)
{
throw new IllegalArgumentException("beanClass is mandatory");
}
return accessorMapByClass.get(beanClass);
}
/***************************************************************************
*
**************************************************************************/
protected final void fireNotifyPropertyPersisted (@Nonnull final Class> propertySetClass,
@Nonnull final String propertyName,
@CheckForNull final Object value)
{
final Collection extends MetadataPersistenceListener> listeners =
Lookup.getDefault().lookupAll(MetadataPersistenceListener.class);
logger.fine("fireNotifyPropertyPersisted(%s, %s, %s) - to %d listeners",
propertySetClass, propertyName, value, listeners.size());
if (!listeners.isEmpty())
{
final MetadataPersistenceEvent event = new MetadataPersistenceEvent(this, propertySetClass, propertyName, value);
for (final MetadataPersistenceListener listener : listeners)
{
try
{
listener.notifyPropertyPersisted(event);
}
catch (Throwable t)
{
logger.throwing(CLASS, "fireNotifyPropertyPersisted", t);
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy