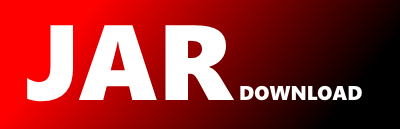
it.tidalwave.metadata.persistence.spi.MistralBeanAccessor Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* blueMarine Metadata - open source media workflow
* Copyright (C) 2007-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluemarine.tidalwave.it
* SCM: https://kenai.com/hg/bluemarine~metadata-src
*
**********************************************************************************************************************/
package it.tidalwave.metadata.persistence.spi;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import java.lang.reflect.Method;
import java.beans.IntrospectionException;
import java.beans.PropertyDescriptor;
import java.util.ArrayList;
import java.util.List;
import org.jdesktop.beansbinding.Property;
import it.tidalwave.beans.FastBeanProperty;
/*******************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
******************************************************************************/
public class MistralBeanAccessor extends IntrospectionBeanAccessor
{
/***************************************************************************
*
* Creates this object passing the class of the represented item and the name
* of the property set.
*
* @param itemClass the class of the represented item
* @param propertySetName the name of the property set
*
**************************************************************************/
public MistralBeanAccessor (@Nonnull final Class beanClass, @Nonnull final String propertySetName)
{
super(beanClass, propertySetName);
}
/***************************************************************************
*
* {@inheritDoc}. The returned properties are those discoverable by
* introspection, for which {@link #isPersistent(String)} returns true and
* having a sister property isXXXAvailable
whose value is
* true
.
*
**************************************************************************/
@Nonnull
public List getPersistentPropertyNames (@Nonnull final T item)
{
final List result = new ArrayList();
try
{
for (final PropertyDescriptor descriptor : findPropertyDescriptors(item))
{
final String propertyName = descriptor.getName();
if (!propertyName.endsWith("Available") && isPersistent(propertyName))
{
final Property availabilityProperty = FastBeanProperty.create(propertyName + "Available");
if (availabilityProperty.isReadable(item) && (Boolean)availabilityProperty.getValue(item))
{
result.add(propertyName);
}
}
}
}
catch (IntrospectionException e)
{
e.printStackTrace(); // FIXME: use Logger
}
return result;
}
/***************************************************************************
*
* {@inheritDoc}. This method provides support for enumeration types by
* returning Integer.class
for them.
*
**************************************************************************/
@Override
@Nonnull
public Class> getDefaultPropertyType (@Nonnull final String propertyName)
throws UnknownPropertyException
{
if (propertyName == null)
{
throw new IllegalArgumentException("propertyName is mandatory");
}
final Class> type = super.getDefaultPropertyType(propertyName);
return type.isEnum() ? Integer.class : type;
}
/***************************************************************************
*
* {@inheritDoc}. This method provides for converting enumeration types by
* calling the static method getValue()
.
*
**************************************************************************/
@Override
@CheckForNull
public Object convertValueToPersistence (@Nonnull final String propertyName,
@CheckForNull final Object value)
throws UnknownPropertyException
{
final Class> type = super.getDefaultPropertyType(propertyName);
if (!type.isEnum())
{
return value;
}
else
{
try
{
final Method method = type.getMethod("getValue");
return method.invoke(value);
}
catch (Exception e)
{
throw new RuntimeException(e);
}
}
}
/***************************************************************************
*
* {@inheritDoc}. This method provides for converting enumeration types by
* calling the static method fromInteger()
.
*
**************************************************************************/
@Override
@CheckForNull
public Object convertValueFromPersistence (@Nonnull final String propertyName,
@CheckForNull Object value)
throws UnknownPropertyException
{
final Class> type = super.getDefaultPropertyType(propertyName);
if (!type.isEnum())
{
return value;
}
else
{
if (!value.getClass().equals(Integer.class))
{
value = Integer.valueOf(((Number)value).intValue());
System.err.println(String.format("Property %s, enum type is not Integer: %s", propertyName, value.getClass()));
}
try
{
final Method method = type.getMethod("fromInteger", int.class);
return method.invoke(null, value);
}
catch (RuntimeException e)
{
throw e;
}
catch (Exception e)
{
throw new RuntimeException(e);
}
}
}
/***************************************************************************
*
* {@inheritDoc}.
*
**************************************************************************/
@Override
@Nonnull
public String toString()
{
return String.format("MistralBeanAccessor[%s]", getPropertySetName());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy