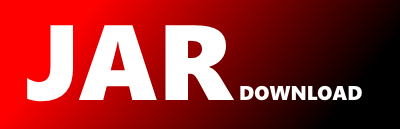
it.tidalwave.metadata.tiff.provider.DirectoryFormatProviderSupport Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* blueMarine Metadata - open source media workflow
* Copyright (C) 2007-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluemarine.tidalwave.it
* SCM: https://kenai.com/hg/bluemarine~metadata-src
*
**********************************************************************************************************************/
package it.tidalwave.metadata.tiff.provider;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
import java.util.MissingResourceException;
import java.text.FieldPosition;
import java.text.Format;
import java.text.ParsePosition;
import it.tidalwave.image.Rational;
import org.openide.util.NbBundle;
import it.tidalwave.metadata.spi.MetadataItemFormatProvider;
class RationalFormatWrapper extends Format
{
private static final long serialVersionUID = 6546987245982745984L;
@Nonnull
private final Format delegate;
public RationalFormatWrapper (@Nonnull final Format delegate)
{
this.delegate = delegate;
}
@Override
public StringBuffer format (@Nonnull Object object,
@Nonnull final StringBuffer toAppendTo,
@Nonnull final FieldPosition position)
{
if (object instanceof Rational)
{
object = ((Rational)object).doubleValue();
}
return delegate.format(object, toAppendTo, position);
}
@Override
public Object parseObject (@Nonnull final String source,
@Nonnull final ParsePosition pos)
{
throw new UnsupportedOperationException("Not supported yet.");
}
@Nonnull
protected Format getDelegate()
{
return delegate;
}
@Override
public String toString()
{
return String.format("RationalFormatWrapper[%s]", delegate);
}
}
/*******************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
******************************************************************************/
public class DirectoryFormatProviderSupport- implements MetadataItemFormatProvider
-
{
protected final Map
formatMap = new HashMap();
private final Class- itemClass;
private final String displayName;
// FIXME: merge with MetadataItemFormatImpl
protected final static Format DEFAULT_FORMAT = new Format()
{
@Override
@Nonnull
public StringBuffer format (@CheckForNull final Object object,
@Nonnull final StringBuffer toAppendTo,
@Nonnull final FieldPosition pos)
{
toAppendTo.append((object != null) ? object.toString() : "");
return toAppendTo;
}
@Override
@Nonnull
public Object parseObject (@Nonnull final String source,
@Nonnull final ParsePosition pos)
{
pos.setIndex(pos.getIndex() + source.length() + 1);
return source;
}
};
public DirectoryFormatProviderSupport (@Nonnull final Class
- itemClass,
@Nonnull final String displayName)
{
this.itemClass = itemClass;
this.displayName = displayName;
}
@Nonnull
public Class
- getItemClass()
{
return itemClass;
}
@Nonnull
public final String getItemDisplayName()
{
return displayName;
}
@Nonnull
public Format getFormat (@Nonnull final String propertyName)
{
Format format = formatMap.get(propertyName);
if (format == null)
{
format = DEFAULT_FORMAT;
}
return new RationalFormatWrapper(format);
}
@Nonnull
public String getDisplayName (@Nonnull final String propertyName)
{
//
// Try Bundle.properties of the current class and superclasses up to
// this class, then give up.
//
for (Class> clazz = getClass();
!clazz.equals(DirectoryFormatProviderSupport.class.getSuperclass());
clazz = clazz.getSuperclass())
{
try
{
return NbBundle.getMessage(clazz, normalized(propertyName));
}
catch (MissingResourceException e)
{
// ok, try next one
}
}
return propertyName;
}
protected String normalized (@Nonnull final String string)
{
return capitalized(string);
}
@Nonnull
private static String capitalized (@Nonnull final String string)
{
return string.substring(0, 1).toUpperCase(Locale.getDefault()) + string.substring(1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy