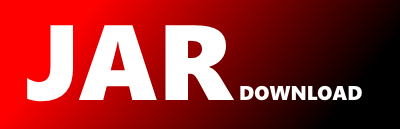
it.tidalwave.metadata.viewer.MetadataPanelProviderSupport Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* blueMarine Metadata - open source media workflow
* Copyright (C) 2007-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluemarine.tidalwave.it
* SCM: https://kenai.com/hg/bluemarine~metadata-src
*
**********************************************************************************************************************/
package it.tidalwave.metadata.viewer;
import it.tidalwave.metadata.NoSuchMetadataTypeException;
import it.tidalwave.util.logging.Logger;
import javax.swing.JComponent;
import org.openide.loaders.DataObject;
import it.tidalwave.metadata.Metadata;
import it.tidalwave.metadata.MetadataItemHolder;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
/*******************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
******************************************************************************/
public abstract class MetadataPanelProviderSupport, Item> implements MetadataPanelProvider
{
private final static String CLASS = MetadataPanelProviderSupport.class.getName();
private final Logger logger = Logger.getLogger(CLASS);
@CheckForNull
protected Pane pane;
@Nonnull
private final Class extends Pane> paneClass;
@Nonnull
private final Class extends Item> itemClass;
@Nonnull
private final String displayName;
@CheckForNull
private DataObject dataObject;
private final Metadata.StorageType sourceType = Metadata.StorageType.INTERNAL; // FIXME: make it changeable
/***************************************************************************
*
*
**************************************************************************/
public MetadataPanelProviderSupport (@Nonnull final String displayName,
@Nonnull final Class extends Pane> paneClass,
@Nonnull final Class extends Item> itemClass)
{
this.displayName = displayName;
this.paneClass = paneClass;
this.itemClass = itemClass;
}
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public String getDisplayName()
{
return displayName;
}
/***************************************************************************
*
*
**************************************************************************/
abstract public boolean isDataObjectSupported (@Nonnull final DataObject dataObject);
/***************************************************************************
*
*
**************************************************************************/
@Nonnull
public synchronized JComponent createPanel()
{
if (pane == null)
{
try
{
pane = paneClass.newInstance();
}
catch (InstantiationException e)
{
throw new RuntimeException(e);
}
catch (IllegalAccessException e)
{
throw new RuntimeException(e);
}
}
return (JComponent)pane;
}
/***************************************************************************
*
*
**************************************************************************/
public void setDataObject (@CheckForNull final DataObject dataObject)
{
logger.info("setDataObject(%s)", dataObject);
final long time = System.currentTimeMillis();
if (pane != null)
{
this.dataObject = dataObject;
if (dataObject != null)
{
final Metadata metadata = dataObject.getLookup().lookup(Metadata.class);
logger.fine(">>>> metadata: %s", metadata);
if (metadata != null)
{
// RequestProcessor.getDefault().post(new Runnable()
// {
// public void run()
{
try
{
final MetadataItemHolder extends Item> holder = metadata.findOrCreateItem(itemClass, sourceType);
logger.fine(">>>> holder: %s", holder);
pane.bind(holder);
}
catch (NoSuchMetadataTypeException e)
{
logger.severe(String.format("Could not bind item, because of %s", e));
}
catch (IllegalArgumentException e)
{
logger.severe(String.format("Could not bind item, because of %s", e));
}
logger.fine(">>>> setDataObject() completed in %d msec", System.currentTimeMillis() - time);
}// });
}
}
}
}
/***************************************************************************
*
*
**************************************************************************/
@CheckForNull
public DataObject getDataObject()
{
return dataObject;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy