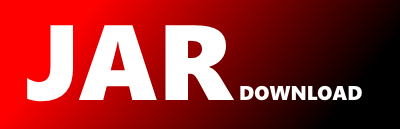
it.tidalwave.netbeans.role.provider.RoleLookupFactory Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* OpenBlueSky - NetBeans Platform Enhancements
* Copyright (C) 2006-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://openbluesky.java.net
* SCM: https://bitbucket.org/tidalwave/openbluesky-src
*
**********************************************************************************************************************/
package it.tidalwave.netbeans.role.provider;
import javax.annotation.Nonnull;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import lombok.extern.slf4j.Slf4j;
import org.openide.util.Lookup;
import org.openide.util.lookup.Lookups;
import org.openide.util.lookup.ProxyLookup;
import it.tidalwave.netbeans.capabilitiesprovider.CapabilitiesProvider;
/***********************************************************************************************************************
*
* A utility class for creating a {@link Lookup} containing roles for am {@link Object}. A typical example of
* use is:
*
*
* public class MyStuff implements Lookup.Provider
* {
* @CheckForNull
* private Lookup lookup;
*
* @Override @Nonnull
* public synchronized Lookup getLookup()
* {
* if (lookup == null)
* {
* lookup = LookupFactory.createLookup(this);
* }
*
* return lookup;
* }
*
* ...
* }
*
*
* If you need to, you can customize your {@code Lookup}:
*
*
* public class MyStuff implements Lookup.Provider
* {
* @CheckForNull
* private Lookup lookup;
*
* @Override @Nonnull
* public synchronized Lookup getLookup()
* {
* if (lookup == null)
* {
* Lookup myOtherLookup = Lookups.fixed(...);
* Lookup yetAnotherLookup = ...;
* lookup = LookupFactory.createLookup(this, myOtherLookup, yetAnotherLookup);
* }
*
* return lookup;
* }
*
* ...
* }
*
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
@Slf4j
public class RoleLookupFactory
{
/*******************************************************************************************************************
*
* Create a {@link Lookup} that contains the capabilities of the owner object, retrieved by looking up one or more
* {@link CapabilitiesProvider}s. It is possible to pass an optional set of extra {@code Lookup} instances that will
* be joined together.
*
* @param owner the owner
* @param extraLookups the extra Lookup
s
* @return the Lookup
for the owner
*
******************************************************************************************************************/
@Nonnull
public static Lookup createLookup (final @Nonnull Object owner,
final @Nonnull Lookup ... extraLookups)
{
return createLookup(Lookup.getDefault(), owner, extraLookups);
}
/*******************************************************************************************************************
*
* @param lookup the context {@code Lookup}
* @param owner the owner
* @param extraLookups the extra Lookup
s
* @return the Lookup
for the owner
*
******************************************************************************************************************/
@Nonnull
public static Lookup createLookup (final @Nonnull Lookup lookup,
final @Nonnull Object owner,
final @Nonnull Lookup ... extraLookups)
{
log.trace("createLookup({}, {}, ...)", lookup, owner);
final List lookups = new ArrayList();
for (final CapabilitiesProvider provider : lookup.lookupAll(CapabilitiesProvider.class))
{
if (provider.getManagedClass().isAssignableFrom(owner.getClass()))
{
lookups.add(Lookups.fixed(provider.createCapabilities(owner).toArray()));
lookups.addAll(provider.createLookups(owner));
}
}
for (final RoleProvider provider : lookup.lookupAll(RoleProvider.class))
{
if (provider.getOwnerClass().isAssignableFrom(owner.getClass()))
{
lookups.add(Lookups.fixed(provider.createRoles(owner).toArray()));
lookups.addAll(provider.createLookups(owner));
}
}
lookups.addAll(Arrays.asList(extraLookups));
log.trace(">>>> lookups for {}: {}", owner, Arrays.toString(extraLookups));
return new ProxyLookup(lookups.toArray(new Lookup[lookups.size()]));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy