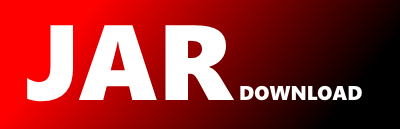
it.tidalwave.netbeans.loggerconfiguration.impl.LoggerConfigurationImpl Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* OpenBlueSky - NetBeans Platform Enhancements
* Copyright (C) 2006-2012 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://openbluesky.java.net
* SCM: https://bitbucket.org/tidalwave/openbluesky-src
*
**********************************************************************************************************************/
package it.tidalwave.netbeans.loggerconfiguration.impl;
import javax.annotation.Nonnull;
import java.util.Collection;
import java.util.Collections;
import java.util.Properties;
import java.util.logging.LogManager;
import java.util.logging.Logger;
import java.util.logging.Handler;
import java.util.prefs.Preferences;
import java.io.BufferedInputStream;
import java.io.ByteArrayInputStream;
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.IOException;
import edu.umd.cs.findbugs.annotations.SuppressWarnings;
import org.openide.util.NbPreferences;
import org.apache.commons.io.IOUtils;
import it.tidalwave.netbeans.loggerconfiguration.LoggerConfiguration;
/*******************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
******************************************************************************/
public class LoggerConfigurationImpl implements LoggerConfiguration
{
private static final String CLASS = LoggerConfigurationImpl.class.getName();
private static final String FILE_HANDLER_PATTERN = "java.util.logging.FileHandler.pattern";
private static final String DEBUG_PROPERTY = "it.tidalwave.netbeans.loggerconfiguration.debug";
private static final String DEBUG_CONFIG_FILE = "log-debug.properties";
private static final String PRODUCTION_CONFIG_FILE = "log.properties";
private static final String VERSION_PREFERENCE = "it.tidalwave.netbeans.loggerconfiguration.version";
private static final String VERSION = "v2";
private static final boolean DEBUG = Boolean.getBoolean(DEBUG_PROPERTY);
/***************************************************************************
*
*
**************************************************************************/
public LoggerConfigurationImpl()
{
}
/***************************************************************************
*
*
**************************************************************************/
@Override
public void initialize (final @Nonnull File logConfigFile,
final @Nonnull String logName,
final @Nonnull File logFolder)
{
initialize(logConfigFile, logName, logFolder, new Properties());
}
/***************************************************************************
*
*
**************************************************************************/
@Override @edu.umd.cs.findbugs.annotations.SuppressWarnings("REC_CATCH_EXCEPTION")
public void initialize (final @Nonnull File logConfigFile,
final @Nonnull String logName,
final @Nonnull File logFolder,
final @Nonnull Properties extraProperties)
{
try
{
System.err.println("INITIALIZING LOGGING (logConfigFile: " + logConfigFile.getAbsolutePath() + ")");
final Preferences preferences = NbPreferences.forModule(LoggerConfigurationImpl.class);
final String version = preferences.get(VERSION_PREFERENCE, "");
final boolean latestVersion = VERSION.equals(version);
if (!logConfigFile.exists() || !latestVersion)
{
generateConfigFile(logConfigFile, extraProperties);
}
preferences.put(VERSION_PREFERENCE, VERSION);
createDirectory(logFolder);
final byte[] buffer = expandMacros(logConfigFile, logName, logFolder);
LogManager.getLogManager().readConfiguration(new ByteArrayInputStream(buffer));
System.err.println(">>>> Initialized logger subsystem");
//
// The formatter must be set programmatically as the property in the log.properties won't
// be honored. I suspect it is related with NetBeans module classloaders as the formatter
// is loaded inside LogManager by using the SystemClassLoader, which only sees the classpath.
//
final SingleLineLogFormatter formatter = new SingleLineLogFormatter();
Logger rootLogger = Logger.getLogger(CLASS);
while (rootLogger.getParent() != null)
{
rootLogger = rootLogger.getParent();
}
for (final Handler handler : rootLogger.getHandlers())
{
handler.setFormatter(formatter);
}
}
catch (Exception e)
{
e.printStackTrace();
}
}
/***************************************************************************
*
* @param file the template configuration file
* @param logName the name of the log file
* @param logFolder the log folder
* @param extraProperties some extra properties to append to the config
*
**************************************************************************/
@Nonnull
@SuppressWarnings("OS_OPEN_STREAM_EXCEPTION_PATH")
private byte[] expandMacros (final @Nonnull File file,
final @Nonnull String logName,
final @Nonnull File logFolder)
throws FileNotFoundException, IOException
{
final Properties properties = new Properties();
final BufferedInputStream bis = new BufferedInputStream(new FileInputStream(file));
properties.load(bis);
bis.close();
String s = properties.getProperty(FILE_HANDLER_PATTERN);
s = s.replaceAll("\\$LOGFOLDER", logFolder.getAbsolutePath());
s = s.replaceAll("\\$LOGNAME", logName);
properties.setProperty(FILE_HANDLER_PATTERN, s);
final ByteArrayOutputStream os = new ByteArrayOutputStream();
properties.store(os, "");
os.close();
return os.toByteArray();
}
/***************************************************************************
*
*
**************************************************************************/
@SuppressWarnings({"OS_OPEN_STREAM_EXCEPTION_PATH", "UI_INHERITANCE_UNSAFE_GETRESOURCE"})
private void generateConfigFile (final @Nonnull File logConfigFile,
final @Nonnull Properties extraProperties)
throws FileNotFoundException, IOException
{
System.err.println(">>>> Generating " + logConfigFile);
final String configFileName = DEBUG ? DEBUG_CONFIG_FILE : PRODUCTION_CONFIG_FILE;
final Properties properties = new Properties();
InputStream is = null;
FileOutputStream fos = null;
try
{
is = getClass().getResourceAsStream(configFileName);
properties.load(is);
for (final String propertyName : (Collection)Collections.list(extraProperties.propertyNames()))
{
properties.setProperty(propertyName, extraProperties.getProperty(propertyName));
}
fos = new FileOutputStream(logConfigFile);
properties.store(fos, "");
}
finally
{
IOUtils.closeQuietly(is);
IOUtils.closeQuietly(fos);
}
}
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
private static void createDirectory (final @Nonnull File folder)
{
if (!folder.mkdirs() && !folder.exists())
{
throw new RuntimeException("Cannot create " + folder);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy