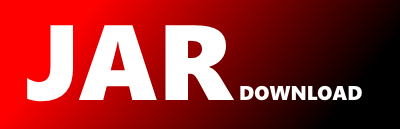
it.tidalwave.semantic.node.impl.RootEntityNode Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* blueMarine Semantic - open source media workflow
* Copyright (C) 2008-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://bluemarine.tidalwave.it
* SCM: https://kenai.com/hg/bluemarine~semantic-src
*
**********************************************************************************************************************/
package it.tidalwave.semantic.node.impl;
import javax.annotation.Nonnull;
import java.util.logging.Logger;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
import java.util.Locale;
import java.text.Collator;
import java.io.IOException;
import it.tidalwave.semantic.other.Entity;
import it.tidalwave.semantic.other.Type;
/*******************************************************************************
*
* A Node subclass for holding the Tags that are children of a given Category.
*
* @author Fabrizio Giudici
* @version $Id: RootEntityNode.java,v 4fbb06e9364c 2011/03/06 21:25:16 fabrizio $
*
******************************************************************************/
public class RootEntityNode extends EntityNode
{
private final static String CLASS = RootEntityNode.class.getName();
private final static Logger logger = Logger.getLogger(CLASS);
private final Type type;
/***************************************************************************
*
*
**************************************************************************/
private static class EntityChildFactory extends EntityNode.EntityChildFactory
{
@Nonnull
private final Type type;
public EntityChildFactory (@Nonnull final Type type)
{
super(null);
this.type = type;
}
@Override
protected boolean createKeys (final List keys)
{
final List entities = new ArrayList(type.getAllEntities());
final Collator collator = Collator.getInstance(Locale.getDefault());
Collections.sort(entities, new Comparator()
{
public int compare (final Entity e1, final Entity e2)
{
return collator.compare(e1.getDisplayName(), e2.getDisplayName());
}
});
keys.addAll(entities);
return true;
}
};
/***************************************************************************
*
* Listens to changes in tags and updates the node. This single listener is
* responsible for updating all the tree.
*
**************************************************************************/
// private final CatalogListener tagListener = new CatalogAdapter()
// {
// @Override
// public void tagAdded (final CatalogEvent event)
// {
// final Tag tag = event.getTag();
// TagNode node = tagChildFactory.getNodeFinder().findNode(tag.getParent());
// //
// // If we don't know yet the parent tag, see if it's a child of our category.
// //
// if ((node == null) && (tag.getCategory().equals(type)))
// {
// node = RootTagNode.this;
// }
//
// if (node != null)
// {
// node.refreshChildren();
// }
// }
//
// @Override
// public void tagRemoved (final CatalogEvent event)
// {
// final Tag tag = event.getTag();
// final TagNode node = tagChildFactory.getNodeFinder().findNode(tag);
//
// if (node != null)
// {
// try
// {
// node.destroy();
// }
// catch (IOException e)
// {
// logger.throwing(CLASS, "tagRemoved()", e);
// }
// }
// }
//
// @Override
// public void tagUpdated (final CatalogEvent event)
// {
// final Tag tag = event.getTag();
// final TagNode node = tagChildFactory.getNodeFinder().findNode(tag);
//
// if (node != null)
// {
// node.refreshAttributes();
// }
// }
// };
/***************************************************************************
*
*
**************************************************************************/
public RootEntityNode (@Nonnull final Type type)
{
super(new EntityChildFactory(type));
this.type = type;
setName("Entities in " + type.getDisplayName()); // init here, setAttributes() won't have category set
// catalog.addListener(tagListener);
}
/***************************************************************************
*
*
**************************************************************************/
@Override
public void destroy()
throws IOException
{
// catalog.removeListener(tagListener);
super.destroy();
}
@Override
public boolean canCopy()
{
return false;
}
@Override
public boolean canCut()
{
return false;
}
@Override
public boolean canDestroy()
{
return false;
}
@Override
public boolean canRename()
{
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy