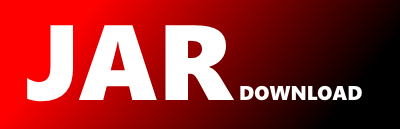
it.tidalwave.integritychecker.archive.impl.InMemoryScan Maven / Gradle / Ivy
/*
* #%L
* *********************************************************************************************************************
*
* SolidBlue - open source safe data
* http://solidblue.tidalwave.it - hg clone https://bitbucket.org/tidalwave/solidblue-src
* %%
* Copyright (C) 2011 - 2014 Tidalwave s.a.s. (http://tidalwave.it)
* %%
* *********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* *********************************************************************************************************************
*
* $Id$
*
* *********************************************************************************************************************
* #L%
*/
package it.tidalwave.integritychecker.archive.impl;
import javax.annotation.CheckForNull;
import javax.annotation.Nonnull;
import javax.annotation.concurrent.NotThreadSafe;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.TreeMap;
import java.nio.file.Path;
import org.joda.time.DateTime;
import it.tidalwave.util.spi.FinderSupport;
import it.tidalwave.integritychecker.archive.Scan;
import it.tidalwave.integritychecker.archive.ScanRecord;
import lombok.Getter;
/***********************************************************************************************************************
*
* An implementation of {@link Scan} based on an in-memory representation.
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
@NotThreadSafe
public class InMemoryScan implements Scan
{
/*******************************************************************************************************************
*
*
******************************************************************************************************************/
class InMemoryScanRecordFinder extends FinderSupport implements ScanRecordFinder
{
@CheckForNull
private Path path;
@Override @Nonnull
public InMemoryScanRecordFinder clone()
{
final InMemoryScanRecordFinder clone = (InMemoryScanRecordFinder)super.clone();
clone.path = this.path;
return clone;
}
@Override @Nonnull
public ScanRecordFinder withPath (final @Nonnull Path path)
{
final InMemoryScanRecordFinder clone = clone();
clone.path = path;
return clone;
}
@Override @Nonnull
protected List extends ScanRecord> computeNeededResults()
{
final List result = new ArrayList<>();
if (path != null)
{
final ScanRecord scanRecord = scanRecordMapByPath.get(path);
if (scanRecord != null)
{
result.add(scanRecord);
}
}
else
{
result.addAll(scanRecordMapByPath.values());
}
return result;
}
}
private final Map scanRecordMapByPath = new TreeMap<>();
@Getter @Nonnull
private final DateTime beginScanDateTime = new DateTime();
@Getter @Nonnull
private DateTime endScanDateTime = new DateTime();
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public ScanRecord.Builder addScanRecord()
{
return new ScanRecord.Builder(new ScanRecord.Builder.Callback()
{
@Override
public void register (final @Nonnull ScanRecord scanRecord)
{
scanRecordMapByPath.put(scanRecord.getFile().getFileName(), scanRecord);
endScanDateTime = new DateTime();
}
});
}
/*******************************************************************************************************************
*
* {@inheritDoc}
*
******************************************************************************************************************/
@Override @Nonnull
public ScanRecordFinder findScanRecord()
{
return new InMemoryScanRecordFinder();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy