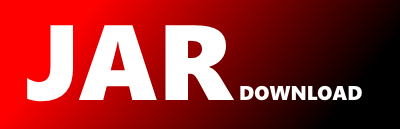
it.tidalwave.integritychecker.archive.spi.ThreadPoolBatchingStrategy Maven / Gradle / Ivy
/*
* #%L
* *********************************************************************************************************************
*
* SolidBlue - open source safe data
* http://solidblue.tidalwave.it - hg clone https://bitbucket.org/tidalwave/solidblue-src
* %%
* Copyright (C) 2011 - 2014 Tidalwave s.a.s. (http://tidalwave.it)
* %%
* *********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
* *********************************************************************************************************************
*
* $Id$
*
* *********************************************************************************************************************
* #L%
*/
package it.tidalwave.integritychecker.archive.spi;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.ThreadPoolExecutor;
import it.tidalwave.util.Task;
import lombok.Getter;
import lombok.extern.slf4j.Slf4j;
import static java.util.concurrent.TimeUnit.*;
/***********************************************************************************************************************
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
@Slf4j
public class ThreadPoolBatchingStrategy implements BatchingStrategy
{
@Getter @Nonnegative
private final int poolSize;
@Getter
private final String name;
public ThreadPoolBatchingStrategy (final @Nonnegative int poolSize)
{
this.poolSize = poolSize;
name = "" + poolSize + "thr";
}
@Override @Nonnull
public TaskBatch createBatch()
{
return new TaskBatch()
{
private final BlockingQueue queue = new ArrayBlockingQueue<>(10000000);
private final ExecutorService executor = new ThreadPoolExecutor(poolSize, poolSize, 1, SECONDS, queue);
@Override
public void add (final @Nonnull Task task)
{
executor.execute(new Runnable()
{
@Override
public void run()
{
try
{
task.run();
}
catch (Throwable t)
{
log.error("", t);
}
}
});
}
@Override
public void waitForCompletion()
{
try
{
executor.shutdown();
executor.awaitTermination(999, DAYS);
}
catch (InterruptedException e)
{
log.info("Interrupted", e);
}
}
};
}
@Override @Nonnull
public String toString()
{
return name;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy