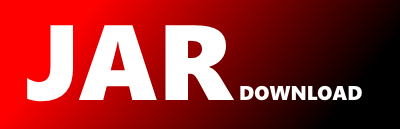
it.tidalwave.integritychecker.ui.impl.swing.IntegrityCheckerPresentationPanel Maven / Gradle / Ivy
The newest version!
/***********************************************************************************************************************
*
* SolidBlue - open source safe data
* Copyright (C) 2011-2012 by Tidalwave s.a.s. (http://tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://solidblue.tidalwave.it
* SCM: https://bitbucket.org/tidalwave/solidblue-src
*
**********************************************************************************************************************/
package it.tidalwave.integritychecker.ui.impl.swing;
import javax.annotation.Nonnull;
import java.awt.EventQueue;
import java.beans.Beans;
import javax.swing.JPanel;
import it.tidalwave.integritychecker.ui.IntegrityCheckerFieldsBean;
import it.tidalwave.integritychecker.ui.IntegrityCheckerPresentation;
import lombok.extern.slf4j.Slf4j;
/***********************************************************************************************************************
*
* @stereotype Presentation
*
* @author Fabrizio Giudici
* @version $Id$
*
**********************************************************************************************************************/
@Slf4j
public final class IntegrityCheckerPresentationPanel extends JPanel implements IntegrityCheckerPresentation
{
public IntegrityCheckerPresentationPanel()
{
initComponents();
if (!Beans.isDesignTime())
{
populateImmediately(new IntegrityCheckerFieldsBean());
}
}
@Override
public void populate (final @Nonnull IntegrityCheckerFieldsBean fields)
{
log.info("STATS: {}", fields);
EventQueue.invokeLater(new Runnable()
{
@Override
public void run()
{
populateImmediately(fields);
}
});
}
private void populateImmediately (final @Nonnull IntegrityCheckerFieldsBean fields)
{
lbDataAmountValue.setText(fields.getTotal());
lbDataProcessedValue.setText(fields.getProcessed());
lbElapsedTimeValue.setText(fields.getElapsedTime());
lbRemainingTimeValue.setText(fields.getRemainingTime());
lbSpeedValue.setText(fields.getSpeed());
pbProgressBar.setValue((int)Math.round(fields.getProgress() * pbProgressBar.getMaximum()));
pbProgressBar.setString(fields.getProgressLabel());
}
/*
* This method is called from within the constructor to initialize the form.
* WARNING: Do NOT modify this code. The content of this method is always regenerated by the Form Editor.
*/
@SuppressWarnings("unchecked")
// //GEN-BEGIN:initComponents
private void initComponents() {
lbElapsedTime = new javax.swing.JLabel();
lbRemainingTime = new javax.swing.JLabel();
lbDataAmount = new javax.swing.JLabel();
lbDataProcessed = new javax.swing.JLabel();
lbSpeed = new javax.swing.JLabel();
pbProgressBar = new javax.swing.JProgressBar();
lbElapsedTimeValue = new javax.swing.JLabel();
lbRemainingTimeValue = new javax.swing.JLabel();
lbDataAmountValue = new javax.swing.JLabel();
lbDataProcessedValue = new javax.swing.JLabel();
lbSpeedValue = new javax.swing.JLabel();
setName("Form"); // NOI18N
lbElapsedTime.setFont(lbElapsedTime.getFont().deriveFont(lbElapsedTime.getFont().getSize()-2f));
lbElapsedTime.setText(org.openide.util.NbBundle.getMessage(IntegrityCheckerPresentationPanel.class, "IntegrityCheckerPresentationPanel.lbElapsedTime.text")); // NOI18N
lbElapsedTime.setName("lbElapsedTime"); // NOI18N
lbRemainingTime.setFont(lbRemainingTime.getFont().deriveFont(lbRemainingTime.getFont().getSize()-2f));
lbRemainingTime.setText(org.openide.util.NbBundle.getMessage(IntegrityCheckerPresentationPanel.class, "IntegrityCheckerPresentationPanel.lbRemainingTime.text")); // NOI18N
lbRemainingTime.setName("lbRemainingTime"); // NOI18N
lbDataAmount.setFont(lbDataAmount.getFont().deriveFont(lbDataAmount.getFont().getSize()-2f));
lbDataAmount.setText(org.openide.util.NbBundle.getMessage(IntegrityCheckerPresentationPanel.class, "IntegrityCheckerPresentationPanel.lbDataAmount.text")); // NOI18N
lbDataAmount.setName("lbDataAmount"); // NOI18N
lbDataProcessed.setFont(lbDataProcessed.getFont().deriveFont(lbDataProcessed.getFont().getSize()-2f));
lbDataProcessed.setText(org.openide.util.NbBundle.getMessage(IntegrityCheckerPresentationPanel.class, "IntegrityCheckerPresentationPanel.lbDataProcessed.text")); // NOI18N
lbDataProcessed.setName("lbDataProcessed"); // NOI18N
lbSpeed.setFont(lbSpeed.getFont().deriveFont(lbSpeed.getFont().getSize()-2f));
lbSpeed.setText(org.openide.util.NbBundle.getMessage(IntegrityCheckerPresentationPanel.class, "IntegrityCheckerPresentationPanel.lbSpeed.text")); // NOI18N
lbSpeed.setName("lbSpeed"); // NOI18N
pbProgressBar.setMaximum(1000);
pbProgressBar.setName("pbProgressBar"); // NOI18N
pbProgressBar.setStringPainted(true);
lbElapsedTimeValue.setText(org.openide.util.NbBundle.getMessage(IntegrityCheckerPresentationPanel.class, "IntegrityCheckerPresentationPanel.lbElapsedTimeValue.text")); // NOI18N
lbElapsedTimeValue.setName("lbElapsedTimeValue"); // NOI18N
lbRemainingTimeValue.setText(org.openide.util.NbBundle.getMessage(IntegrityCheckerPresentationPanel.class, "IntegrityCheckerPresentationPanel.lbRemainingTimeValue.text")); // NOI18N
lbRemainingTimeValue.setName("lbRemainingTimeValue"); // NOI18N
lbDataAmountValue.setText(org.openide.util.NbBundle.getMessage(IntegrityCheckerPresentationPanel.class, "IntegrityCheckerPresentationPanel.lbDataAmountValue.text")); // NOI18N
lbDataAmountValue.setName("lbDataAmountValue"); // NOI18N
lbDataProcessedValue.setText(org.openide.util.NbBundle.getMessage(IntegrityCheckerPresentationPanel.class, "IntegrityCheckerPresentationPanel.lbDataProcessedValue.text")); // NOI18N
lbDataProcessedValue.setName("lbDataProcessedValue"); // NOI18N
lbDataProcessedValue.setSize(new java.awt.Dimension(150, 16));
lbSpeedValue.setText(org.openide.util.NbBundle.getMessage(IntegrityCheckerPresentationPanel.class, "IntegrityCheckerPresentationPanel.lbSpeedValue.text")); // NOI18N
lbSpeedValue.setMaximumSize(new java.awt.Dimension(150, 16));
lbSpeedValue.setMinimumSize(new java.awt.Dimension(150, 16));
lbSpeedValue.setName("lbSpeedValue"); // NOI18N
lbSpeedValue.setPreferredSize(new java.awt.Dimension(150, 16));
lbSpeedValue.setSize(new java.awt.Dimension(150, 16));
javax.swing.GroupLayout layout = new javax.swing.GroupLayout(this);
this.setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(36, 36, 36)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(pbProgressBar, javax.swing.GroupLayout.PREFERRED_SIZE, 406, javax.swing.GroupLayout.PREFERRED_SIZE)
.addGroup(layout.createSequentialGroup()
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.TRAILING)
.addComponent(lbSpeed)
.addComponent(lbDataProcessed)
.addComponent(lbDataAmount)
.addComponent(lbRemainingTime)
.addComponent(lbElapsedTime))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING, false)
.addComponent(lbElapsedTimeValue, javax.swing.GroupLayout.PREFERRED_SIZE, 256, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(lbRemainingTimeValue, javax.swing.GroupLayout.PREFERRED_SIZE, 256, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(lbDataAmountValue, javax.swing.GroupLayout.PREFERRED_SIZE, 256, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(lbDataProcessedValue, javax.swing.GroupLayout.PREFERRED_SIZE, 256, javax.swing.GroupLayout.PREFERRED_SIZE)
.addComponent(lbSpeedValue, javax.swing.GroupLayout.PREFERRED_SIZE, 256, javax.swing.GroupLayout.PREFERRED_SIZE))))
.addGap(40, 40, 40))
);
layout.linkSize(javax.swing.SwingConstants.HORIZONTAL, new java.awt.Component[] {lbDataAmountValue, lbDataProcessedValue, lbElapsedTimeValue, lbRemainingTimeValue, lbSpeedValue});
layout.setVerticalGroup(
layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(42, 42, 42)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(lbElapsedTime)
.addComponent(lbElapsedTimeValue))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(lbRemainingTime)
.addComponent(lbRemainingTimeValue))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(lbDataAmount)
.addComponent(lbDataAmountValue))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(lbDataProcessed)
.addComponent(lbDataProcessedValue))
.addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED)
.addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE)
.addComponent(lbSpeed)
.addComponent(lbSpeedValue, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))
.addGap(18, 18, 18)
.addComponent(pbProgressBar, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)
.addContainerGap(53, Short.MAX_VALUE))
);
layout.linkSize(javax.swing.SwingConstants.VERTICAL, new java.awt.Component[] {lbDataAmountValue, lbDataProcessedValue, lbElapsedTimeValue, lbRemainingTimeValue, lbSpeedValue});
}// //GEN-END:initComponents
// Variables declaration - do not modify//GEN-BEGIN:variables
private javax.swing.JLabel lbDataAmount;
private javax.swing.JLabel lbDataAmountValue;
private javax.swing.JLabel lbDataProcessed;
private javax.swing.JLabel lbDataProcessedValue;
private javax.swing.JLabel lbElapsedTime;
private javax.swing.JLabel lbElapsedTimeValue;
private javax.swing.JLabel lbRemainingTime;
private javax.swing.JLabel lbRemainingTimeValue;
private javax.swing.JLabel lbSpeed;
private javax.swing.JLabel lbSpeedValue;
private javax.swing.JProgressBar pbProgressBar;
// End of variables declaration//GEN-END:variables
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy