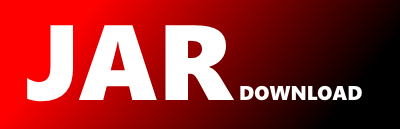
it.tidalwave.role.Composite Maven / Gradle / Ivy
/***********************************************************************************************************************
*
* These Foolish Things - Miscellaneous utilities
* Copyright (C) 2009-2011 by Tidalwave s.a.s. (http://www.tidalwave.it)
*
***********************************************************************************************************************
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*
***********************************************************************************************************************
*
* WWW: http://thesefoolishthings.java.net
* SCM: https://bitbucket.org/tidalwave/thesefoolishthings-src
*
**********************************************************************************************************************/
package it.tidalwave.role;
import javax.annotation.Nonnull;
import java.util.Collections;
import java.util.List;
import it.tidalwave.util.Finder;
import it.tidalwave.util.NotFoundException;
import it.tidalwave.util.spi.FinderSupport;
/***********************************************************************************************************************
*
* The role of a composite object, that is an object which contains children.
*
* @author Fabrizio Giudici
* @version $Id$
* @it.tidalwave.javadoc.stable
*
**********************************************************************************************************************/
public interface Composite>
{
//@bluebook-begin other
public static final Class Composite = Composite.class;
static class EmptyFinder extends FinderSupport
© 2015 - 2025 Weber Informatics LLC | Privacy Policy