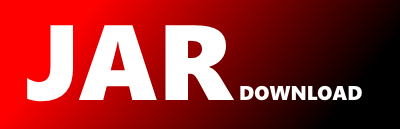
it.unibo.alchemist.model.geometry.Intersection2D.kt Maven / Gradle / Ivy
/*
* Copyright (C) 2010-2023, Danilo Pianini and contributors
* listed, for each module, in the respective subproject's build.gradle.kts file.
*
* This file is part of Alchemist, and is distributed under the terms of the
* GNU General Public License, with a linking exception,
* as described in the file LICENSE in the Alchemist distribution's top directory.
*/
package it.unibo.alchemist.model.geometry
/**
* Describes the result an intersection operation in an euclidean 2D space.
* Type [V] must extend [Vector2D].
* The requirement is not explicitly enforced to allow the class to work covariantly.
*/
sealed class Intersection2D {
/**
* List of intersection points (in case of infinite points this is empty).
*/
open val asList: List = emptyList()
/**
* Objects do not intersect.
*/
object None : Intersection2D()
/**
* Objects intersect in a single [point].
*/
data class SinglePoint>(
val point: P,
) : Intersection2D
() {
override val asList = listOf(point)
}
/**
* Objects intersect in a discrete number of [points].
*/
data class MultiplePoints
>(
val points: List
,
) : Intersection2D
() {
override val asList: List
get() = points
}
/**
* Objects intersect in infinite points (e.g. overlapping segments).
*/
object InfinitePoints : Intersection2D()
/**
* Factory methods for [Intersection2D].
*/
companion object {
/**
* @returns the correct intersection object depending of the number of items in the list.
*/
fun > create(points: List
): Intersection2D
=
when {
points.isEmpty() -> None
points.size == 1 -> SinglePoint(points.first())
else -> MultiplePoints(points)
}
}
}