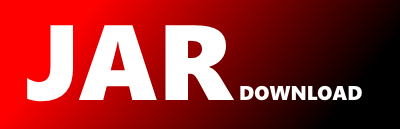
commonMain.it.unibo.collektive.stdlib.booleanarrays.FieldedArraysExtensions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stdlib-jvm Show documentation
Show all versions of stdlib-jvm Show documentation
DSL for Aggregate Computing in Kotlin
// This file is auto-generated by the Collektive code generator. Do not edit it manually.
@file:Suppress("all","ktlint")
package it.unibo.collektive.stdlib.booleanarrays
import it.unibo.collektive.`field`.Field
import it.unibo.collektive.`field`.Field.Companion.checkAligned
import kotlin.Any
import kotlin.Boolean
import kotlin.BooleanArray
import kotlin.CharSequence
import kotlin.Comparable
import kotlin.Comparator
import kotlin.Function1
import kotlin.Function2
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Collection
import kotlin.collections.IndexedValue
import kotlin.collections.Iterable
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.collections.Set
import kotlin.jvm.JvmName
import kotlin.random.Random
import kotlin.ranges.IntRange
public object FieldedArraysExtensions {
@JvmName("all_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_Boolean_end")
public inline fun Field.all(crossinline
predicate: Function1): Field =
this.mapWithId { _, receiver -> receiver.all(predicate) }
@JvmName("all_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun BooleanArray.all(predicate: Field>):
Field = predicate.mapWithId { id, receiver -> this.all(predicate[id]) }
@JvmName("all_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun
Field.all(predicate: Field>):
Field {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.all(predicate[id]) }
}
@JvmName("any_with_Field_of_BooleanArray_end")
public fun Field.any(): Field = map { it.any() }
@JvmName("any_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_Boolean_end")
public inline fun Field.any(crossinline
predicate: Function1): Field =
this.mapWithId { _, receiver -> receiver.any(predicate) }
@JvmName("any_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun BooleanArray.any(predicate: Field>):
Field = predicate.mapWithId { id, receiver -> this.any(predicate[id]) }
@JvmName("any_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun
Field.any(predicate: Field>):
Field {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.any(predicate[id]) }
}
@JvmName("asIterable_with_Field_of_BooleanArray_end")
public fun Field.asIterable(): Field> =
map { it.asIterable() }
@JvmName("asList_with_Field_of_BooleanArray_end")
public fun Field.asList(): Field> =
map { it.asList() }
@JvmName("associate_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_Pair_of_K_and_V_end_end")
public inline fun Field.associate(crossinline
transform: Function1>): Field> =
this.mapWithId { _, receiver -> receiver.associate(transform) }
@JvmName("associate_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_Pair_of_K_and_V_end_end_end")
public fun
BooleanArray.associate(transform: Field>>):
Field> = transform.mapWithId { id, receiver -> this.associate(transform[id]) }
@JvmName("associate_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_Pair_of_K_and_V_end_end_end")
public fun
Field.associate(transform: Field>>):
Field> {
checkAligned(this, transform)
return this.mapWithId { id, receiver -> receiver.associate(transform[id]) }
}
@JvmName("associateBy_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_K_end")
public inline fun Field.associateBy(crossinline
keySelector: Function1): Field> =
this.mapWithId { _, receiver -> receiver.associateBy(keySelector) }
@JvmName("associateBy_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_K_end_end")
public fun BooleanArray.associateBy(keySelector: Field>):
Field> =
keySelector.mapWithId { id, receiver -> this.associateBy(keySelector[id]) }
@JvmName("associateBy_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_K_end_end")
public fun
Field.associateBy(keySelector: Field>):
Field> {
checkAligned(this, keySelector)
return this.mapWithId { id, receiver -> receiver.associateBy(keySelector[id]) }
}
@JvmName("associateBy_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_K_end_and_Function1_of_Boolean_and_V_end")
public inline fun Field.associateBy(crossinline
keySelector: Function1, crossinline valueTransform: Function1):
Field> =
this.mapWithId { _, receiver -> receiver.associateBy(keySelector, valueTransform) }
@JvmName("associateBy_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_K_end_end_and_Function1_of_Boolean_and_V_end")
public inline fun
BooleanArray.associateBy(keySelector: Field>, crossinline
valueTransform: Function1): Field> =
keySelector.mapWithId { id, receiver -> this.associateBy(keySelector[id], valueTransform) }
@JvmName("associateBy_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_K_end_end_and_Function1_of_Boolean_and_V_end")
public inline fun
Field.associateBy(keySelector: Field>, crossinline
valueTransform: Function1): Field> {
checkAligned(this, keySelector)
return this.mapWithId { id, receiver -> receiver.associateBy(keySelector[id], valueTransform) }
}
@JvmName("associateBy_with_BooleanArray_and_Function1_of_Boolean_and_K_end_and_Field_of_Function1_of_Boolean_and_V_end_end")
public inline fun BooleanArray.associateBy(crossinline
keySelector: Function1, valueTransform: Field>):
Field> =
valueTransform.mapWithId { id, receiver -> this.associateBy(keySelector, valueTransform[id]) }
@JvmName("associateBy_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_K_end_and_Field_of_Function1_of_Boolean_and_V_end_end")
public inline fun Field.associateBy(crossinline
keySelector: Function1, valueTransform: Field>):
Field> {
checkAligned(this, valueTransform)
return this.mapWithId { id, receiver -> receiver.associateBy(keySelector, valueTransform[id]) }
}
@JvmName("associateBy_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_K_end_end_and_Field_of_Function1_of_Boolean_and_V_end_end")
public fun
BooleanArray.associateBy(keySelector: Field>,
valueTransform: Field>): Field> {
checkAligned(keySelector, valueTransform)
return keySelector.mapWithId { id, receiver -> this.associateBy(keySelector[id], valueTransform[id]) }
}
@JvmName("associateBy_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_K_end_end_and_Field_of_Function1_of_Boolean_and_V_end_end")
public fun
Field.associateBy(keySelector: Field>,
valueTransform: Field>): Field> {
checkAligned(this, keySelector, valueTransform)
return this.mapWithId { id, receiver -> receiver.associateBy(keySelector[id], valueTransform[id]) }
}
@JvmName("contains_with_Field_of_BooleanArray_end_and_Boolean")
public fun Field.contains(element: Boolean): Field =
this.mapWithId { _, receiver -> receiver.contains(element) }
@JvmName("contains_with_BooleanArray_and_Field_of_Boolean_end")
public fun BooleanArray.contains(element: Field): Field =
element.mapWithId { id, receiver -> this.contains(element[id]) }
@JvmName("contains_with_Field_of_BooleanArray_end_and_Field_of_Boolean_end")
public fun Field.contains(element: Field):
Field {
checkAligned(this, element)
return this.mapWithId { id, receiver -> receiver.contains(element[id]) }
}
@JvmName("count_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_Boolean_end")
public inline fun Field.count(crossinline
predicate: Function1): Field =
this.mapWithId { _, receiver -> receiver.count(predicate) }
@JvmName("count_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun BooleanArray.count(predicate: Field>):
Field = predicate.mapWithId { id, receiver -> this.count(predicate[id]) }
@JvmName("count_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun
Field.count(predicate: Field>):
Field {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.count(predicate[id]) }
}
@JvmName("distinct_with_Field_of_BooleanArray_end")
public fun Field.distinct(): Field> =
map { it.distinct() }
@JvmName("distinctBy_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_K_end")
public inline fun Field.distinctBy(crossinline
selector: Function1): Field> =
this.mapWithId { _, receiver -> receiver.distinctBy(selector) }
@JvmName("distinctBy_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_K_end_end")
public fun BooleanArray.distinctBy(selector: Field>):
Field> =
selector.mapWithId { id, receiver -> this.distinctBy(selector[id]) }
@JvmName("distinctBy_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_K_end_end")
public fun
Field.distinctBy(selector: Field>):
Field> {
checkAligned(this, selector)
return this.mapWithId { id, receiver -> receiver.distinctBy(selector[id]) }
}
@JvmName("drop_with_Field_of_BooleanArray_end_and_Int")
public fun Field.drop(n: Int): Field> =
this.mapWithId { _, receiver -> receiver.drop(n) }
@JvmName("drop_with_BooleanArray_and_Field_of_Int_end")
public fun BooleanArray.drop(n: Field): Field> =
n.mapWithId { id, receiver -> this.drop(n[id]) }
@JvmName("drop_with_Field_of_BooleanArray_end_and_Field_of_Int_end")
public fun Field.drop(n: Field): Field> {
checkAligned(this, n)
return this.mapWithId { id, receiver -> receiver.drop(n[id]) }
}
@JvmName("dropLast_with_Field_of_BooleanArray_end_and_Int")
public fun Field.dropLast(n: Int): Field> =
this.mapWithId { _, receiver -> receiver.dropLast(n) }
@JvmName("dropLast_with_BooleanArray_and_Field_of_Int_end")
public fun BooleanArray.dropLast(n: Field): Field> =
n.mapWithId { id, receiver -> this.dropLast(n[id]) }
@JvmName("dropLast_with_Field_of_BooleanArray_end_and_Field_of_Int_end")
public fun Field.dropLast(n: Field):
Field> {
checkAligned(this, n)
return this.mapWithId { id, receiver -> receiver.dropLast(n[id]) }
}
@JvmName("dropLastWhile_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_Boolean_end")
public inline fun Field.dropLastWhile(crossinline
predicate: Function1): Field> =
this.mapWithId { _, receiver -> receiver.dropLastWhile(predicate) }
@JvmName("dropLastWhile_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun
BooleanArray.dropLastWhile(predicate: Field>):
Field> =
predicate.mapWithId { id, receiver -> this.dropLastWhile(predicate[id]) }
@JvmName("dropLastWhile_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun
Field.dropLastWhile(predicate: Field>):
Field> {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.dropLastWhile(predicate[id]) }
}
@JvmName("dropWhile_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_Boolean_end")
public inline fun Field.dropWhile(crossinline
predicate: Function1): Field> =
this.mapWithId { _, receiver -> receiver.dropWhile(predicate) }
@JvmName("dropWhile_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun BooleanArray.dropWhile(predicate: Field>):
Field> =
predicate.mapWithId { id, receiver -> this.dropWhile(predicate[id]) }
@JvmName("dropWhile_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun
Field.dropWhile(predicate: Field>):
Field> {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.dropWhile(predicate[id]) }
}
@JvmName("filter_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_Boolean_end")
public inline fun Field.filter(crossinline
predicate: Function1): Field> =
this.mapWithId { _, receiver -> receiver.filter(predicate) }
@JvmName("filter_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun BooleanArray.filter(predicate: Field>):
Field> = predicate.mapWithId { id, receiver -> this.filter(predicate[id]) }
@JvmName("filter_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun
Field.filter(predicate: Field>):
Field> {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.filter(predicate[id]) }
}
@JvmName("filterIndexed_with_Field_of_BooleanArray_end_and_Function2_of_Int_and_Boolean_and_Boolean_end")
public inline fun Field.filterIndexed(crossinline
predicate: Function2): Field> =
this.mapWithId { _, receiver -> receiver.filterIndexed(predicate) }
@JvmName("filterIndexed_with_BooleanArray_and_Field_of_Function2_of_Int_and_Boolean_and_Boolean_end_end")
public fun
BooleanArray.filterIndexed(predicate: Field>):
Field> =
predicate.mapWithId { id, receiver -> this.filterIndexed(predicate[id]) }
@JvmName("filterIndexed_with_Field_of_BooleanArray_end_and_Field_of_Function2_of_Int_and_Boolean_and_Boolean_end_end")
public fun
Field.filterIndexed(predicate: Field>):
Field> {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.filterIndexed(predicate[id]) }
}
@JvmName("filterNot_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_Boolean_end")
public inline fun Field.filterNot(crossinline
predicate: Function1): Field> =
this.mapWithId { _, receiver -> receiver.filterNot(predicate) }
@JvmName("filterNot_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun BooleanArray.filterNot(predicate: Field>):
Field> =
predicate.mapWithId { id, receiver -> this.filterNot(predicate[id]) }
@JvmName("filterNot_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun
Field.filterNot(predicate: Field>):
Field> {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.filterNot(predicate[id]) }
}
@JvmName("first_with_Field_of_BooleanArray_end")
public fun Field.first(): Field = map { it.first() }
@JvmName("first_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_Boolean_end")
public inline fun Field.first(crossinline
predicate: Function1): Field =
this.mapWithId { _, receiver -> receiver.first(predicate) }
@JvmName("first_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun BooleanArray.first(predicate: Field>):
Field = predicate.mapWithId { id, receiver -> this.first(predicate[id]) }
@JvmName("first_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun
Field.first(predicate: Field>):
Field {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.first(predicate[id]) }
}
@JvmName("firstOrNull_with_Field_of_BooleanArray_end")
public fun Field.firstOrNull(): Field =
map { it.firstOrNull() }
@JvmName("firstOrNull_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_Boolean_end")
public inline fun Field.firstOrNull(crossinline
predicate: Function1): Field =
this.mapWithId { _, receiver -> receiver.firstOrNull(predicate) }
@JvmName("firstOrNull_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun BooleanArray.firstOrNull(predicate: Field>):
Field = predicate.mapWithId { id, receiver -> this.firstOrNull(predicate[id]) }
@JvmName("firstOrNull_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_Boolean_end_end")
public fun
Field.firstOrNull(predicate: Field>):
Field {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.firstOrNull(predicate[id]) }
}
@JvmName("flatMap_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_collections_Iterable_of_R_end_end")
public inline fun Field.flatMap(crossinline
transform: Function1>): Field> =
this.mapWithId { _, receiver -> receiver.flatMap(transform) }
@JvmName("flatMap_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_collections_Iterable_of_R_end_end_end")
public fun
BooleanArray.flatMap(transform: Field>>):
Field> = transform.mapWithId { id, receiver -> this.flatMap(transform[id]) }
@JvmName("flatMap_with_Field_of_BooleanArray_end_and_Field_of_Function1_of_Boolean_and_collections_Iterable_of_R_end_end_end")
public fun
Field.flatMap(transform: Field>>):
Field> {
checkAligned(this, transform)
return this.mapWithId { id, receiver -> receiver.flatMap(transform[id]) }
}
@JvmName("getOrNull_with_Field_of_BooleanArray_end_and_Int")
public fun Field.getOrNull(index: Int): Field =
this.mapWithId { _, receiver -> receiver.getOrNull(index) }
@JvmName("getOrNull_with_BooleanArray_and_Field_of_Int_end")
public fun BooleanArray.getOrNull(index: Field): Field =
index.mapWithId { id, receiver -> this.getOrNull(index[id]) }
@JvmName("getOrNull_with_Field_of_BooleanArray_end_and_Field_of_Int_end")
public fun Field.getOrNull(index: Field):
Field {
checkAligned(this, index)
return this.mapWithId { id, receiver -> receiver.getOrNull(index[id]) }
}
@JvmName("groupBy_with_Field_of_BooleanArray_end_and_Function1_of_Boolean_and_K_end")
public inline fun Field.groupBy(crossinline
keySelector: Function1): Field>> =
this.mapWithId { _, receiver -> receiver.groupBy(keySelector) }
@JvmName("groupBy_with_BooleanArray_and_Field_of_Function1_of_Boolean_and_K_end_end")
public fun BooleanArray.groupBy(keySelector: Field>):
Field