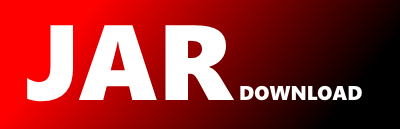
commonMain.it.unibo.collektive.stdlib.iterables.FieldedCollectionsExtensions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stdlib-jvm Show documentation
Show all versions of stdlib-jvm Show documentation
DSL for Aggregate Computing in Kotlin
// This file is auto-generated by the Collektive code generator. Do not edit it manually.
@file:Suppress("all","ktlint")
package it.unibo.collektive.stdlib.iterables
import it.unibo.collektive.`field`.Field
import it.unibo.collektive.`field`.Field.Companion.checkAligned
import kotlin.Any
import kotlin.Boolean
import kotlin.Byte
import kotlin.CharSequence
import kotlin.Comparable
import kotlin.Comparator
import kotlin.Double
import kotlin.Float
import kotlin.Function1
import kotlin.Function2
import kotlin.Function3
import kotlin.Int
import kotlin.Long
import kotlin.Pair
import kotlin.Short
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Grouping
import kotlin.collections.IndexedValue
import kotlin.collections.Iterable
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.collections.Set
import kotlin.jvm.JvmName
import kotlin.random.Random
import kotlin.sequences.Sequence
public object FieldedCollectionsExtensions {
@JvmName("all_with_Field_of_collections_Iterable_of_T_end_end_and_Function1_of_T_and_Boolean_end")
public inline fun Field>.all(crossinline
predicate: Function1): Field =
this.mapWithId { _, receiver -> receiver.all(predicate) }
@JvmName("all_with_collections_Iterable_of_T_end_and_Field_of_Function1_of_T_and_Boolean_end_end")
public fun Iterable.all(predicate: Field>):
Field = predicate.mapWithId { id, receiver -> this.all(predicate[id]) }
@JvmName("all_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Function1_of_T_and_Boolean_end_end")
public fun Field>.all(predicate: Field>):
Field {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.all(predicate[id]) }
}
@JvmName("any_with_Field_of_collections_Iterable_of_T_end_end")
public fun Field>.any(): Field = map { it.any() }
@JvmName("any_with_Field_of_collections_Iterable_of_T_end_end_and_Function1_of_T_and_Boolean_end")
public inline fun Field>.any(crossinline
predicate: Function1): Field =
this.mapWithId { _, receiver -> receiver.any(predicate) }
@JvmName("any_with_collections_Iterable_of_T_end_and_Field_of_Function1_of_T_and_Boolean_end_end")
public fun Iterable.any(predicate: Field>):
Field = predicate.mapWithId { id, receiver -> this.any(predicate[id]) }
@JvmName("any_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Function1_of_T_and_Boolean_end_end")
public fun Field>.any(predicate: Field>):
Field {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.any(predicate[id]) }
}
@JvmName("associate_with_Field_of_collections_Iterable_of_T_end_end_and_Function1_of_T_and_Pair_of_K_and_V_end_end")
public inline fun Field>.associate(crossinline
transform: Function1>): Field> =
this.mapWithId { _, receiver -> receiver.associate(transform) }
@JvmName("associate_with_collections_Iterable_of_T_end_and_Field_of_Function1_of_T_and_Pair_of_K_and_V_end_end_end")
public fun
Iterable.associate(transform: Field>>): Field> =
transform.mapWithId { id, receiver -> this.associate(transform[id]) }
@JvmName("associate_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Function1_of_T_and_Pair_of_K_and_V_end_end_end")
public fun
Field>.associate(transform: Field>>):
Field> {
checkAligned(this, transform)
return this.mapWithId { id, receiver -> receiver.associate(transform[id]) }
}
@JvmName("associateBy_with_Field_of_collections_Iterable_of_T_end_end_and_Function1_of_T_and_K_end")
public inline fun Field>.associateBy(crossinline
keySelector: Function1): Field> =
this.mapWithId { _, receiver -> receiver.associateBy(keySelector) }
@JvmName("associateBy_with_collections_Iterable_of_T_end_and_Field_of_Function1_of_T_and_K_end_end")
public fun Iterable.associateBy(keySelector: Field>):
Field> =
keySelector.mapWithId { id, receiver -> this.associateBy(keySelector[id]) }
@JvmName("associateBy_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Function1_of_T_and_K_end_end")
public fun
Field>.associateBy(keySelector: Field>):
Field> {
checkAligned(this, keySelector)
return this.mapWithId { id, receiver -> receiver.associateBy(keySelector[id]) }
}
@JvmName("associateBy_with_Field_of_collections_Iterable_of_T_end_end_and_Function1_of_T_and_K_end_and_Function1_of_T_and_V_end")
public inline fun Field>.associateBy(crossinline
keySelector: Function1, crossinline valueTransform: Function1):
Field> =
this.mapWithId { _, receiver -> receiver.associateBy(keySelector, valueTransform) }
@JvmName("associateBy_with_collections_Iterable_of_T_end_and_Field_of_Function1_of_T_and_K_end_end_and_Function1_of_T_and_V_end")
public inline fun
Iterable.associateBy(keySelector: Field>, crossinline
valueTransform: Function1): Field> =
keySelector.mapWithId { id, receiver -> this.associateBy(keySelector[id], valueTransform) }
@JvmName("associateBy_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Function1_of_T_and_K_end_end_and_Function1_of_T_and_V_end")
public inline fun
Field>.associateBy(keySelector: Field>, crossinline
valueTransform: Function1): Field> {
checkAligned(this, keySelector)
return this.mapWithId { id, receiver -> receiver.associateBy(keySelector[id], valueTransform) }
}
@JvmName("associateBy_with_collections_Iterable_of_T_end_and_Function1_of_T_and_K_end_and_Field_of_Function1_of_T_and_V_end_end")
public inline fun Iterable.associateBy(crossinline
keySelector: Function1, valueTransform: Field>):
Field> =
valueTransform.mapWithId { id, receiver -> this.associateBy(keySelector, valueTransform[id]) }
@JvmName("associateBy_with_Field_of_collections_Iterable_of_T_end_end_and_Function1_of_T_and_K_end_and_Field_of_Function1_of_T_and_V_end_end")
public inline fun Field>.associateBy(crossinline
keySelector: Function1, valueTransform: Field>):
Field> {
checkAligned(this, valueTransform)
return this.mapWithId { id, receiver -> receiver.associateBy(keySelector, valueTransform[id]) }
}
@JvmName("associateBy_with_collections_Iterable_of_T_end_and_Field_of_Function1_of_T_and_K_end_end_and_Field_of_Function1_of_T_and_V_end_end")
public fun Iterable.associateBy(keySelector: Field>,
valueTransform: Field>): Field> {
checkAligned(keySelector, valueTransform)
return keySelector.mapWithId { id, receiver -> this.associateBy(keySelector[id], valueTransform[id]) }
}
@JvmName("associateBy_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Function1_of_T_and_K_end_end_and_Field_of_Function1_of_T_and_V_end_end")
public fun
Field>.associateBy(keySelector: Field>,
valueTransform: Field>): Field> {
checkAligned(this, keySelector, valueTransform)
return this.mapWithId { id, receiver -> receiver.associateBy(keySelector[id], valueTransform[id]) }
}
@JvmName("associateWith_with_Field_of_collections_Iterable_of_K_end_end_and_Function1_of_K_and_V_end")
public inline fun Field>.associateWith(crossinline
valueSelector: Function1): Field> =
this.mapWithId { _, receiver -> receiver.associateWith(valueSelector) }
@JvmName("associateWith_with_collections_Iterable_of_K_end_and_Field_of_Function1_of_K_and_V_end_end")
public fun Iterable.associateWith(valueSelector: Field>):
Field> =
valueSelector.mapWithId { id, receiver -> this.associateWith(valueSelector[id]) }
@JvmName("associateWith_with_Field_of_collections_Iterable_of_K_end_end_and_Field_of_Function1_of_K_and_V_end_end")
public fun
Field>.associateWith(valueSelector: Field>):
Field> {
checkAligned(this, valueSelector)
return this.mapWithId { id, receiver -> receiver.associateWith(valueSelector[id]) }
}
@JvmName("average_with_Field_of_collections_Iterable_of_Byte_end_end")
public fun Field>.average(): Field =
map { it.average() }
@JvmName("average_with_Field_of_collections_Iterable_of_Short_end_end")
public fun Field>.average(): Field =
map { it.average() }
@JvmName("average_with_Field_of_collections_Iterable_of_Int_end_end")
public fun Field>.average(): Field = map { it.average() }
@JvmName("average_with_Field_of_collections_Iterable_of_Long_end_end")
public fun Field>.average(): Field =
map { it.average() }
@JvmName("average_with_Field_of_collections_Iterable_of_Float_end_end")
public fun Field>.average(): Field =
map { it.average() }
@JvmName("average_with_Field_of_collections_Iterable_of_Double_end_end")
public fun Field>.average(): Field =
map { it.average() }
@JvmName("chunked_with_Field_of_collections_Iterable_of_T_end_end_and_Int")
public fun Field>.chunked(size: Int): Field>> =
this.mapWithId { _, receiver -> receiver.chunked(size) }
@JvmName("chunked_with_collections_Iterable_of_T_end_and_Field_of_Int_end")
public fun Iterable.chunked(size: Field): Field>> =
size.mapWithId { id, receiver -> this.chunked(size[id]) }
@JvmName("chunked_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Int_end")
public fun Field>.chunked(size: Field):
Field>> {
checkAligned(this, size)
return this.mapWithId { id, receiver -> receiver.chunked(size[id]) }
}
@JvmName("chunked_with_Field_of_collections_Iterable_of_T_end_end_and_Int_and_Function1_of_collections_List_of_T_end_and_R_end")
public fun Field>.chunked(size: Int,
transform: Function1, R>): Field> =
this.mapWithId { _, receiver -> receiver.chunked(size, transform) }
@JvmName("chunked_with_collections_Iterable_of_T_end_and_Field_of_Int_end_and_Function1_of_collections_List_of_T_end_and_R_end")
public fun Iterable.chunked(size: Field,
transform: Function1, R>): Field> =
size.mapWithId { id, receiver -> this.chunked(size[id], transform) }
@JvmName("chunked_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Int_end_and_Function1_of_collections_List_of_T_end_and_R_end")
public fun Field>.chunked(size: Field,
transform: Function1, R>): Field> {
checkAligned(this, size)
return this.mapWithId { id, receiver -> receiver.chunked(size[id], transform) }
}
@JvmName("chunked_with_collections_Iterable_of_T_end_and_Int_and_Field_of_Function1_of_collections_List_of_T_end_and_R_end_end")
public fun Iterable.chunked(size: Int,
transform: Field, R>>): Field> =
transform.mapWithId { id, receiver -> this.chunked(size, transform[id]) }
@JvmName("chunked_with_Field_of_collections_Iterable_of_T_end_end_and_Int_and_Field_of_Function1_of_collections_List_of_T_end_and_R_end_end")
public fun Field>.chunked(size: Int,
transform: Field, R>>): Field> {
checkAligned(this, transform)
return this.mapWithId { id, receiver -> receiver.chunked(size, transform[id]) }
}
@JvmName("chunked_with_collections_Iterable_of_T_end_and_Field_of_Int_end_and_Field_of_Function1_of_collections_List_of_T_end_and_R_end_end")
public fun Iterable.chunked(size: Field,
transform: Field, R>>): Field> {
checkAligned(size, transform)
return size.mapWithId { id, receiver -> this.chunked(size[id], transform[id]) }
}
@JvmName("chunked_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Int_end_and_Field_of_Function1_of_collections_List_of_T_end_and_R_end_end")
public fun Field>.chunked(size: Field,
transform: Field, R>>): Field> {
checkAligned(this, size, transform)
return this.mapWithId { id, receiver -> receiver.chunked(size[id], transform[id]) }
}
@JvmName("contains_with_Field_of_collections_Iterable_of_T_end_end_and_T")
public fun Field>.contains(element: T): Field =
this.mapWithId { _, receiver -> receiver.contains(element) }
@JvmName("contains_with_collections_Iterable_of_T_end_and_Field_of_T_end")
public fun Iterable.contains(element: Field): Field =
element.mapWithId { id, receiver -> this.contains(element[id]) }
@JvmName("contains_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_T_end")
public fun Field>.contains(element: Field):
Field {
checkAligned(this, element)
return this.mapWithId { id, receiver -> receiver.contains(element[id]) }
}
@JvmName("count_with_Field_of_collections_Iterable_of_T_end_end")
public fun Field>.count(): Field = map { it.count() }
@JvmName("count_with_Field_of_collections_Iterable_of_T_end_end_and_Function1_of_T_and_Boolean_end")
public inline fun Field>.count(crossinline
predicate: Function1): Field =
this.mapWithId { _, receiver -> receiver.count(predicate) }
@JvmName("count_with_collections_Iterable_of_T_end_and_Field_of_Function1_of_T_and_Boolean_end_end")
public fun Iterable.count(predicate: Field>):
Field = predicate.mapWithId { id, receiver -> this.count(predicate[id]) }
@JvmName("count_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Function1_of_T_and_Boolean_end_end")
public fun
Field>.count(predicate: Field>): Field {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.count(predicate[id]) }
}
@JvmName("distinct_with_Field_of_collections_Iterable_of_T_end_end")
public fun Field>.distinct(): Field> =
map { it.distinct() }
@JvmName("distinctBy_with_Field_of_collections_Iterable_of_T_end_end_and_Function1_of_T_and_K_end")
public inline fun Field>.distinctBy(crossinline
selector: Function1): Field> =
this.mapWithId { _, receiver -> receiver.distinctBy(selector) }
@JvmName("distinctBy_with_collections_Iterable_of_T_end_and_Field_of_Function1_of_T_and_K_end_end")
public fun Iterable.distinctBy(selector: Field>):
Field> = selector.mapWithId { id, receiver -> this.distinctBy(selector[id]) }
@JvmName("distinctBy_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Function1_of_T_and_K_end_end")
public fun
Field>.distinctBy(selector: Field>): Field> {
checkAligned(this, selector)
return this.mapWithId { id, receiver -> receiver.distinctBy(selector[id]) }
}
@JvmName("drop_with_Field_of_collections_Iterable_of_T_end_end_and_Int")
public fun Field>.drop(n: Int): Field> =
this.mapWithId { _, receiver -> receiver.drop(n) }
@JvmName("drop_with_collections_Iterable_of_T_end_and_Field_of_Int_end")
public fun Iterable.drop(n: Field): Field> =
n.mapWithId { id, receiver -> this.drop(n[id]) }
@JvmName("drop_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Int_end")
public fun Field>.drop(n: Field): Field> {
checkAligned(this, n)
return this.mapWithId { id, receiver -> receiver.drop(n[id]) }
}
@JvmName("dropWhile_with_Field_of_collections_Iterable_of_T_end_end_and_Function1_of_T_and_Boolean_end")
public inline fun Field>.dropWhile(crossinline
predicate: Function1): Field> =
this.mapWithId { _, receiver -> receiver.dropWhile(predicate) }
@JvmName("dropWhile_with_collections_Iterable_of_T_end_and_Field_of_Function1_of_T_and_Boolean_end_end")
public fun Iterable.dropWhile(predicate: Field>):
Field> = predicate.mapWithId { id, receiver -> this.dropWhile(predicate[id]) }
@JvmName("dropWhile_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Function1_of_T_and_Boolean_end_end")
public fun
Field>.dropWhile(predicate: Field>):
Field> {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.dropWhile(predicate[id]) }
}
@JvmName("elementAt_with_Field_of_collections_Iterable_of_T_end_end_and_Int")
public fun Field>.elementAt(index: Int): Field =
this.mapWithId { _, receiver -> receiver.elementAt(index) }
@JvmName("elementAt_with_collections_Iterable_of_T_end_and_Field_of_Int_end")
public fun Iterable.elementAt(index: Field): Field =
index.mapWithId { id, receiver -> this.elementAt(index[id]) }
@JvmName("elementAt_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Int_end")
public fun Field>.elementAt(index: Field): Field {
checkAligned(this, index)
return this.mapWithId { id, receiver -> receiver.elementAt(index[id]) }
}
@JvmName("elementAtOrElse_with_Field_of_collections_Iterable_of_T_end_end_and_Int_and_Function1_of_Int_and_T_end")
public fun Field>.elementAtOrElse(index: Int,
defaultValue: Function1): Field =
this.mapWithId { _, receiver -> receiver.elementAtOrElse(index, defaultValue) }
@JvmName("elementAtOrElse_with_collections_Iterable_of_T_end_and_Field_of_Int_end_and_Function1_of_Int_and_T_end")
public fun Iterable.elementAtOrElse(index: Field,
defaultValue: Function1): Field =
index.mapWithId { id, receiver -> this.elementAtOrElse(index[id], defaultValue) }
@JvmName("elementAtOrElse_with_Field_of_collections_Iterable_of_T_end_end_and_Field_of_Int_end_and_Function1_of_Int_and_T_end")
public fun Field>.elementAtOrElse(index: Field,
defaultValue: Function1): Field