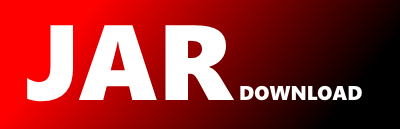
commonMain.it.unibo.collektive.stdlib.longs.FieldedLongs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stdlib-jvm Show documentation
Show all versions of stdlib-jvm Show documentation
DSL for Aggregate Computing in Kotlin
// This file is auto-generated by the Collektive code generator. Do not edit it manually.
@file:Suppress("all","ktlint")
package it.unibo.collektive.stdlib.longs
import it.unibo.collektive.`field`.Field
import it.unibo.collektive.`field`.Field.Companion.checkAligned
import kotlin.Any
import kotlin.Byte
import kotlin.Double
import kotlin.Float
import kotlin.Int
import kotlin.Long
import kotlin.Short
import kotlin.Suppress
import kotlin.jvm.JvmName
import kotlin.ranges.LongRange
public object FieldedLongs {
@JvmName("and_with_Field_of_Long_end_and_Long")
public infix fun Field.and(other: Long): Field =
this.mapWithId { _, receiver -> receiver.and(other) }
@JvmName("and_with_Long_and_Field_of_Long_end")
public infix fun Long.and(other: Field): Field =
other.mapWithId { id, receiver -> this.and(other[id]) }
@JvmName("and_with_Field_of_Long_end_and_Field_of_Long_end")
public infix fun Field.and(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.and(other[id]) }
}
@JvmName("compareTo_with_Field_of_Long_end_and_Byte")
public fun Field.compareTo(other: Byte): Field =
this.mapWithId { _, receiver -> receiver.compareTo(other) }
@JvmName("compareTo_with_Long_and_Field_of_Byte_end")
public fun Long.compareTo(other: Field): Field =
other.mapWithId { id, receiver -> this.compareTo(other[id]) }
@JvmName("compareTo_with_Field_of_Long_end_and_Field_of_Byte_end")
public fun Field.compareTo(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.compareTo(other[id]) }
}
@JvmName("compareTo_with_Field_of_Long_end_and_Double")
public fun Field.compareTo(other: Double): Field =
this.mapWithId { _, receiver -> receiver.compareTo(other) }
@JvmName("compareTo_with_Long_and_Field_of_Double_end")
public fun Long.compareTo(other: Field): Field =
other.mapWithId { id, receiver -> this.compareTo(other[id]) }
@JvmName("compareTo_with_Field_of_Long_end_and_Field_of_Double_end")
public fun Field.compareTo(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.compareTo(other[id]) }
}
@JvmName("compareTo_with_Field_of_Long_end_and_Float")
public fun Field.compareTo(other: Float): Field =
this.mapWithId { _, receiver -> receiver.compareTo(other) }
@JvmName("compareTo_with_Long_and_Field_of_Float_end")
public fun Long.compareTo(other: Field): Field =
other.mapWithId { id, receiver -> this.compareTo(other[id]) }
@JvmName("compareTo_with_Field_of_Long_end_and_Field_of_Float_end")
public fun Field.compareTo(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.compareTo(other[id]) }
}
@JvmName("compareTo_with_Field_of_Long_end_and_Int")
public fun Field.compareTo(other: Int): Field =
this.mapWithId { _, receiver -> receiver.compareTo(other) }
@JvmName("compareTo_with_Long_and_Field_of_Int_end")
public fun Long.compareTo(other: Field): Field =
other.mapWithId { id, receiver -> this.compareTo(other[id]) }
@JvmName("compareTo_with_Field_of_Long_end_and_Field_of_Int_end")
public fun Field.compareTo(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.compareTo(other[id]) }
}
@JvmName("compareTo_with_Field_of_Long_end_and_Long")
public fun Field.compareTo(other: Long): Field =
this.mapWithId { _, receiver -> receiver.compareTo(other) }
@JvmName("compareTo_with_Long_and_Field_of_Long_end")
public fun Long.compareTo(other: Field): Field =
other.mapWithId { id, receiver -> this.compareTo(other[id]) }
@JvmName("compareTo_with_Field_of_Long_end_and_Field_of_Long_end")
public fun Field.compareTo(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.compareTo(other[id]) }
}
@JvmName("compareTo_with_Field_of_Long_end_and_Short")
public fun Field.compareTo(other: Short): Field =
this.mapWithId { _, receiver -> receiver.compareTo(other) }
@JvmName("compareTo_with_Long_and_Field_of_Short_end")
public fun Long.compareTo(other: Field): Field =
other.mapWithId { id, receiver -> this.compareTo(other[id]) }
@JvmName("compareTo_with_Field_of_Long_end_and_Field_of_Short_end")
public fun Field.compareTo(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.compareTo(other[id]) }
}
@JvmName("div_with_Field_of_Long_end_and_Byte")
public operator fun Field.div(other: Byte): Field =
this.mapWithId { _, receiver -> receiver.div(other) }
@JvmName("div_with_Long_and_Field_of_Byte_end")
public operator fun Long.div(other: Field): Field =
other.mapWithId { id, receiver -> this.div(other[id]) }
@JvmName("div_with_Field_of_Long_end_and_Field_of_Byte_end")
public operator fun Field.div(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.div(other[id]) }
}
@JvmName("div_with_Field_of_Long_end_and_Double")
public operator fun Field.div(other: Double): Field =
this.mapWithId { _, receiver -> receiver.div(other) }
@JvmName("div_with_Long_and_Field_of_Double_end")
public operator fun Long.div(other: Field): Field =
other.mapWithId { id, receiver -> this.div(other[id]) }
@JvmName("div_with_Field_of_Long_end_and_Field_of_Double_end")
public operator fun Field.div(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.div(other[id]) }
}
@JvmName("div_with_Field_of_Long_end_and_Float")
public operator fun Field.div(other: Float): Field =
this.mapWithId { _, receiver -> receiver.div(other) }
@JvmName("div_with_Long_and_Field_of_Float_end")
public operator fun Long.div(other: Field): Field =
other.mapWithId { id, receiver -> this.div(other[id]) }
@JvmName("div_with_Field_of_Long_end_and_Field_of_Float_end")
public operator fun Field.div(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.div(other[id]) }
}
@JvmName("div_with_Field_of_Long_end_and_Int")
public operator fun Field.div(other: Int): Field =
this.mapWithId { _, receiver -> receiver.div(other) }
@JvmName("div_with_Long_and_Field_of_Int_end")
public operator fun Long.div(other: Field): Field =
other.mapWithId { id, receiver -> this.div(other[id]) }
@JvmName("div_with_Field_of_Long_end_and_Field_of_Int_end")
public operator fun Field.div(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.div(other[id]) }
}
@JvmName("div_with_Field_of_Long_end_and_Long")
public operator fun Field.div(other: Long): Field =
this.mapWithId { _, receiver -> receiver.div(other) }
@JvmName("div_with_Long_and_Field_of_Long_end")
public operator fun Long.div(other: Field): Field =
other.mapWithId { id, receiver -> this.div(other[id]) }
@JvmName("div_with_Field_of_Long_end_and_Field_of_Long_end")
public operator fun Field.div(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.div(other[id]) }
}
@JvmName("div_with_Field_of_Long_end_and_Short")
public operator fun Field.div(other: Short): Field =
this.mapWithId { _, receiver -> receiver.div(other) }
@JvmName("div_with_Long_and_Field_of_Short_end")
public operator fun Long.div(other: Field): Field =
other.mapWithId { id, receiver -> this.div(other[id]) }
@JvmName("div_with_Field_of_Long_end_and_Field_of_Short_end")
public operator fun Field.div(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.div(other[id]) }
}
@JvmName("inv_with_Field_of_Long_end")
public fun Field.inv(): Field = map { it.inv() }
@JvmName("minus_with_Field_of_Long_end_and_Byte")
public operator fun Field.minus(other: Byte): Field =
this.mapWithId { _, receiver -> receiver.minus(other) }
@JvmName("minus_with_Long_and_Field_of_Byte_end")
public operator fun Long.minus(other: Field): Field =
other.mapWithId { id, receiver -> this.minus(other[id]) }
@JvmName("minus_with_Field_of_Long_end_and_Field_of_Byte_end")
public operator fun Field.minus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.minus(other[id]) }
}
@JvmName("minus_with_Field_of_Long_end_and_Double")
public operator fun Field.minus(other: Double): Field =
this.mapWithId { _, receiver -> receiver.minus(other) }
@JvmName("minus_with_Long_and_Field_of_Double_end")
public operator fun Long.minus(other: Field): Field =
other.mapWithId { id, receiver -> this.minus(other[id]) }
@JvmName("minus_with_Field_of_Long_end_and_Field_of_Double_end")
public operator fun Field.minus(other: Field):
Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.minus(other[id]) }
}
@JvmName("minus_with_Field_of_Long_end_and_Float")
public operator fun Field.minus(other: Float): Field =
this.mapWithId { _, receiver -> receiver.minus(other) }
@JvmName("minus_with_Long_and_Field_of_Float_end")
public operator fun Long.minus(other: Field): Field =
other.mapWithId { id, receiver -> this.minus(other[id]) }
@JvmName("minus_with_Field_of_Long_end_and_Field_of_Float_end")
public operator fun Field.minus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.minus(other[id]) }
}
@JvmName("minus_with_Field_of_Long_end_and_Int")
public operator fun Field.minus(other: Int): Field =
this.mapWithId { _, receiver -> receiver.minus(other) }
@JvmName("minus_with_Long_and_Field_of_Int_end")
public operator fun Long.minus(other: Field): Field =
other.mapWithId { id, receiver -> this.minus(other[id]) }
@JvmName("minus_with_Field_of_Long_end_and_Field_of_Int_end")
public operator fun Field.minus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.minus(other[id]) }
}
@JvmName("minus_with_Field_of_Long_end_and_Long")
public operator fun Field.minus(other: Long): Field =
this.mapWithId { _, receiver -> receiver.minus(other) }
@JvmName("minus_with_Long_and_Field_of_Long_end")
public operator fun Long.minus(other: Field): Field =
other.mapWithId { id, receiver -> this.minus(other[id]) }
@JvmName("minus_with_Field_of_Long_end_and_Field_of_Long_end")
public operator fun Field.minus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.minus(other[id]) }
}
@JvmName("minus_with_Field_of_Long_end_and_Short")
public operator fun Field.minus(other: Short): Field =
this.mapWithId { _, receiver -> receiver.minus(other) }
@JvmName("minus_with_Long_and_Field_of_Short_end")
public operator fun Long.minus(other: Field): Field =
other.mapWithId { id, receiver -> this.minus(other[id]) }
@JvmName("minus_with_Field_of_Long_end_and_Field_of_Short_end")
public operator fun Field.minus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.minus(other[id]) }
}
@JvmName("or_with_Field_of_Long_end_and_Long")
public infix fun Field.or(other: Long): Field =
this.mapWithId { _, receiver -> receiver.or(other) }
@JvmName("or_with_Long_and_Field_of_Long_end")
public infix fun Long.or(other: Field): Field =
other.mapWithId { id, receiver -> this.or(other[id]) }
@JvmName("or_with_Field_of_Long_end_and_Field_of_Long_end")
public infix fun Field.or(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.or(other[id]) }
}
@JvmName("plus_with_Field_of_Long_end_and_Byte")
public operator fun Field.plus(other: Byte): Field =
this.mapWithId { _, receiver -> receiver.plus(other) }
@JvmName("plus_with_Long_and_Field_of_Byte_end")
public operator fun Long.plus(other: Field): Field =
other.mapWithId { id, receiver -> this.plus(other[id]) }
@JvmName("plus_with_Field_of_Long_end_and_Field_of_Byte_end")
public operator fun Field.plus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.plus(other[id]) }
}
@JvmName("plus_with_Field_of_Long_end_and_Double")
public operator fun Field.plus(other: Double): Field =
this.mapWithId { _, receiver -> receiver.plus(other) }
@JvmName("plus_with_Long_and_Field_of_Double_end")
public operator fun Long.plus(other: Field): Field =
other.mapWithId { id, receiver -> this.plus(other[id]) }
@JvmName("plus_with_Field_of_Long_end_and_Field_of_Double_end")
public operator fun Field.plus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.plus(other[id]) }
}
@JvmName("plus_with_Field_of_Long_end_and_Float")
public operator fun Field.plus(other: Float): Field =
this.mapWithId { _, receiver -> receiver.plus(other) }
@JvmName("plus_with_Long_and_Field_of_Float_end")
public operator fun Long.plus(other: Field): Field =
other.mapWithId { id, receiver -> this.plus(other[id]) }
@JvmName("plus_with_Field_of_Long_end_and_Field_of_Float_end")
public operator fun Field.plus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.plus(other[id]) }
}
@JvmName("plus_with_Field_of_Long_end_and_Int")
public operator fun Field.plus(other: Int): Field =
this.mapWithId { _, receiver -> receiver.plus(other) }
@JvmName("plus_with_Long_and_Field_of_Int_end")
public operator fun Long.plus(other: Field): Field =
other.mapWithId { id, receiver -> this.plus(other[id]) }
@JvmName("plus_with_Field_of_Long_end_and_Field_of_Int_end")
public operator fun Field.plus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.plus(other[id]) }
}
@JvmName("plus_with_Field_of_Long_end_and_Long")
public operator fun Field.plus(other: Long): Field =
this.mapWithId { _, receiver -> receiver.plus(other) }
@JvmName("plus_with_Long_and_Field_of_Long_end")
public operator fun Long.plus(other: Field): Field =
other.mapWithId { id, receiver -> this.plus(other[id]) }
@JvmName("plus_with_Field_of_Long_end_and_Field_of_Long_end")
public operator fun Field