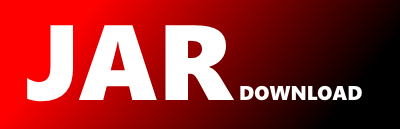
commonMain.it.unibo.collektive.stdlib.maps.FieldedMapsExtensions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stdlib-jvm Show documentation
Show all versions of stdlib-jvm Show documentation
DSL for Aggregate Computing in Kotlin
// This file is auto-generated by the Collektive code generator. Do not edit it manually.
@file:Suppress("all","ktlint")
package it.unibo.collektive.stdlib.maps
import it.unibo.collektive.`field`.Field
import it.unibo.collektive.`field`.Field.Companion.checkAligned
import kotlin.Any
import kotlin.Boolean
import kotlin.Function1
import kotlin.Function2
import kotlin.Int
import kotlin.Pair
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Iterable
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
import kotlin.sequences.Sequence
public object FieldedMapsExtensions {
@JvmName("all_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end")
public inline fun Field>.all(crossinline
predicate: Function1, Boolean>): Field =
this.mapWithId { _, receiver -> receiver.all(predicate) }
@JvmName("all_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end_end")
public fun
Map.all(predicate: Field, Boolean>>):
Field = predicate.mapWithId { id, receiver -> this.all(predicate[id]) }
@JvmName("all_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end_end")
public fun
Field>.all(predicate: Field, Boolean>>):
Field {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.all(predicate[id]) }
}
@JvmName("any_with_Field_of_collections_Map_of_out K_and_V_end_end")
public fun Field>.any(): Field = map { it.any() }
@JvmName("any_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end")
public inline fun Field>.any(crossinline
predicate: Function1, Boolean>): Field =
this.mapWithId { _, receiver -> receiver.any(predicate) }
@JvmName("any_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end_end")
public fun
Map.any(predicate: Field, Boolean>>):
Field = predicate.mapWithId { id, receiver -> this.any(predicate[id]) }
@JvmName("any_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end_end")
public fun
Field>.any(predicate: Field, Boolean>>):
Field {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.any(predicate[id]) }
}
@JvmName("count_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end")
public inline fun Field>.count(crossinline
predicate: Function1, Boolean>): Field =
this.mapWithId { _, receiver -> receiver.count(predicate) }
@JvmName("count_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end_end")
public fun
Map.count(predicate: Field, Boolean>>): Field
= predicate.mapWithId { id, receiver -> this.count(predicate[id]) }
@JvmName("count_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end_end")
public fun
Field>.count(predicate: Field, Boolean>>):
Field {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.count(predicate[id]) }
}
@JvmName("filter_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end")
public inline fun Field>.filter(crossinline
predicate: Function1, Boolean>): Field> =
this.mapWithId { _, receiver -> receiver.filter(predicate) }
@JvmName("filter_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end_end")
public fun
Map.filter(predicate: Field, Boolean>>):
Field> = predicate.mapWithId { id, receiver -> this.filter(predicate[id]) }
@JvmName("filter_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end_end")
public fun
Field>.filter(predicate: Field, Boolean>>):
Field> {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.filter(predicate[id]) }
}
@JvmName("filterKeys_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_K_and_Boolean_end")
public inline fun Field>.filterKeys(crossinline
predicate: Function1): Field> =
this.mapWithId { _, receiver -> receiver.filterKeys(predicate) }
@JvmName("filterKeys_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_K_and_Boolean_end_end")
public fun Map.filterKeys(predicate: Field>):
Field> = predicate.mapWithId { id, receiver -> this.filterKeys(predicate[id]) }
@JvmName("filterKeys_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_K_and_Boolean_end_end")
public fun
Field>.filterKeys(predicate: Field>):
Field> {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.filterKeys(predicate[id]) }
}
@JvmName("filterNot_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end")
public inline fun Field>.filterNot(crossinline
predicate: Function1, Boolean>): Field> =
this.mapWithId { _, receiver -> receiver.filterNot(predicate) }
@JvmName("filterNot_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end_end")
public fun
Map.filterNot(predicate: Field, Boolean>>):
Field> = predicate.mapWithId { id, receiver -> this.filterNot(predicate[id]) }
@JvmName("filterNot_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end_end")
public fun
Field>.filterNot(predicate: Field, Boolean>>):
Field> {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.filterNot(predicate[id]) }
}
@JvmName("filterValues_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_V_and_Boolean_end")
public inline fun Field>.filterValues(crossinline
predicate: Function1): Field> =
this.mapWithId { _, receiver -> receiver.filterValues(predicate) }
@JvmName("filterValues_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_V_and_Boolean_end_end")
public fun
Map.filterValues(predicate: Field>): Field>
= predicate.mapWithId { id, receiver -> this.filterValues(predicate[id]) }
@JvmName("filterValues_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_V_and_Boolean_end_end")
public fun
Field>.filterValues(predicate: Field>):
Field> {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.filterValues(predicate[id]) }
}
@JvmName("flatMap_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_collections_Map_Entry_of_K_and_V_end_and_collections_Iterable_of_R_end_end")
public inline fun Field>.flatMap(crossinline
transform: Function1, Iterable>): Field> =
this.mapWithId { _, receiver -> receiver.flatMap(transform) }
@JvmName("flatMap_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_collections_Iterable_of_R_end_end_end")
public fun
Map.flatMap(transform: Field, Iterable>>):
Field> = transform.mapWithId { id, receiver -> this.flatMap(transform[id]) }
@JvmName("flatMap_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_collections_Iterable_of_R_end_end_end")
public fun
Field>.flatMap(transform: Field, Iterable>>):
Field> {
checkAligned(this, transform)
return this.mapWithId { id, receiver -> receiver.flatMap(transform[id]) }
}
@JvmName("flatMap_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_collections_Map_Entry_of_K_and_V_end_and_sequences_Sequence_of_R_end_end")
public inline fun Field>.flatMap(crossinline
transform: Function1, Sequence>): Field> =
this.mapWithId { _, receiver -> receiver.flatMap(transform) }
@JvmName("flatMap_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_sequences_Sequence_of_R_end_end_end")
public fun
Map.flatMap(transform: Field, Sequence>>):
Field> = transform.mapWithId { id, receiver -> this.flatMap(transform[id]) }
@JvmName("flatMap_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_sequences_Sequence_of_R_end_end_end")
public fun
Field>.flatMap(transform: Field, Sequence>>):
Field> {
checkAligned(this, transform)
return this.mapWithId { id, receiver -> receiver.flatMap(transform[id]) }
}
@JvmName("getValue_with_Field_of_collections_Map_of_K_and_V_end_end_and_K")
public fun Field>.getValue(key: K): Field =
this.mapWithId { _, receiver -> receiver.getValue(key) }
@JvmName("getValue_with_collections_Map_of_K_and_V_end_and_Field_of_K_end")
public fun Map.getValue(key: Field): Field =
key.mapWithId { id, receiver -> this.getValue(key[id]) }
@JvmName("getValue_with_Field_of_collections_Map_of_K_and_V_end_end_and_Field_of_K_end")
public fun Field>.getValue(key: Field): Field {
checkAligned(this, key)
return this.mapWithId { id, receiver -> receiver.getValue(key[id]) }
}
@JvmName("mapKeys_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_collections_Map_Entry_of_K_and_V_end_and_R_end")
public inline fun Field>.mapKeys(crossinline
transform: Function1, R>): Field> =
this.mapWithId { _, receiver -> receiver.mapKeys(transform) }
@JvmName("mapKeys_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_R_end_end")
public fun
Map.mapKeys(transform: Field, R>>):
Field> = transform.mapWithId { id, receiver -> this.mapKeys(transform[id]) }
@JvmName("mapKeys_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_R_end_end")
public fun
Field>.mapKeys(transform: Field, R>>):
Field> {
checkAligned(this, transform)
return this.mapWithId { id, receiver -> receiver.mapKeys(transform[id]) }
}
@JvmName("mapNotNull_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_collections_Map_Entry_of_K_and_V_end_and_R_nullable_end")
public inline fun Field>.mapNotNull(crossinline
transform: Function1, R?>): Field> =
this.mapWithId { _, receiver -> receiver.mapNotNull(transform) }
@JvmName("mapNotNull_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_R_nullable_end_end")
public fun
Map.mapNotNull(transform: Field, R?>>):
Field> = transform.mapWithId { id, receiver -> this.mapNotNull(transform[id]) }
@JvmName("mapNotNull_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_R_nullable_end_end")
public fun
Field>.mapNotNull(transform: Field, R?>>):
Field> {
checkAligned(this, transform)
return this.mapWithId { id, receiver -> receiver.mapNotNull(transform[id]) }
}
@JvmName("mapValues_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_collections_Map_Entry_of_K_and_V_end_and_R_end")
public inline fun Field>.mapValues(crossinline
transform: Function1, R>): Field> =
this.mapWithId { _, receiver -> receiver.mapValues(transform) }
@JvmName("mapValues_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_R_end_end")
public fun
Map.mapValues(transform: Field, R>>):
Field> = transform.mapWithId { id, receiver -> this.mapValues(transform[id]) }
@JvmName("mapValues_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_R_end_end")
public fun
Field>.mapValues(transform: Field, R>>):
Field> {
checkAligned(this, transform)
return this.mapWithId { id, receiver -> receiver.mapValues(transform[id]) }
}
@JvmName("minus_with_Field_of_collections_Map_of_out K_and_V_end_end_and_K")
public operator fun Field>.minus(key: K): Field>
= this.mapWithId { _, receiver -> receiver.minus(key) }
@JvmName("minus_with_collections_Map_of_out K_and_V_end_and_Field_of_K_end")
public operator fun Map.minus(key: Field): Field>
= key.mapWithId { id, receiver -> this.minus(key[id]) }
@JvmName("minus_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_K_end")
public operator fun Field>.minus(key: Field):
Field> {
checkAligned(this, key)
return this.mapWithId { id, receiver -> receiver.minus(key[id]) }
}
@JvmName("minus_with_Field_of_collections_Map_of_out K_and_V_end_end_and_collections_Iterable_of_K_end")
public operator fun Field>.minus(keys: Iterable):
Field> = this.mapWithId { _, receiver -> receiver.minus(keys) }
@JvmName("minus_with_collections_Map_of_out K_and_V_end_and_Field_of_collections_Iterable_of_K_end_end")
public operator fun Map.minus(keys: Field>):
Field> = keys.mapWithId { id, receiver -> this.minus(keys[id]) }
@JvmName("minus_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_collections_Iterable_of_K_end_end")
public operator fun Field>.minus(keys: Field>):
Field> {
checkAligned(this, keys)
return this.mapWithId { id, receiver -> receiver.minus(keys[id]) }
}
@JvmName("minus_with_Field_of_collections_Map_of_out K_and_V_end_end_and_sequences_Sequence_of_K_end")
public operator fun Field>.minus(keys: Sequence):
Field> = this.mapWithId { _, receiver -> receiver.minus(keys) }
@JvmName("minus_with_collections_Map_of_out K_and_V_end_and_Field_of_sequences_Sequence_of_K_end_end")
public operator fun Map.minus(keys: Field>):
Field> = keys.mapWithId { id, receiver -> this.minus(keys[id]) }
@JvmName("minus_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_sequences_Sequence_of_K_end_end")
public operator fun Field>.minus(keys: Field>):
Field> {
checkAligned(this, keys)
return this.mapWithId { id, receiver -> receiver.minus(keys[id]) }
}
@JvmName("none_with_Field_of_collections_Map_of_out K_and_V_end_end")
public fun Field>.none(): Field = map { it.none() }
@JvmName("none_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end")
public inline fun Field>.none(crossinline
predicate: Function1, Boolean>): Field =
this.mapWithId { _, receiver -> receiver.none(predicate) }
@JvmName("none_with_collections_Map_of_out K_and_V_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end_end")
public fun
Map.none(predicate: Field, Boolean>>):
Field = predicate.mapWithId { id, receiver -> this.none(predicate[id]) }
@JvmName("none_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Boolean_end_end")
public fun
Field>.none(predicate: Field, Boolean>>):
Field {
checkAligned(this, predicate)
return this.mapWithId { id, receiver -> receiver.none(predicate[id]) }
}
@JvmName("onEach_with_Field_of_M_end_and_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Unit_end")
public inline fun , ID : Any> Field.onEach(crossinline
action: Function1, Unit>): Field =
this.mapWithId { _, receiver -> receiver.onEach(action) }
@JvmName("onEach_with_M_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Unit_end_end")
public fun , ID : Any>
M.onEach(action: Field, Unit>>): Field =
action.mapWithId { id, receiver -> this.onEach(action[id]) }
@JvmName("onEach_with_Field_of_M_end_and_Field_of_Function1_of_collections_Map_Entry_of_K_and_V_end_and_Unit_end_end")
public fun , ID : Any>
Field.onEach(action: Field, Unit>>): Field {
checkAligned(this, action)
return this.mapWithId { id, receiver -> receiver.onEach(action[id]) }
}
@JvmName("onEachIndexed_with_Field_of_M_end_and_Function2_of_Int_and_collections_Map_Entry_of_K_and_V_end_and_Unit_end")
public inline fun , ID : Any> Field.onEachIndexed(crossinline
action: Function2, Unit>): Field =
this.mapWithId { _, receiver -> receiver.onEachIndexed(action) }
@JvmName("onEachIndexed_with_M_and_Field_of_Function2_of_Int_and_collections_Map_Entry_of_K_and_V_end_and_Unit_end_end")
public fun , ID : Any>
M.onEachIndexed(action: Field, Unit>>): Field =
action.mapWithId { id, receiver -> this.onEachIndexed(action[id]) }
@JvmName("onEachIndexed_with_Field_of_M_end_and_Field_of_Function2_of_Int_and_collections_Map_Entry_of_K_and_V_end_and_Unit_end_end")
public fun , ID : Any>
Field.onEachIndexed(action: Field, Unit>>):
Field {
checkAligned(this, action)
return this.mapWithId { id, receiver -> receiver.onEachIndexed(action[id]) }
}
@JvmName("plus_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Pair_of_K_and_V_end")
public operator fun Field>.plus(pair: Pair):
Field> = this.mapWithId { _, receiver -> receiver.plus(pair) }
@JvmName("plus_with_collections_Map_of_out K_and_V_end_and_Field_of_Pair_of_K_and_V_end_end")
public operator fun Map.plus(pair: Field>):
Field> = pair.mapWithId { id, receiver -> this.plus(pair[id]) }
@JvmName("plus_with_Field_of_collections_Map_of_out K_and_V_end_end_and_Field_of_Pair_of_K_and_V_end_end")
public operator fun Field>.plus(pair: Field>):
Field> {
checkAligned(this, pair)
return this.mapWithId { id, receiver -> receiver.plus(pair[id]) }
}
@JvmName("plus_with_Field_of_collections_Map_of_out K_and_V_end_end_and_collections_Iterable_of_Pair_of_K_and_V_end_end")
public operator fun