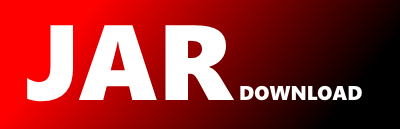
commonMain.it.unibo.collektive.stdlib.triples.FieldedTriples.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stdlib-jvm Show documentation
Show all versions of stdlib-jvm Show documentation
DSL for Aggregate Computing in Kotlin
// This file is auto-generated by the Collektive code generator. Do not edit it manually.
@file:Suppress("all","ktlint")
package it.unibo.collektive.stdlib.triples
import it.unibo.collektive.`field`.Field
import it.unibo.collektive.`field`.Field.Companion.checkAligned
import kotlin.Any
import kotlin.Suppress
import kotlin.Triple
import kotlin.jvm.JvmName
public object FieldedTriples {
@JvmName("component1_with_Field_of_Triple_of_A_and_B_and_C_end_end")
public operator fun Field>.component1(): Field =
map { it.component1() }
@JvmName("component2_with_Field_of_Triple_of_A_and_B_and_C_end_end")
public operator fun Field>.component2(): Field =
map { it.component2() }
@JvmName("component3_with_Field_of_Triple_of_A_and_B_and_C_end_end")
public operator fun Field>.component3(): Field =
map { it.component3() }
@JvmName("copy_with_Field_of_Triple_of_A_and_B_and_C_end_end_and_A_and_B_and_C")
public fun Field>.copy(
first: A,
second: B,
third: C,
): Field> =
this.mapWithId { _, receiver -> receiver.copy(first, second, third) }
@JvmName("copy_with_Triple_of_A_and_B_and_C_end_and_Field_of_A_end_and_B_and_C")
public fun Triple.copy(
first: Field,
second: B,
third: C,
): Field> =
first.mapWithId { id, receiver -> this.copy(first[id], second, third) }
@JvmName("copy_with_Field_of_Triple_of_A_and_B_and_C_end_end_and_Field_of_A_end_and_B_and_C")
public fun Field>.copy(
first: Field,
second: B,
third: C,
): Field> {
checkAligned(this, first)
return this.mapWithId { id, receiver -> receiver.copy(first[id], second, third) }
}
@JvmName("copy_with_Triple_of_A_and_B_and_C_end_and_A_and_Field_of_B_end_and_C")
public fun Triple.copy(
first: A,
second: Field,
third: C,
): Field> =
second.mapWithId { id, receiver -> this.copy(first, second[id], third) }
@JvmName("copy_with_Field_of_Triple_of_A_and_B_and_C_end_end_and_A_and_Field_of_B_end_and_C")
public fun Field>.copy(
first: A,
second: Field,
third: C,
): Field> {
checkAligned(this, second)
return this.mapWithId { id, receiver -> receiver.copy(first, second[id], third) }
}
@JvmName("copy_with_Triple_of_A_and_B_and_C_end_and_Field_of_A_end_and_Field_of_B_end_and_C")
public fun Triple.copy(
first: Field,
second: Field,
third: C,
): Field> {
checkAligned(first, second)
return first.mapWithId { id, receiver -> this.copy(first[id], second[id], third) }
}
@JvmName("copy_with_Field_of_Triple_of_A_and_B_and_C_end_end_and_Field_of_A_end_and_Field_of_B_end_and_C")
public fun Field>.copy(
first: Field,
second: Field,
third: C,
): Field> {
checkAligned(this, first, second)
return this.mapWithId { id, receiver -> receiver.copy(first[id], second[id], third) }
}
@JvmName("copy_with_Triple_of_A_and_B_and_C_end_and_A_and_B_and_Field_of_C_end")
public fun Triple.copy(
first: A,
second: B,
third: Field,
): Field> =
third.mapWithId { id, receiver -> this.copy(first, second, third[id]) }
@JvmName("copy_with_Field_of_Triple_of_A_and_B_and_C_end_end_and_A_and_B_and_Field_of_C_end")
public fun Field>.copy(
first: A,
second: B,
third: Field,
): Field> {
checkAligned(this, third)
return this.mapWithId { id, receiver -> receiver.copy(first, second, third[id]) }
}
@JvmName("copy_with_Triple_of_A_and_B_and_C_end_and_Field_of_A_end_and_B_and_Field_of_C_end")
public fun Triple.copy(
first: Field,
second: B,
third: Field,
): Field> {
checkAligned(first, third)
return first.mapWithId { id, receiver -> this.copy(first[id], second, third[id]) }
}
@JvmName("copy_with_Field_of_Triple_of_A_and_B_and_C_end_end_and_Field_of_A_end_and_B_and_Field_of_C_end")
public fun Field>.copy(
first: Field,
second: B,
third: Field,
): Field> {
checkAligned(this, first, third)
return this.mapWithId { id, receiver -> receiver.copy(first[id], second, third[id]) }
}
@JvmName("copy_with_Triple_of_A_and_B_and_C_end_and_A_and_Field_of_B_end_and_Field_of_C_end")
public fun Triple.copy(
first: A,
second: Field,
third: Field,
): Field> {
checkAligned(second, third)
return second.mapWithId { id, receiver -> this.copy(first, second[id], third[id]) }
}
@JvmName("copy_with_Field_of_Triple_of_A_and_B_and_C_end_end_and_A_and_Field_of_B_end_and_Field_of_C_end")
public fun Field>.copy(
first: A,
second: Field,
third: Field,
): Field> {
checkAligned(this, second, third)
return this.mapWithId { id, receiver -> receiver.copy(first, second[id], third[id]) }
}
@JvmName("copy_with_Triple_of_A_and_B_and_C_end_and_Field_of_A_end_and_Field_of_B_end_and_Field_of_C_end")
public fun Triple.copy(
first: Field,
second: Field,
third: Field,
): Field> {
checkAligned(first, second, third)
return first.mapWithId { id, receiver -> this.copy(first[id], second[id], third[id]) }
}
@JvmName("copy_with_Field_of_Triple_of_A_and_B_and_C_end_end_and_Field_of_A_end_and_Field_of_B_end_and_Field_of_C_end")
public fun Field>.copy(
first: Field,
second: Field,
third: Field,
): Field> {
checkAligned(this, first, second, third)
return this.mapWithId { id, receiver -> receiver.copy(first[id], second[id], third[id]) }
}
@JvmName("first_with_Field_of_Triple_of_A_and_B_and_C_end_end")
public fun Field>.first(): Field = map { it.first }
@JvmName("second_with_Field_of_Triple_of_A_and_B_and_C_end_end")
public fun Field>.second(): Field =
map { it.second }
@JvmName("third_with_Field_of_Triple_of_A_and_B_and_C_end_end")
public fun Field>.third(): Field = map { it.third }
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy