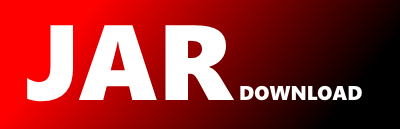
commonMain.it.unibo.collektive.stdlib.ulongs.FieldedULongs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of stdlib-jvm Show documentation
Show all versions of stdlib-jvm Show documentation
DSL for Aggregate Computing in Kotlin
// This file is auto-generated by the Collektive code generator. Do not edit it manually.
@file:Suppress("all","ktlint")
package it.unibo.collektive.stdlib.ulongs
import it.unibo.collektive.`field`.Field
import it.unibo.collektive.`field`.Field.Companion.checkAligned
import kotlin.Any
import kotlin.Byte
import kotlin.Double
import kotlin.Float
import kotlin.Int
import kotlin.Long
import kotlin.Short
import kotlin.Suppress
import kotlin.UByte
import kotlin.UInt
import kotlin.ULong
import kotlin.UShort
import kotlin.jvm.JvmName
import kotlin.ranges.ULongRange
public object FieldedULongs {
@JvmName("and_with_Field_of_ULong_end_and_ULong")
public infix fun Field.and(other: ULong): Field =
this.mapWithId { _, receiver -> receiver.and(other) }
@JvmName("and_with_ULong_and_Field_of_ULong_end")
public infix fun ULong.and(other: Field): Field =
other.mapWithId { id, receiver -> this.and(other[id]) }
@JvmName("and_with_Field_of_ULong_end_and_Field_of_ULong_end")
public infix fun Field.and(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.and(other[id]) }
}
@JvmName("compareTo_with_Field_of_ULong_end_and_UByte")
public fun Field.compareTo(other: UByte): Field =
this.mapWithId { _, receiver -> receiver.compareTo(other) }
@JvmName("compareTo_with_ULong_and_Field_of_UByte_end")
public fun ULong.compareTo(other: Field): Field =
other.mapWithId { id, receiver -> this.compareTo(other[id]) }
@JvmName("compareTo_with_Field_of_ULong_end_and_Field_of_UByte_end")
public fun Field.compareTo(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.compareTo(other[id]) }
}
@JvmName("compareTo_with_Field_of_ULong_end_and_UInt")
public fun Field.compareTo(other: UInt): Field =
this.mapWithId { _, receiver -> receiver.compareTo(other) }
@JvmName("compareTo_with_ULong_and_Field_of_UInt_end")
public fun ULong.compareTo(other: Field): Field =
other.mapWithId { id, receiver -> this.compareTo(other[id]) }
@JvmName("compareTo_with_Field_of_ULong_end_and_Field_of_UInt_end")
public fun Field.compareTo(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.compareTo(other[id]) }
}
@JvmName("compareTo_with_Field_of_ULong_end_and_ULong")
public fun Field.compareTo(other: ULong): Field =
this.mapWithId { _, receiver -> receiver.compareTo(other) }
@JvmName("compareTo_with_ULong_and_Field_of_ULong_end")
public fun ULong.compareTo(other: Field): Field =
other.mapWithId { id, receiver -> this.compareTo(other[id]) }
@JvmName("compareTo_with_Field_of_ULong_end_and_Field_of_ULong_end")
public fun Field.compareTo(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.compareTo(other[id]) }
}
@JvmName("compareTo_with_Field_of_ULong_end_and_UShort")
public fun Field.compareTo(other: UShort): Field =
this.mapWithId { _, receiver -> receiver.compareTo(other) }
@JvmName("compareTo_with_ULong_and_Field_of_UShort_end")
public fun ULong.compareTo(other: Field): Field =
other.mapWithId { id, receiver -> this.compareTo(other[id]) }
@JvmName("compareTo_with_Field_of_ULong_end_and_Field_of_UShort_end")
public fun Field.compareTo(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.compareTo(other[id]) }
}
@JvmName("div_with_Field_of_ULong_end_and_UByte")
public operator fun Field.div(other: UByte): Field =
this.mapWithId { _, receiver -> receiver.div(other) }
@JvmName("div_with_ULong_and_Field_of_UByte_end")
public operator fun ULong.div(other: Field): Field =
other.mapWithId { id, receiver -> this.div(other[id]) }
@JvmName("div_with_Field_of_ULong_end_and_Field_of_UByte_end")
public operator fun Field.div(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.div(other[id]) }
}
@JvmName("div_with_Field_of_ULong_end_and_UInt")
public operator fun Field.div(other: UInt): Field =
this.mapWithId { _, receiver -> receiver.div(other) }
@JvmName("div_with_ULong_and_Field_of_UInt_end")
public operator fun ULong.div(other: Field): Field =
other.mapWithId { id, receiver -> this.div(other[id]) }
@JvmName("div_with_Field_of_ULong_end_and_Field_of_UInt_end")
public operator fun Field.div(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.div(other[id]) }
}
@JvmName("div_with_Field_of_ULong_end_and_ULong")
public operator fun Field.div(other: ULong): Field =
this.mapWithId { _, receiver -> receiver.div(other) }
@JvmName("div_with_ULong_and_Field_of_ULong_end")
public operator fun ULong.div(other: Field): Field =
other.mapWithId { id, receiver -> this.div(other[id]) }
@JvmName("div_with_Field_of_ULong_end_and_Field_of_ULong_end")
public operator fun Field.div(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.div(other[id]) }
}
@JvmName("div_with_Field_of_ULong_end_and_UShort")
public operator fun Field.div(other: UShort): Field =
this.mapWithId { _, receiver -> receiver.div(other) }
@JvmName("div_with_ULong_and_Field_of_UShort_end")
public operator fun ULong.div(other: Field): Field =
other.mapWithId { id, receiver -> this.div(other[id]) }
@JvmName("div_with_Field_of_ULong_end_and_Field_of_UShort_end")
public operator fun Field.div(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.div(other[id]) }
}
@JvmName("floorDiv_with_Field_of_ULong_end_and_UByte")
public fun Field.floorDiv(other: UByte): Field =
this.mapWithId { _, receiver -> receiver.floorDiv(other) }
@JvmName("floorDiv_with_ULong_and_Field_of_UByte_end")
public fun ULong.floorDiv(other: Field): Field =
other.mapWithId { id, receiver -> this.floorDiv(other[id]) }
@JvmName("floorDiv_with_Field_of_ULong_end_and_Field_of_UByte_end")
public fun Field.floorDiv(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.floorDiv(other[id]) }
}
@JvmName("floorDiv_with_Field_of_ULong_end_and_UInt")
public fun Field.floorDiv(other: UInt): Field =
this.mapWithId { _, receiver -> receiver.floorDiv(other) }
@JvmName("floorDiv_with_ULong_and_Field_of_UInt_end")
public fun ULong.floorDiv(other: Field): Field =
other.mapWithId { id, receiver -> this.floorDiv(other[id]) }
@JvmName("floorDiv_with_Field_of_ULong_end_and_Field_of_UInt_end")
public fun Field.floorDiv(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.floorDiv(other[id]) }
}
@JvmName("floorDiv_with_Field_of_ULong_end_and_ULong")
public fun Field.floorDiv(other: ULong): Field =
this.mapWithId { _, receiver -> receiver.floorDiv(other) }
@JvmName("floorDiv_with_ULong_and_Field_of_ULong_end")
public fun ULong.floorDiv(other: Field): Field =
other.mapWithId { id, receiver -> this.floorDiv(other[id]) }
@JvmName("floorDiv_with_Field_of_ULong_end_and_Field_of_ULong_end")
public fun Field.floorDiv(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.floorDiv(other[id]) }
}
@JvmName("floorDiv_with_Field_of_ULong_end_and_UShort")
public fun Field.floorDiv(other: UShort): Field =
this.mapWithId { _, receiver -> receiver.floorDiv(other) }
@JvmName("floorDiv_with_ULong_and_Field_of_UShort_end")
public fun ULong.floorDiv(other: Field): Field =
other.mapWithId { id, receiver -> this.floorDiv(other[id]) }
@JvmName("floorDiv_with_Field_of_ULong_end_and_Field_of_UShort_end")
public fun Field.floorDiv(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.floorDiv(other[id]) }
}
@JvmName("inv_with_Field_of_ULong_end")
public fun Field.inv(): Field = map { it.inv() }
@JvmName("minus_with_Field_of_ULong_end_and_UByte")
public operator fun Field.minus(other: UByte): Field =
this.mapWithId { _, receiver -> receiver.minus(other) }
@JvmName("minus_with_ULong_and_Field_of_UByte_end")
public operator fun ULong.minus(other: Field): Field =
other.mapWithId { id, receiver -> this.minus(other[id]) }
@JvmName("minus_with_Field_of_ULong_end_and_Field_of_UByte_end")
public operator fun Field.minus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.minus(other[id]) }
}
@JvmName("minus_with_Field_of_ULong_end_and_UInt")
public operator fun Field.minus(other: UInt): Field =
this.mapWithId { _, receiver -> receiver.minus(other) }
@JvmName("minus_with_ULong_and_Field_of_UInt_end")
public operator fun ULong.minus(other: Field): Field =
other.mapWithId { id, receiver -> this.minus(other[id]) }
@JvmName("minus_with_Field_of_ULong_end_and_Field_of_UInt_end")
public operator fun Field.minus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.minus(other[id]) }
}
@JvmName("minus_with_Field_of_ULong_end_and_ULong")
public operator fun Field.minus(other: ULong): Field =
this.mapWithId { _, receiver -> receiver.minus(other) }
@JvmName("minus_with_ULong_and_Field_of_ULong_end")
public operator fun ULong.minus(other: Field): Field =
other.mapWithId { id, receiver -> this.minus(other[id]) }
@JvmName("minus_with_Field_of_ULong_end_and_Field_of_ULong_end")
public operator fun Field.minus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.minus(other[id]) }
}
@JvmName("minus_with_Field_of_ULong_end_and_UShort")
public operator fun Field.minus(other: UShort): Field =
this.mapWithId { _, receiver -> receiver.minus(other) }
@JvmName("minus_with_ULong_and_Field_of_UShort_end")
public operator fun ULong.minus(other: Field): Field =
other.mapWithId { id, receiver -> this.minus(other[id]) }
@JvmName("minus_with_Field_of_ULong_end_and_Field_of_UShort_end")
public operator fun Field.minus(other: Field):
Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.minus(other[id]) }
}
@JvmName("mod_with_Field_of_ULong_end_and_UByte")
public fun Field.mod(other: UByte): Field =
this.mapWithId { _, receiver -> receiver.mod(other) }
@JvmName("mod_with_ULong_and_Field_of_UByte_end")
public fun ULong.mod(other: Field): Field =
other.mapWithId { id, receiver -> this.mod(other[id]) }
@JvmName("mod_with_Field_of_ULong_end_and_Field_of_UByte_end")
public fun Field.mod(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.mod(other[id]) }
}
@JvmName("mod_with_Field_of_ULong_end_and_UInt")
public fun Field.mod(other: UInt): Field =
this.mapWithId { _, receiver -> receiver.mod(other) }
@JvmName("mod_with_ULong_and_Field_of_UInt_end")
public fun ULong.mod(other: Field): Field =
other.mapWithId { id, receiver -> this.mod(other[id]) }
@JvmName("mod_with_Field_of_ULong_end_and_Field_of_UInt_end")
public fun Field.mod(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.mod(other[id]) }
}
@JvmName("mod_with_Field_of_ULong_end_and_ULong")
public fun Field.mod(other: ULong): Field =
this.mapWithId { _, receiver -> receiver.mod(other) }
@JvmName("mod_with_ULong_and_Field_of_ULong_end")
public fun ULong.mod(other: Field): Field =
other.mapWithId { id, receiver -> this.mod(other[id]) }
@JvmName("mod_with_Field_of_ULong_end_and_Field_of_ULong_end")
public fun Field.mod(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.mod(other[id]) }
}
@JvmName("mod_with_Field_of_ULong_end_and_UShort")
public fun Field.mod(other: UShort): Field =
this.mapWithId { _, receiver -> receiver.mod(other) }
@JvmName("mod_with_ULong_and_Field_of_UShort_end")
public fun ULong.mod(other: Field): Field =
other.mapWithId { id, receiver -> this.mod(other[id]) }
@JvmName("mod_with_Field_of_ULong_end_and_Field_of_UShort_end")
public fun Field.mod(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.mod(other[id]) }
}
@JvmName("or_with_Field_of_ULong_end_and_ULong")
public infix fun Field.or(other: ULong): Field =
this.mapWithId { _, receiver -> receiver.or(other) }
@JvmName("or_with_ULong_and_Field_of_ULong_end")
public infix fun ULong.or(other: Field): Field =
other.mapWithId { id, receiver -> this.or(other[id]) }
@JvmName("or_with_Field_of_ULong_end_and_Field_of_ULong_end")
public infix fun Field.or(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.or(other[id]) }
}
@JvmName("plus_with_Field_of_ULong_end_and_UByte")
public operator fun Field.plus(other: UByte): Field =
this.mapWithId { _, receiver -> receiver.plus(other) }
@JvmName("plus_with_ULong_and_Field_of_UByte_end")
public operator fun ULong.plus(other: Field): Field =
other.mapWithId { id, receiver -> this.plus(other[id]) }
@JvmName("plus_with_Field_of_ULong_end_and_Field_of_UByte_end")
public operator fun Field.plus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.plus(other[id]) }
}
@JvmName("plus_with_Field_of_ULong_end_and_UInt")
public operator fun Field.plus(other: UInt): Field =
this.mapWithId { _, receiver -> receiver.plus(other) }
@JvmName("plus_with_ULong_and_Field_of_UInt_end")
public operator fun ULong.plus(other: Field): Field =
other.mapWithId { id, receiver -> this.plus(other[id]) }
@JvmName("plus_with_Field_of_ULong_end_and_Field_of_UInt_end")
public operator fun Field.plus(other: Field): Field {
checkAligned(this, other)
return this.mapWithId { id, receiver -> receiver.plus(other[id]) }
}
@JvmName("plus_with_Field_of_ULong_end_and_ULong")
public operator fun Field.plus(other: ULong): Field =
this.mapWithId { _, receiver -> receiver.plus(other) }
@JvmName("plus_with_ULong_and_Field_of_ULong_end")
public operator fun ULong.plus(other: Field): Field =
other.mapWithId { id, receiver -> this.plus(other[id]) }
@JvmName("plus_with_Field_of_ULong_end_and_Field_of_ULong_end")
public operator fun Field