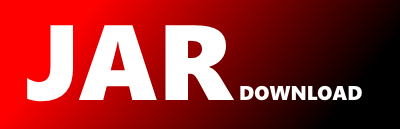
commonMain.it.unibo.tuprolog.bdd.BinaryDecisionDiagramOperators.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bdd-jvm Show documentation
Show all versions of bdd-jvm Show documentation
Multi-platform library for representing and manipulating Binary Decision Diagrams
/**
* @author Jason Dellaluce
*/
@file:JvmName("BinaryDecisionDiagramOperators")
package it.unibo.tuprolog.bdd
import it.unibo.tuprolog.bdd.impl.BinaryApplyExpansionVisitor
import it.unibo.tuprolog.bdd.impl.UnaryApplyExpansionVisitor
import kotlin.js.JsName
import kotlin.jvm.JvmName
/**
* Applies the "Apply" construction algorithm over [BinaryDecisionDiagram]s
* using a given boolean operator. The result is a Reduced Ordered Binary
* Decision Diagram (ROBDD).
* */
@JsName("applyUnary")
fun > BinaryDecisionDiagram.apply(
unaryOp: (Boolean) -> Boolean
): BinaryDecisionDiagram {
return runOperationAndCatchErrors {
this.applyThenExpansion(
unaryOp,
0,
0
) { _, _, _ -> 0 }
}.first
}
/**
* Applies the "Apply" construction algorithm over two [BinaryDecisionDiagram]s
* using a given boolean operator. The result is a Reduced Ordered Binary
* Decision Diagram (ROBDD).
* */
@JsName("applyBinary")
fun > BinaryDecisionDiagram.apply(
that: BinaryDecisionDiagram,
binaryOp: (Boolean, Boolean) -> Boolean
): BinaryDecisionDiagram {
return runOperationAndCatchErrors {
this.applyThenExpansion(
that,
binaryOp,
0,
0
) { _, _, _ -> 0 }
}.first
}
/**
* Applies the "Apply" construction algorithm over [BinaryDecisionDiagram]s
* using a given boolean operator, and computes a value using the Shannon
* Expansion over the result. The result is an instance of [Pair] of which
* [Pair.first] is the Reduced Ordered Binary Decision Diagram (ROBDD) produced
* by the operation, and [Pair.second] is the value of type [T] computed with
* the Shannon Expansion.
*
* By definition, invoking [apply] and then [expansion] should produce the same
* result.
* */
@JsName("applyUnaryThenExpansion")
fun , E> BinaryDecisionDiagram.applyThenExpansion(
unaryOp: (Boolean) -> Boolean,
expansionFalseTerminal: E,
expansionTrueTerminal: E,
expansionOperator: (node: T, low: E, high: E) -> E
): Pair, E> {
return runOperationAndCatchErrors {
this.accept(
UnaryApplyExpansionVisitor(
BinaryDecisionDiagramBuilder.reducedOf(),
unaryOp,
expansionFalseTerminal,
expansionTrueTerminal,
expansionOperator
)
)
}
}
/**
* Applies the "Apply" construction algorithm over two [BinaryDecisionDiagram]s
* using a given boolean operator, and computes a value using the Shannon
* Expansion over the result. The result is an instance of [Pair] of which
* [Pair.first] is the Reduced Ordered Binary Decision Diagram (ROBDD) produced
* by the operation, and [Pair.second] is the value of type [T] computed with
* the Shannon Expansion.
*
* By definition, invoking [apply] and then [expansion] should produce the same
* result.
* */
@JsName("applyBinaryThenExpansion")
fun , E> BinaryDecisionDiagram.applyThenExpansion(
that: BinaryDecisionDiagram,
binaryOp: (Boolean, Boolean) -> Boolean,
expansionFalseTerminal: E,
expansionTrueTerminal: E,
expansionOperator: (node: T, low: E, high: E) -> E
): Pair, E> {
return runOperationAndCatchErrors {
this.accept(
BinaryApplyExpansionVisitor(
BinaryDecisionDiagramBuilder.reducedOf(),
that,
binaryOp,
expansionFalseTerminal,
expansionTrueTerminal,
expansionOperator
)
)
}
}
/**
* Performs the "Not" unary boolean operation over a [BinaryDecisionDiagram].
* The result is a Reduced Ordered Binary Decision Diagram (ROBDD).
*/
@JsName("not")
fun > BinaryDecisionDiagram.not(): BinaryDecisionDiagram {
return runOperationAndCatchErrors {
this.apply { a -> !a }
}
}
/**
* Performs the "Not" unary boolean operation over a [BinaryDecisionDiagram]
* and computes a value using the Shannon Expansion over the result.
* The result is an instance of [Pair] of which [Pair.first] is the
* Reduced Ordered Binary Decision Diagram (ROBDD) produced by the operation,
* and [Pair.second] is the value of type [T] computed with the
* Shannon Expansion.
*
* By definition, invoking [apply] and then [expansion] should produce the same
* result.
* */
@JsName("notThenExpansion")
fun , E> BinaryDecisionDiagram.notThenExpansion(
expansionFalseTerminal: E,
expansionTrueTerminal: E,
expansionOperator: (node: T, low: E, high: E) -> E
): Pair, E> {
return runOperationAndCatchErrors {
this.applyThenExpansion(
{ a -> !a },
expansionFalseTerminal,
expansionTrueTerminal,
expansionOperator
)
}
}
/**
* Performs the "And" unary boolean operation over two
* [BinaryDecisionDiagram]s. The result is a Reduced Ordered Binary
* Decision Diagram (ROBDD).
*/
@JsName("and")
infix fun > BinaryDecisionDiagram.and(
that: BinaryDecisionDiagram
): BinaryDecisionDiagram {
return runOperationAndCatchErrors {
this.apply(that) { a, b -> a && b }
}
}
/**
* Performs the "And" unary boolean operation over two [BinaryDecisionDiagram]s
* and computes a value using the Shannon Expansion over the result.
* The result is an instance of [Pair] of which [Pair.first] is the
* Reduced Ordered Binary Decision Diagram (ROBDD) produced by the operation,
* and [Pair.second] is the value of type [T] computed with the
* Shannon Expansion.
*
* By definition, invoking [apply] and then [expansion] should produce the same
* result.
* */
@JsName("andThenExpansion")
fun , E> BinaryDecisionDiagram.andThenExpansion(
that: BinaryDecisionDiagram,
expansionFalseTerminal: E,
expansionTrueTerminal: E,
expansionOperator: (node: T, low: E, high: E) -> E
): Pair, E> {
return runOperationAndCatchErrors {
this.applyThenExpansion(
that,
{ a, b -> a && b },
expansionFalseTerminal,
expansionTrueTerminal,
expansionOperator
)
}
}
/**
* Performs the "Or" unary boolean operation over two [BinaryDecisionDiagram]s.
* The result is a Reduced Ordered Binary Decision Diagram (ROBDD).
*/
@JsName("or")
infix fun > BinaryDecisionDiagram.or(
that: BinaryDecisionDiagram
): BinaryDecisionDiagram {
return runOperationAndCatchErrors {
this.apply(that) { a, b -> a || b }
}
}
/**
* Performs the "Or" unary boolean operation over two [BinaryDecisionDiagram]s
* and computes a value using the Shannon Expansion over the result.
* The result is an instance of [Pair] of which [Pair.first] is the
* Reduced Ordered Binary Decision Diagram (ROBDD) produced by the operation,
* and [Pair.second] is the value of type [T] computed with the
* Shannon Expansion.
*
* By definition, invoking [apply] and then [expansion] should produce the same
* result.
* */
@JsName("orThenExpansion")
fun , E> BinaryDecisionDiagram.orThenExpansion(
that: BinaryDecisionDiagram,
expansionFalseTerminal: E,
expansionTrueTerminal: E,
expansionOperator: (node: T, low: E, high: E) -> E
): Pair, E> {
return runOperationAndCatchErrors {
this.applyThenExpansion(
that,
{ a, b -> a || b },
expansionFalseTerminal,
expansionTrueTerminal,
expansionOperator
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy