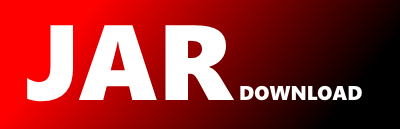
it.unibo.tuprolog.parser.TestPrologLexer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of parser-js Show documentation
Show all versions of parser-js Show documentation
Internal, JS-specific implementation of a Prolog syntax parser supporting dynamic operators definition
package it.unibo.tuprolog.parser
import kotlin.test.Test
import kotlin.test.assertEquals
import kotlin.test.assertFalse
import kotlin.test.assertTrue
class TestPrologLexer {
@Test
fun testInitialisation() {
assertEquals("PrologLexer.g4", PrologLexer.grammarFileName)
val modeNames = arrayOf("DEFAULT_MODE")
PrologLexer.modeNames.forEachIndexed { index, mode ->
assertEquals(modeNames[index], mode)
}
val channelNames = arrayOf("DEFAULT_TOKEN_CHANNEL", "HIDDEN")
PrologLexer.channelNames.forEachIndexed { index, channel ->
assertEquals(channelNames[index], channel)
}
val ruleNames = arrayOf(
"INTEGER", "HEX", "OCT", "BINARY", "SIGN",
"FLOAT", "CHAR", "BOOL", "LPAR", "RPAR",
"LSQUARE", "RSQUARE", "EMPTY_LIST",
"LBRACE", "RBRACE", "EMPTY_SET", "VARIABLE",
"SQ_STRING", "DQ_STRING", "COMMA", "PIPE",
"CUT", "FULL_STOP", "FullStopTerminator",
"WHITE_SPACES", "COMMENT", "LINE_COMMENT",
"OPERATOR", "ATOM", "Symbols", "Escapable",
"DoubleSQ", "DoubleDQ", "OpSymbol",
"Atom", "Ws", "OctDigit", "BinDigit",
"HexDigit", "Digit", "Zero"
)
PrologLexer.ruleNames.forEachIndexed { index, rule ->
assertEquals(ruleNames[index], rule)
}
}
@Test
fun testLexerRecognizeOperators() {
val lexer = PrologLexer("a ; b :- c")
val ops = listOf(";", ":-", "+")
lexer.addOperators(";", ":-", "+")
lexer.getOperators().forEachIndexed { index, op ->
assertEquals(ops[index], op)
}
assertTrue(lexer.isOperator(";"))
assertFalse(lexer.isOperator("jj"))
assertTrue(lexer.isOperator(":-"))
assertFalse(lexer.isOperator("+a"))
}
@Test
fun testLexerUnquote() {
val lexer = PrologLexer(null)
assertEquals("String", lexer.unquote("'String'"))
assertEquals("sec''sds", lexer.unquote("'sec''sds'"))
}
@Test
fun testLexerEscape() {
val lexer = PrologLexer(null)
lexer.escape("first\\nsec\\rthird", StringType.SINGLE_QUOTED)
assertEquals("first\nsec\rthird", lexer.escape("first\\nsec\\rthird", StringType.SINGLE_QUOTED))
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy