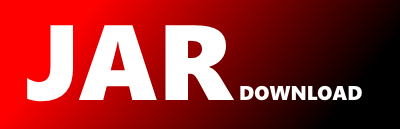
jvmMain.it.unibo.tuprolog.serialize.JvmTermObjectifier.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of serialize-core-jvm Show documentation
Show all versions of serialize-core-jvm Show documentation
JSON and YAML serialization support for logic terms and clauses
The newest version!
package it.unibo.tuprolog.serialize
import it.unibo.tuprolog.core.Atom
import it.unibo.tuprolog.core.Block
import it.unibo.tuprolog.core.Cons
import it.unibo.tuprolog.core.Directive
import it.unibo.tuprolog.core.EmptyBlock
import it.unibo.tuprolog.core.EmptyList
import it.unibo.tuprolog.core.Fact
import it.unibo.tuprolog.core.Indicator
import it.unibo.tuprolog.core.Integer
import it.unibo.tuprolog.core.List
import it.unibo.tuprolog.core.Real
import it.unibo.tuprolog.core.Rule
import it.unibo.tuprolog.core.Struct
import it.unibo.tuprolog.core.Term
import it.unibo.tuprolog.core.Truth
import it.unibo.tuprolog.core.Tuple
import it.unibo.tuprolog.core.Var
@Suppress("TooManyFunctions")
internal class JvmTermObjectifier : TermObjectifier {
override fun defaultValue(term: Term): Any {
error("Cannot objectify term: $term")
}
override fun objectifyMany(values: Iterable): Any {
return values.map { objectify(it) }
}
override fun visitVar(term: Var): Map =
mapOf(
"var" to term.name,
)
override fun visitTruth(term: Truth): Any =
when (term) {
Truth.TRUE -> true
Truth.FALSE -> false
Truth.FAIL -> "fail"
else -> NotImplementedError("Serialization of Truth value: $term")
}
override fun visitStruct(term: Struct): Map =
mapOf(
"fun" to term.functor,
"args" to term.args.map { it.accept(this) },
)
override fun visitAtom(term: Atom): String = term.value
@Suppress("SwallowedException")
override fun visitInteger(term: Integer): Any =
try {
term.value.toLongExact()
} catch (e: ArithmeticException) {
mapOf(
"integer" to term.value.toString(),
)
}
override fun visitReal(term: Real): Any =
mapOf(
"real" to term.value.toString(),
)
override fun visitBlock(term: Block): Map =
mapOf(
"block" to term.toList().map { it.accept(this) },
)
override fun visitEmptyBlock(term: EmptyBlock): Map = visitBlock(term)
override fun visitList(term: List): Map {
val listed = term.toList()
return if (term.isWellFormed) {
mapOf(
"list" to listed.map { it.accept(this) },
)
} else {
mapOf(
"list" to listed.subList(0, listed.lastIndex).map { it.accept(this) },
"tail" to listed[listed.lastIndex].accept(this),
)
}
}
override fun visitCons(term: Cons): Map = visitList(term)
override fun visitEmptyList(term: EmptyList): Map = visitList(term)
override fun visitTuple(term: Tuple): Map =
mapOf(
"tuple" to term.toList().map { it.accept(this) },
)
override fun visitIndicator(term: Indicator): Map =
mapOf(
"name" to term.nameTerm.accept(this),
"arity" to term.arityTerm.accept(this),
)
override fun visitRule(term: Rule): Map =
mapOf(
"head" to term.head.accept(this),
"body" to term.body.accept(this),
)
override fun visitFact(term: Fact): Map =
mapOf(
"head" to term.head.accept(this),
)
override fun visitDirective(term: Directive): Map =
mapOf(
"body" to term.body.accept(this),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy