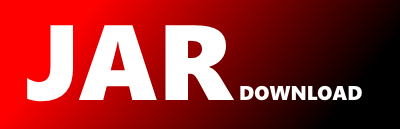
commonMain.it.unibo.tuprolog.solve.Utils.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of solve-jvm Show documentation
Show all versions of solve-jvm Show documentation
Resolution-agnostic API for logic solvers
@file:JvmName("Utils")
package it.unibo.tuprolog.solve
import it.unibo.tuprolog.core.Clause
import it.unibo.tuprolog.core.Directive
import it.unibo.tuprolog.core.Struct
import it.unibo.tuprolog.core.operators.Operator
import it.unibo.tuprolog.core.operators.OperatorSet
import it.unibo.tuprolog.solve.function.FunctionWrapper
import it.unibo.tuprolog.solve.function.LogicFunction
import it.unibo.tuprolog.solve.library.Library
import it.unibo.tuprolog.solve.library.Runtime
import it.unibo.tuprolog.solve.library.toRuntime
import it.unibo.tuprolog.solve.primitive.Primitive
import it.unibo.tuprolog.solve.primitive.PrimitiveWrapper
import it.unibo.tuprolog.solve.rule.RuleWrapper
import it.unibo.tuprolog.theory.Theory
import kotlin.js.JsName
import kotlin.jvm.JvmName
import kotlin.jvm.JvmOverloads
/** Performs the given [action] on each element, giving a lookahead hint (i.e. if there's another element to process after). */
inline fun Iterator.forEachWithLookahead(action: (T, Boolean) -> Unit) {
while (hasNext()) {
action(next(), hasNext())
}
}
/** Performs the given [action] on each element, giving a lookahead hint (i.e. if there's another element to process after). */
inline fun Iterable.forEachWithLookahead(action: (T, Boolean) -> Unit) = iterator().forEachWithLookahead(action)
/** Performs the given [action] on each element, giving a lookahead hint (i.e. if there's another element to process after). */
inline fun Sequence.forEachWithLookahead(action: (T, Boolean) -> Unit) = iterator().forEachWithLookahead(action)
fun Iterable.getAllOperators(): Sequence {
return asSequence()
.filterIsInstance()
.map { it.body }
.filterIsInstance()
.filter { it.arity == 3 && it.functor == "op" }
.map { Operator.fromTerm(it) }
.filterNotNull()
}
fun Library.getAllOperators(): Sequence {
return operators.asSequence()
}
fun Runtime.getAllOperators(): Sequence {
return operators.asSequence()
}
fun getAllOperators(
libraries: Runtime,
vararg theories: Theory,
): Sequence {
return libraries.getAllOperators() + sequenceOf(*theories).flatMap { it.getAllOperators() }
}
fun Sequence.toOperatorSet(): OperatorSet {
return OperatorSet(this)
}
@JvmOverloads
@JsName("libraryOf")
fun libraryOf(
alias: String? = null,
item1: AbstractWrapper<*>,
vararg items: AbstractWrapper<*>,
): Library {
val clauses = mutableListOf()
val primitives = mutableMapOf()
val functions = mutableMapOf()
for (item in arrayOf(item1, *items)) {
when (item) {
is PrimitiveWrapper<*> -> primitives += item.descriptionPair
is FunctionWrapper<*> -> functions += item.descriptionPair
is RuleWrapper<*> -> clauses.add(item.implementation)
else -> throw NotImplementedError("Cannot handle wrappers of type ${item::class}")
}
}
val library = Library.of(primitives, clauses, OperatorSet.EMPTY, functions)
return alias?.let { Library.of(it, library) } ?: library
}
@JvmOverloads
@JsName("runtimeOf")
fun runtimeOf(
alias: String? = null,
item1: AbstractWrapper<*>,
vararg items: AbstractWrapper<*>,
): Runtime {
return libraryOf(alias, item1, *items).toRuntime()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy