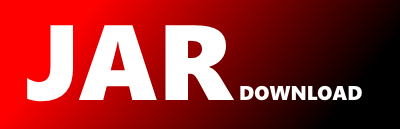
it.unibz.inf.ontop.dbschema.QuotedIDFactoryMySQL Maven / Gradle / Ivy
package it.unibz.inf.ontop.dbschema;
/*
* #%L
* ontop-obdalib-core
* %%
* Copyright (C) 2009 - 2014 Free University of Bozen-Bolzano
* %%
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
* #L%
*/
import it.unibz.inf.ontop.dbschema.QuotedID;
import it.unibz.inf.ontop.dbschema.QuotedIDFactory;
import it.unibz.inf.ontop.dbschema.RelationID;
/**
* Creates QuotedIdentifiers following the rules of MySQL:
* - unquoted table identifiers are preserved
* - unquoted column identifiers are not case-sensitive
* - quoted identifiers are preserved
*
* @author Roman Kontchakov
*
*/
public class QuotedIDFactoryMySQL implements QuotedIDFactory {
private final String quotationString;
private final boolean caseSensitiveTableNames;
/**
* used only in DBMetadataExtractor
*/
QuotedIDFactoryMySQL(boolean caseSensitiveTableNames, String quotationString) {
this.quotationString = quotationString;
this.caseSensitiveTableNames = caseSensitiveTableNames;
}
@Override
public QuotedID createAttributeID(String s) {
if (s == null)
return new QuotedID(s, QuotedID.NO_QUOTATION);
if (s.startsWith("\"") && s.endsWith("\""))
return new QuotedID(s.substring(1, s.length() - 1), quotationString, false);
if (s.startsWith("`") && s.endsWith("`"))
return new QuotedID(s.substring(1, s.length() - 1), quotationString, false);
if (s.startsWith("[") && s.endsWith("]"))
return new QuotedID(s.substring(1, s.length() - 1), quotationString, false);
if (s.startsWith("'") && s.endsWith("'"))
return new QuotedID(s.substring(1, s.length() - 1), quotationString, false);
return new QuotedID(s, QuotedID.NO_QUOTATION, false);
}
@Override
public RelationID createRelationID(String schema, String table) {
return new RelationID(createFromString(schema), createFromString(table));
}
public QuotedID createFromString(String s) {
if (s == null)
return new QuotedID(s, QuotedID.NO_QUOTATION);
if (s.startsWith("\"") && s.endsWith("\""))
return new QuotedID(s.substring(1, s.length() - 1), quotationString, caseSensitiveTableNames);
if (s.startsWith("`") && s.endsWith("`"))
return new QuotedID(s.substring(1, s.length() - 1), quotationString, caseSensitiveTableNames);
if (s.startsWith("[") && s.endsWith("]"))
return new QuotedID(s.substring(1, s.length() - 1), quotationString, caseSensitiveTableNames);
if (s.startsWith("'") && s.endsWith("'"))
return new QuotedID(s.substring(1, s.length() - 1), quotationString, caseSensitiveTableNames);
return new QuotedID(s, QuotedID.NO_QUOTATION, caseSensitiveTableNames);
}
@Override
public String getIDQuotationString() {
return quotationString;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy