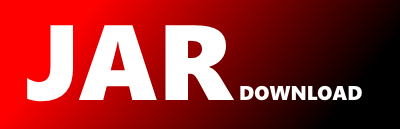
it.unibz.inf.ontop.si.repository.impl.SemanticIndexCache Maven / Gradle / Ivy
package it.unibz.inf.ontop.si.repository.impl;
import it.unibz.inf.ontop.spec.ontology.*;
import it.unibz.inf.ontop.spec.ontology.ClassifiedTBox;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
public class SemanticIndexCache {
private final Map classRanges = new HashMap<>();
private final Map opeRanges = new HashMap<>();
private final Map dpeRanges = new HashMap<>();
private final ClassifiedTBox reasonerDag;
public SemanticIndexCache(ClassifiedTBox reasonerDag) {
this.reasonerDag = reasonerDag;
}
public void buildSemanticIndexFromReasoner() {
//create the indexes
SemanticIndexBuilder engine = new SemanticIndexBuilder(reasonerDag);
/*
* Creating cache of semantic indexes and ranges
*/
for (Entry description : engine.getIndexedClasses()) {
OClass cdesc = (OClass)description.getKey();
classRanges.put(cdesc, description.getValue());
}
for (Entry description : engine.getIndexedObjectProperties()) {
opeRanges.put(description.getKey(), description.getValue());
}
for (Entry description : engine.getIndexedDataProperties()) {
dpeRanges.put(description.getKey(), description.getValue());
}
}
/***
* Returns the intervals (semantic index) for a class or property.
*
* @param name
* @param i
* @return
*/
public SemanticIndexRange getEntry(OClass concept) {
return classRanges.get(concept);
}
public SemanticIndexRange getEntry(ObjectPropertyExpression ope) {
return opeRanges.get(ope);
}
public SemanticIndexRange getEntry(DataPropertyExpression dpe) {
return dpeRanges.get(dpe);
}
public void setIndex(OClass concept, int idx) {
SemanticIndexRange range = new SemanticIndexRange(idx);
classRanges.put(concept, range);
}
public void setIndex(ObjectPropertyExpression ope, Integer idx) {
SemanticIndexRange range = new SemanticIndexRange(idx);
opeRanges.put(ope, range);
}
public void setIndex(DataPropertyExpression dpe, Integer idx) {
SemanticIndexRange range = new SemanticIndexRange(idx);
dpeRanges.put(dpe, range);
}
/*
* these three methods are used only by SI Repository to save the metadata
*/
public Set> getClassIndexEntries() {
return classRanges.entrySet();
}
public Set> getObjectPropertyIndexEntries() {
return opeRanges.entrySet();
}
public Set> getDataPropertyIndexEntries() {
return dpeRanges.entrySet();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy