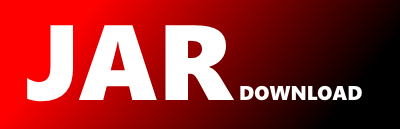
src.it.unimi.di.mg4j.index.AbstractIndexIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mg4j Show documentation
Show all versions of mg4j Show documentation
MG4J (Managing Gigabytes for Java) is a free full-text search engine for large document collections written in Java.
package it.unimi.di.mg4j.index;
/*
* MG4J: Managing Gigabytes for Java
*
* Copyright (C) 2006-2012 Sebastiano Vigna
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 3 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, see .
*
*/
import it.unimi.di.mg4j.search.DocumentIterator;
import it.unimi.di.mg4j.search.visitor.DocumentIteratorVisitor;
import java.io.IOException;
/** A very basic abstract implementation of an index iterator,
* providing an obvious implementation of {@link IndexIterator#term()}, {@link IndexIterator#id()}, {@link DocumentIterator#weight()}
* and of the {@linkplain #accept(DocumentIteratorVisitor) visiting methods}.
*
*/
public abstract class AbstractIndexIterator implements IndexIterator {
/** The term associated with this index iterator. */
protected String term;
/** The identifier associated with this index iterator. */
protected int id;
/** The weight associated with this index iterator. */
protected double weight = 1;
public String term() {
return term;
}
public IndexIterator term( final CharSequence term ) {
this.term = term == null ? null : term.toString();
return this;
}
public int id() {
return id;
}
public IndexIterator id( final int id ) {
this.id = id;
return this;
}
public double weight() {
return weight;
}
public IndexIterator weight( final double weight ) {
this.weight = weight;
return this;
}
public T accept( DocumentIteratorVisitor visitor ) throws IOException {
// TODO: there used to be a visitPost(); check that this works
return visitor.visit( this );
}
public T acceptOnTruePaths( DocumentIteratorVisitor visitor ) throws IOException {
return visitor.visit( this );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy