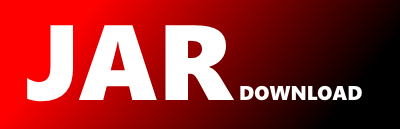
src.it.unimi.dsi.util.package-info Maven / Gradle / Ivy
Show all versions of dsiutils Show documentation
/**
* Miscellaneaous utility classes.
*
*
Pseudorandom number generators
*
* We provide a number of fast, high-quality PRNGs with different features. As a general review:
*
* - {@link it.unimi.dsi.util.SplitMix64Random SplitMix64Random} is the fastest generator, but it has a relatively short period (264) so it should
* not be used to generate very long sequences (the rule of thumb to have a period greater than the square of the length of the sequence you want to generate).
* It is a non-splittable version of Java 8's
SplittableRandom
.
* - {@link it.unimi.dsi.util.XorShift128PlusRandom XorShift128PlusRandom} is a fast-as-light, all-purpose top-quality generator. It is slightly slower than {@link it.unimi.dsi.util.SplitMix64Random SplitMix64Random},
* but its period (2128 − 1) is sufficient for any single-thread application.
*
- {@link it.unimi.dsi.util.XorShift1024StarRandom XorShift1024StarRandom} is fast and provides a long period (21024 − 1) for massive parallel computations.
*
*
* Both {@link it.unimi.dsi.util.XorShift128PlusRandom XorShift128PlusRandom} and {@link it.unimi.dsi.util.XorShift1024StarRandom XorShift1024StarRandom}
* provide {@linkplain it.unimi.dsi.util.XorShift128PlusRandom#jump() jump functions} which make it possible to generate long non-overlapping sequences.
*
*
A table summarizing timings is provided below. Note that we test several different method parameters to show
* the large gap between full 64-bit generators and ThreadLocalRandom
.
*
*
*
* ThreadLocalRandom
* SplittableRandom
* {@link it.unimi.dsi.util.SplitMix64RandomGenerator SplitMix64RandomGenerator}
* {@link it.unimi.dsi.util.XorShift128PlusRandom XorShift128PlusRandom}
* {@link it.unimi.dsi.util.XorShift1024StarRandom XorShift1024StarRandom}
* nextInt() 1.72 1.26 1.17 1.47 2.18
* nextLong() 1.66 1.25 1.19 1.35 2.07
* nextDouble() 2.07 2.07 1.54 1.55 2.21
* nextInt(1000000) 2.85 2.66 2.23 2.83 3.31
* nextInt(2^29+2^28) 7.44 7.04 2.69 3.14 3.60
* nextInt(2^30) 1.80 1.38 1.60 2.39 2.83
* nextInt(2^30+1) 14.98 13.52 2.40 2.96 3.33
* nextInt(2^30+2^29) 7.36 6.86 2.52 3.01 3.52
* nextLong(1000000000000) 2.68 2.62 2.74 2.71 3.70
* nextLong(2^62+1) 14.95 14.09 14.54 12.90 15.35
*
*
* Unfortunately, we have no way to control the optimizations performed
* by the JVM. In C, for example, we use
* gcc
's -fno-move-loop-invariants
and -fno-unroll-loops
options. These
* options are essential to get a sensible result: without them, the
* optimizer can move outside the testing loop constant loads (e.g.,
* multiplicative constants). SplittableRandom
and {@link it.unimi.dsi.util.SplitMix64RandomGenerator SplitMix64RandomGenerator}
* are particularly advantaged in this respect, as they contain three large constants whose
* load can be moved outside the testing loop. The best advice is always that of measuring
* the speed of your application with different generators.
*
*
The quality of all generators we provide is very high: for instance, they perform better than WELL1024a
* or MT19937
(AKA the Mersenne Twister) in the TestU01 BigCrush test suite.
* In particular, {@link it.unimi.dsi.util.SplitMix64Random SplitMix64Random}, {@link it.unimi.dsi.util.XorShift128PlusRandom XorShift128PlusRandom} and {@link it.unimi.dsi.util.XorShift1024StarRandom XorShift1024StarRandom} pass BigCrush.
* More details can be found on the xorshift*
/xorshift+
generators and the PRNG shootout page.
*
*
For each generator, we provide a version that extends {@link java.util.Random}, overriding (as usual) the {@link java.util.Random#next(int) next(int)} method. Nonetheless,
* since the generators are all inherently 64-bit also {@link java.util.Random#nextInt() nextInt()}, {@link java.util.Random#nextFloat() nextFloat()},
* {@link java.util.Random#nextLong() nextLong()}, {@link java.util.Random#nextDouble() nextDouble()}, {@link java.util.Random#nextBoolean() nextBoolean()}
* and {@link java.util.Random#nextBytes(byte[]) nextBytes(byte[])} have been overridden for speed (preserving, of course, {@link java.util.Random}'s semantics).
* In particular, {@link java.util.Random#nextDouble() nextDouble()} and {@link java.util.Random#nextFloat() nextFloat()}
* use a multiplication-free conversion.
*
*
If you do not need an instance of {@link java.util.Random}, or if you need a {@link org.apache.commons.math3.random.RandomGenerator} to use
* with Commons Math, there is for each generator a corresponding {@link org.apache.commons.math3.random.RandomGenerator RandomGenerator}
* implementation, which indeed we suggest to use in general if you do not need a generator implementing {@link java.util.Random}.
*/
package it.unimi.dsi.util;