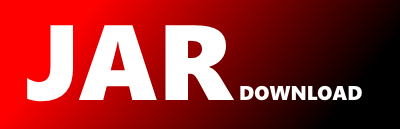
drv.SortedSets.drv Maven / Gradle / Ivy
Show all versions of fastutil Show documentation
/*
* Copyright (C) 2002-2016 Sebastiano Vigna
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package PACKAGE;
import java.util.SortedSet;
import java.util.NoSuchElementException;
#if #keys(reference)
import java.util.Comparator;
#endif
/** A class providing static methods and objects that do useful things with type-specific sorted sets.
*
* @see java.util.Collections
*/
public class SORTED_SETS {
private SORTED_SETS() {}
/** An immutable class representing the empty sorted set and implementing a type-specific set interface.
*
* This class may be useful to implement your own in case you subclass
* a type-specific sorted set.
*/
public static class EmptySet KEY_GENERIC extends SETS.EmptySet KEY_GENERIC implements SORTED_SET KEY_GENERIC, java.io.Serializable, Cloneable {
private static final long serialVersionUID = -7046029254386353129L;
protected EmptySet() {}
public boolean remove( KEY_TYPE ok ) { throw new UnsupportedOperationException(); }
@Deprecated
public KEY_BIDI_ITERATOR KEY_GENERIC KEY_ITERATOR_METHOD() { return iterator(); }
SUPPRESS_WARNINGS_KEY_UNCHECKED
public KEY_BIDI_ITERATOR KEY_GENERIC iterator( KEY_GENERIC_TYPE from ) { return ITERATORS.EMPTY_ITERATOR; }
SUPPRESS_WARNINGS_KEY_UNCHECKED
public SORTED_SET KEY_GENERIC subSet( KEY_GENERIC_TYPE from, KEY_GENERIC_TYPE to ) { return EMPTY_SET; }
SUPPRESS_WARNINGS_KEY_UNCHECKED
public SORTED_SET KEY_GENERIC headSet( KEY_GENERIC_TYPE from ) { return EMPTY_SET; }
SUPPRESS_WARNINGS_KEY_UNCHECKED
public SORTED_SET KEY_GENERIC tailSet( KEY_GENERIC_TYPE to ) { return EMPTY_SET; }
public KEY_GENERIC_TYPE FIRST() { throw new NoSuchElementException(); }
public KEY_GENERIC_TYPE LAST() { throw new NoSuchElementException(); }
public KEY_COMPARATOR KEY_SUPER_GENERIC comparator() { return null; }
#if #keys(primitive)
public SORTED_SET KEY_GENERIC subSet( KEY_GENERIC_CLASS from, KEY_GENERIC_CLASS to ) { return EMPTY_SET; }
public SORTED_SET KEY_GENERIC headSet( KEY_GENERIC_CLASS from ) { return EMPTY_SET; }
public SORTED_SET KEY_GENERIC tailSet( KEY_GENERIC_CLASS to ) { return EMPTY_SET; }
public KEY_GENERIC_CLASS first() { throw new NoSuchElementException(); }
public KEY_GENERIC_CLASS last() { throw new NoSuchElementException(); }
#endif
public Object clone() { return EMPTY_SET; }
private Object readResolve() { return EMPTY_SET; }
}
/** An empty sorted set (immutable). It is serializable and cloneable.
*
*/
SUPPRESS_WARNINGS_KEY_RAWTYPES
public static final EmptySet EMPTY_SET = new EmptySet();
#if #keys(reference)
/** Return an empty sorted set (immutable). It is serializable and cloneable.
*
*
This method provides a typesafe access to {@link #EMPTY_SET}.
* @return an empty sorted set (immutable).
*/
@SuppressWarnings("unchecked")
public static KEY_GENERIC SET KEY_GENERIC emptySet() {
return EMPTY_SET;
}
#endif
/** A class representing a singleton sorted set.
*
*
This class may be useful to implement your own in case you subclass
* a type-specific sorted set.
*/
public static class Singleton KEY_GENERIC extends SETS.Singleton KEY_GENERIC implements SORTED_SET KEY_GENERIC, java.io.Serializable, Cloneable {
private static final long serialVersionUID = -7046029254386353129L;
final KEY_COMPARATOR KEY_SUPER_GENERIC comparator;
private Singleton( final KEY_GENERIC_TYPE element, final KEY_COMPARATOR KEY_SUPER_GENERIC comparator ) {
super( element );
this.comparator = comparator;
}
private Singleton( final KEY_GENERIC_TYPE element ) {
this( element, null );
}
SUPPRESS_WARNINGS_KEY_UNCHECKED
final int compare( final KEY_GENERIC_TYPE k1, final KEY_GENERIC_TYPE k2 ) {
return comparator == null ? KEY_CMP( k1, k2 ) : comparator.compare( k1, k2 );
}
@Deprecated
public KEY_BIDI_ITERATOR KEY_GENERIC KEY_ITERATOR_METHOD() {
return iterator();
}
public KEY_BIDI_ITERATOR KEY_GENERIC iterator( KEY_GENERIC_TYPE from ) {
KEY_BIDI_ITERATOR KEY_GENERIC i = iterator();
if ( compare( element, from ) <= 0 ) i.next();
return i;
}
public KEY_COMPARATOR KEY_SUPER_GENERIC comparator() { return comparator; }
SUPPRESS_WARNINGS_KEY_UNCHECKED
public SORTED_SET KEY_GENERIC subSet( final KEY_GENERIC_TYPE from, final KEY_GENERIC_TYPE to ) { if ( compare( from, element ) <= 0 && compare( element, to ) < 0 ) return this; return EMPTY_SET; }
SUPPRESS_WARNINGS_KEY_UNCHECKED
public SORTED_SET KEY_GENERIC headSet( final KEY_GENERIC_TYPE to ) { if ( compare( element, to ) < 0 ) return this; return EMPTY_SET; }
SUPPRESS_WARNINGS_KEY_UNCHECKED
public SORTED_SET KEY_GENERIC tailSet( final KEY_GENERIC_TYPE from ) { if ( compare( from, element ) <= 0 ) return this; return EMPTY_SET; }
public KEY_GENERIC_TYPE FIRST() { return element; }
public KEY_GENERIC_TYPE LAST() { return element; }
#if #keys(primitive)
/** {@inheritDoc}
* @deprecated Please use the corresponding type-specific method instead. */
@Deprecated
public KEY_CLASS first() { return KEY2OBJ( element ); }
/** {@inheritDoc}
* @deprecated Please use the corresponding type-specific method instead. */
@Deprecated
public KEY_CLASS last() { return KEY2OBJ( element ); }
/** {@inheritDoc}
* @deprecated Please use the corresponding type-specific method instead. */
@Deprecated
public SORTED_SET KEY_GENERIC subSet( final KEY_CLASS from, final KEY_CLASS to ) { return subSet( KEY_CLASS2TYPE( from ), KEY_CLASS2TYPE( to ) ); }
/** {@inheritDoc}
* @deprecated Please use the corresponding type-specific method instead. */
@Deprecated
public SORTED_SET KEY_GENERIC headSet( final KEY_CLASS to ) { return headSet( KEY_CLASS2TYPE( to ) ); }
/** {@inheritDoc}
* @deprecated Please use the corresponding type-specific method instead. */
@Deprecated
public SORTED_SET KEY_GENERIC tailSet( final KEY_CLASS from ) { return tailSet( KEY_CLASS2TYPE( from ) ); }
#endif
}
/** Returns a type-specific immutable sorted set containing only the specified element. The returned sorted set is serializable and cloneable.
*
* @param element the only element of the returned sorted set.
* @return a type-specific immutable sorted set containing just element
.
*/
public static KEY_GENERIC SORTED_SET KEY_GENERIC singleton( final KEY_GENERIC_TYPE element ) {
return new Singleton KEY_GENERIC( element );
}
/** Returns a type-specific immutable sorted set containing only the specified element, and using a specified comparator. The returned sorted set is serializable and cloneable.
*
* @param element the only element of the returned sorted set.
* @param comparator the comparator to use in the returned sorted set.
* @return a type-specific immutable sorted set containing just element
.
*/
public static KEY_GENERIC SORTED_SET KEY_GENERIC singleton( final KEY_GENERIC_TYPE element, final KEY_COMPARATOR KEY_SUPER_GENERIC comparator ) {
return new Singleton KEY_GENERIC( element, comparator );
}
#if #keys(primitive)
/** Returns a type-specific immutable sorted set containing only the specified element. The returned sorted set is serializable and cloneable.
*
* @param element the only element of the returned sorted set.
* @return a type-specific immutable sorted set containing just element
.
*/
public static KEY_GENERIC SORTED_SET KEY_GENERIC singleton( final Object element ) {
return new Singleton( KEY_OBJ2TYPE( element ) );
}
/** Returns a type-specific immutable sorted set containing only the specified element, and using a specified comparator. The returned sorted set is serializable and cloneable.
*
* @param element the only element of the returned sorted set.
* @param comparator the comparator to use in the returned sorted set.
* @return a type-specific immutable sorted set containing just element
.
*/
public static KEY_GENERIC SORTED_SET KEY_GENERIC singleton( final Object element, final KEY_COMPARATOR KEY_SUPER_GENERIC comparator ) {
return new Singleton( KEY_OBJ2TYPE( element ), comparator );
}
#endif
/** A synchronized wrapper class for sorted sets. */
public static class SynchronizedSortedSet KEY_GENERIC extends SETS.SynchronizedSet KEY_GENERIC implements SORTED_SET KEY_GENERIC, java.io.Serializable {
private static final long serialVersionUID = -7046029254386353129L;
protected final SORTED_SET KEY_GENERIC sortedSet;
protected SynchronizedSortedSet( final SORTED_SET KEY_GENERIC s, final Object sync ) {
super( s, sync );
sortedSet = s;
}
protected SynchronizedSortedSet( final SORTED_SET KEY_GENERIC s ) {
super( s );
sortedSet = s;
}
public KEY_COMPARATOR KEY_SUPER_GENERIC comparator() { synchronized( sync ) { return sortedSet.comparator(); } }
public SORTED_SET KEY_GENERIC subSet( final KEY_GENERIC_TYPE from, final KEY_GENERIC_TYPE to ) { return new SynchronizedSortedSet KEY_GENERIC( sortedSet.subSet( from, to ), sync ); }
public SORTED_SET KEY_GENERIC headSet( final KEY_GENERIC_TYPE to ) { return new SynchronizedSortedSet KEY_GENERIC( sortedSet.headSet( to ), sync ); }
public SORTED_SET KEY_GENERIC tailSet( final KEY_GENERIC_TYPE from ) { return new SynchronizedSortedSet KEY_GENERIC( sortedSet.tailSet( from ), sync ); }
public KEY_BIDI_ITERATOR KEY_GENERIC iterator() { return sortedSet.iterator(); }
public KEY_BIDI_ITERATOR KEY_GENERIC iterator( final KEY_GENERIC_TYPE from ) { return sortedSet.iterator( from ); }
@Deprecated
public KEY_BIDI_ITERATOR KEY_GENERIC KEY_ITERATOR_METHOD() { return sortedSet.iterator(); }
public KEY_GENERIC_TYPE FIRST() { synchronized( sync ) { return sortedSet.FIRST(); } }
public KEY_GENERIC_TYPE LAST() { synchronized( sync ) { return sortedSet.LAST(); } }
#if #keys(primitive)
public KEY_CLASS first() { synchronized( sync ) { return sortedSet.first(); } }
public KEY_CLASS last() { synchronized( sync ) { return sortedSet.last(); } }
public SORTED_SET KEY_GENERIC subSet( final KEY_CLASS from, final KEY_CLASS to ) { return new SynchronizedSortedSet( sortedSet.subSet( from, to ), sync ); }
public SORTED_SET KEY_GENERIC headSet( final KEY_CLASS to ) { return new SynchronizedSortedSet( sortedSet.headSet( to ), sync ); }
public SORTED_SET KEY_GENERIC tailSet( final KEY_CLASS from ) { return new SynchronizedSortedSet( sortedSet.tailSet( from ), sync ); }
#endif
}
/** Returns a synchronized type-specific sorted set backed by the given type-specific sorted set.
*
* @param s the sorted set to be wrapped in a synchronized sorted set.
* @return a synchronized view of the specified sorted set.
* @see java.util.Collections#synchronizedSortedSet(SortedSet)
*/
public static KEY_GENERIC SORTED_SET KEY_GENERIC synchronize( final SORTED_SET KEY_GENERIC s ) { return new SynchronizedSortedSet KEY_GENERIC( s ); }
/** Returns a synchronized type-specific sorted set backed by the given type-specific sorted set, using an assigned object to synchronize.
*
* @param s the sorted set to be wrapped in a synchronized sorted set.
* @param sync an object that will be used to synchronize the access to the sorted set.
* @return a synchronized view of the specified sorted set.
* @see java.util.Collections#synchronizedSortedSet(SortedSet)
*/
public static KEY_GENERIC SORTED_SET KEY_GENERIC synchronize( final SORTED_SET KEY_GENERIC s, final Object sync ) { return new SynchronizedSortedSet KEY_GENERIC( s, sync ); }
/** An unmodifiable wrapper class for sorted sets. */
public static class UnmodifiableSortedSet KEY_GENERIC extends SETS.UnmodifiableSet KEY_GENERIC implements SORTED_SET KEY_GENERIC, java.io.Serializable {
private static final long serialVersionUID = -7046029254386353129L;
protected final SORTED_SET KEY_GENERIC sortedSet;
protected UnmodifiableSortedSet( final SORTED_SET KEY_GENERIC s ) {
super( s );
sortedSet = s;
}
public KEY_COMPARATOR KEY_SUPER_GENERIC comparator() { return sortedSet.comparator(); }
public SORTED_SET KEY_GENERIC subSet( final KEY_GENERIC_TYPE from, final KEY_GENERIC_TYPE to ) { return new UnmodifiableSortedSet KEY_GENERIC( sortedSet.subSet( from, to ) ); }
public SORTED_SET KEY_GENERIC headSet( final KEY_GENERIC_TYPE to ) { return new UnmodifiableSortedSet KEY_GENERIC( sortedSet.headSet( to ) ); }
public SORTED_SET KEY_GENERIC tailSet( final KEY_GENERIC_TYPE from ) { return new UnmodifiableSortedSet KEY_GENERIC( sortedSet.tailSet( from ) ); }
public KEY_BIDI_ITERATOR KEY_GENERIC iterator() { return ITERATORS.unmodifiable( sortedSet.iterator() ); }
public KEY_BIDI_ITERATOR KEY_GENERIC iterator( final KEY_GENERIC_TYPE from ) { return ITERATORS.unmodifiable( sortedSet.iterator( from ) ); }
@Deprecated
public KEY_BIDI_ITERATOR KEY_GENERIC KEY_ITERATOR_METHOD() { return iterator(); }
public KEY_GENERIC_TYPE FIRST() { return sortedSet.FIRST(); }
public KEY_GENERIC_TYPE LAST() { return sortedSet.LAST(); }
#if #keys(primitive)
public KEY_CLASS first() { return sortedSet.first(); }
public KEY_CLASS last() { return sortedSet.last(); }
public SORTED_SET KEY_GENERIC subSet( final KEY_GENERIC_CLASS from, final KEY_GENERIC_CLASS to ) { return new UnmodifiableSortedSet( sortedSet.subSet( from, to ) ); }
public SORTED_SET KEY_GENERIC headSet( final KEY_GENERIC_CLASS to ) { return new UnmodifiableSortedSet( sortedSet.headSet( to ) ); }
public SORTED_SET KEY_GENERIC tailSet( final KEY_GENERIC_CLASS from ) { return new UnmodifiableSortedSet( sortedSet.tailSet( from ) ); }
#endif
}
/** Returns an unmodifiable type-specific sorted set backed by the given type-specific sorted set.
*
* @param s the sorted set to be wrapped in an unmodifiable sorted set.
* @return an unmodifiable view of the specified sorted set.
* @see java.util.Collections#unmodifiableSortedSet(SortedSet)
*/
public static KEY_GENERIC SORTED_SET KEY_GENERIC unmodifiable( final SORTED_SET KEY_GENERIC s ) { return new UnmodifiableSortedSet KEY_GENERIC( s ); }
#if defined(TEST) && ! #keyclass( Reference )
private static KEY_TYPE genKey() {
#if #keyclass(Byte) || #keyclass(Short) || #keyclass(Character)
return (KEY_TYPE)(r.nextInt());
#elif #keys(primitive)
return r.NEXT_KEY();
#elif #keyclass(Object)
return Integer.toBinaryString( r.nextInt() );
#endif
}
protected static void testSets( KEY_TYPE k, SORTED_SET m, SortedSet t, int level ) {
int n = 100;
int c;
long ms;
boolean mThrowsIllegal, tThrowsIllegal, mThrowsNoElement, tThrowsNoElement, mThrowsIndex, tThrowsIndex, mThrowsUnsupp, tThrowsUnsupp;
boolean rt = false, rm = false;
if ( level == 0 ) return;
/* Now we check that m and t are equal. */
if ( !m.equals( t ) || ! t.equals( m ) ) System.err.println("m: " + m + " t: " + t);
ensure( m.equals( t ), "Error (" + level + ", " + seed + "): ! m.equals( t ) at start" );
ensure( t.equals( m ), "Error (" + level + ", " + seed + "): ! t.equals( m ) at start" );
/* Now we check that m actually holds that data. */
for(java.util.Iterator i=t.iterator(); i.hasNext(); ) {
ensure( m.contains( i.next() ), "Error (" + level + ", " + seed + "): m and t differ on an entry after insertion (iterating on t)" );
}
/* Now we check that m actually holds that data, but iterating on m. */
for(java.util.Iterator i=m.iterator(); i.hasNext(); ) {
ensure( t.contains( i.next() ), "Error (" + level + ", " + seed + "): m and t differ on an entry after insertion (iterating on m)" );
}
/* Now we check that inquiries about random data give the same answer in m and t. For
m we use the polymorphic method. */
for(int i=0; i 1 ) r = new java.util.Random( seed = Long.parseLong( arg[ 1 ] ) );
try {
test();
} catch( Throwable e ) {
e.printStackTrace( System.err );
System.err.println( "seed: " + seed );
}
}
#endif
}