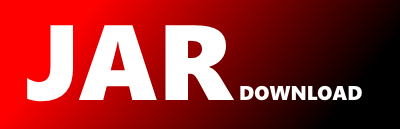
src.it.unimi.dsi.big.mg4j.index.cluster.ContiguousDocumentalStrategy Maven / Gradle / Ivy
Show all versions of mg4j-big Show documentation
package it.unimi.dsi.big.mg4j.index.cluster;
/*
* MG4J: Managing Gigabytes for Java (big)
*
* Copyright (C) 2006-2011 Sebastiano Vigna
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 3 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, see .
*
*/
import it.unimi.dsi.util.Properties;
import java.io.Serializable;
import java.util.Arrays;
/** A documental partitioning and clustering strategy that partitions an index into contiguous segments.
*
* To partition an index in contiguous parts, you must provide an array
* of cutpoints, which define each part. More precisely, given
* cutpoints c0,c2,…,ck,
* the global index will be partitioned into k local indices containing the documents
* from c0 (included) to c1 (excluded), from
* c1 (included) to c2 and so on. Note that
* necessarily c0=0 and ck=N,
* where N is the number of globally indexed documents.
*
*
The {@link #properties()} method provides two properties, pointerfrom and pointerto,
* that contain the first (included) and last (excluded) pointer in the local index.
*
* @author Alessandro Arrabito
* @author Sebastiano Vigna
*/
public class ContiguousDocumentalStrategy implements DocumentalPartitioningStrategy, DocumentalClusteringStrategy,Serializable{
private static final long serialVersionUID = 0L;
/** The cutpoints.*/
private final long[] cutPoint;
/** The (cached) number of segments. */
private final int k;
/** Creates a new contiguous strategy with the given cutpoints.
*
*
Note that {@link DocumentalStrategies} has ready-made factory
* methods for the common cases.
*
* @param cutPoint an array of cutpoints (see the class description}.
*/
public ContiguousDocumentalStrategy( final long... cutPoint ) {
if ( cutPoint.length == 0 ) throw new IllegalArgumentException( "Empty cutpoint array" );
if ( cutPoint[ 0 ] != 0 ) throw new IllegalArgumentException( "The first cutpoint must be 0" );
this.cutPoint = cutPoint;
this.k = cutPoint.length - 1;
}
public int numberOfLocalIndices() {
return k;
}
public int localIndex( final long globalPointer ) {
if ( globalPointer >= cutPoint[ k ] ) throw new IndexOutOfBoundsException( Long.toString( globalPointer ) );
for ( int i = k; i-- != 0; ) if ( globalPointer >= cutPoint[ i ] ) return i;
throw new IndexOutOfBoundsException( Long.toString( globalPointer ) );
}
public long localPointer( final long globalPointer ) {
return globalPointer - cutPoint[ localIndex( globalPointer ) ];
}
public long globalPointer( final int localIndex, final long localPointer ) {
return localPointer + cutPoint[ localIndex ];
}
public long numberOfDocuments( final int localIndex ) {
return cutPoint[ localIndex + 1 ] - cutPoint[ localIndex ];
}
public Properties[] properties() {
Properties[] properties = new Properties[ k ];
for( int i = 0; i < k; i++ ) {
properties[ i ] = new Properties();
properties[ i ].addProperty( "pointerfrom", cutPoint[ i ] );
properties[ i ].addProperty( "pointerto", cutPoint[ i + 1 ] );
}
return properties;
}
public String toString() {
return Arrays.toString( cutPoint );
}
}