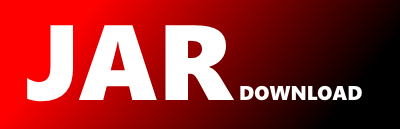
src.it.unimi.dsi.big.mg4j.index.remote.RemoteBitStreamHPIndex Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mg4j-big Show documentation
Show all versions of mg4j-big Show documentation
MG4J (Managing Gigabytes for Java) is a free full-text search engine for large document collections written in Java. The big version is a fork of the original MG4J that can handle more than 2^31 terms and documents.
The newest version!
package it.unimi.dsi.big.mg4j.index.remote;
/*
* MG4J: Managing Gigabytes for Java
*
* Copyright (C) 2006-2011 Sebastiano Vigna
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 3 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, see .
*
*/
import it.unimi.dsi.big.mg4j.index.BitStreamHPIndex;
import it.unimi.dsi.big.mg4j.index.CompressionFlags;
import it.unimi.dsi.big.mg4j.index.TermProcessor;
import it.unimi.dsi.big.mg4j.index.payload.Payload;
import it.unimi.dsi.big.util.PrefixMap;
import it.unimi.dsi.big.util.StringMap;
import it.unimi.dsi.fastutil.ints.IntBigList;
import it.unimi.dsi.fastutil.longs.LongBigList;
import it.unimi.dsi.io.InputBitStream;
import it.unimi.dsi.util.Properties;
import java.io.IOException;
import java.io.InputStream;
import java.net.SocketAddress;
public class RemoteBitStreamHPIndex extends BitStreamHPIndex {
static final long serialVersionUID = 0L;
// TODO: find a way to use index-specific default buffer sizes
/** The default buffer size. */
@SuppressWarnings("hiding")
public final static int DEFAULT_BUFFER_SIZE = 512;
/** The address of the socket associated with this index. */
public final SocketAddress address;
public RemoteBitStreamHPIndex( final SocketAddress address, long numberOfDocuments, long numberOfTerms, final long numberOfPostings, final long numberOfOccurrences, int maxCount,
Payload payload, CompressionFlags.Coding frequencyCoding, CompressionFlags.Coding pointerCoding, CompressionFlags.Coding countCoding, CompressionFlags.Coding positionCoding, int quantum, int height, int bufferSize, TermProcessor termProcessor, String field, Properties properties, StringMap extends CharSequence> termMap, PrefixMap extends CharSequence> prefixMap,
IntBigList sizes, LongBigList offsets ) {
super( numberOfDocuments, numberOfTerms, numberOfPostings, numberOfOccurrences, maxCount, payload, frequencyCoding, pointerCoding, countCoding, positionCoding, quantum, height, bufferSize, termProcessor, field, properties,
termMap, prefixMap, sizes, offsets );
this.address = address;
}
@Override
public InputBitStream getPositionsInputBitStream(int bufferSize) throws IOException {
return new InputBitStream( new RemoteInputStream( address, IndexServer.GET_CLIENT_POS_INPUT_STREAM ), bufferSize );
}
@Override
public InputStream getPositionsInputStream() throws IOException {
return new RemoteInputStream( address, IndexServer.GET_CLIENT_POS_INPUT_STREAM );
}
@Override
public InputBitStream getInputBitStream(int bufferSize) throws IOException {
return new InputBitStream( new RemoteInputStream( address, IndexServer.GET_CLIENT_INPUT_STREAM ), bufferSize );
}
@Override
public InputStream getInputStream() throws IOException {
return new RemoteInputStream( address, IndexServer.GET_CLIENT_INPUT_STREAM );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy