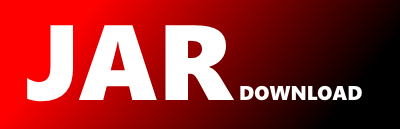
src.it.unimi.dsi.big.mg4j.index.remote.RemoteIndex Maven / Gradle / Ivy
Show all versions of mg4j-big Show documentation
package it.unimi.dsi.big.mg4j.index.remote;
/*
* MG4J: Managing Gigabytes for Java (big)
*
* Copyright (C) 2006-2011 Sebastiano Vigna
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 3 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, see .
*
*/
import it.unimi.dsi.fastutil.ints.IntBigList;
import it.unimi.dsi.big.mg4j.index.Index;
import it.unimi.dsi.big.mg4j.index.IndexIterator;
import it.unimi.dsi.big.mg4j.index.IndexReader;
import it.unimi.dsi.big.mg4j.index.TermProcessor;
import it.unimi.dsi.big.mg4j.index.payload.Payload;
import it.unimi.dsi.util.Properties;
import java.io.IOException;
import java.net.SocketAddress;
/** A remote index.
*
* Remote indices (as opposed to {@linkplain RemoteBitStreamIndex remote bitstream indices})
* provide access to document iterators only. For composite indices this is the only possible access, as there is
* not underlying bit stream to export.
*
*
When a new {@link it.unimi.dsi.big.mg4j.index.IndexReader} is created, this index
* connects to the server and will start a new server thread that will interact with the returned
* {@link it.unimi.dsi.big.mg4j.index.remote.RemoteIndexReader}.
*
* @author Sebastiano Vigna
* @since 1.1
*/
public class RemoteIndex extends Index {
private static final long serialVersionUID = 0L;
/** The default buffer size. */
public final static int DEFAULT_BUFFER_SIZE = 1024;
/** The socket exporting the index. */
public final SocketAddress socketAddress;
public RemoteIndex( final SocketAddress socketAddress, final long numberOfDocuments, final long numberOfTerms, final long numberOfPostings, final long numberOfOccurrences, final int maxCount,
final Payload payload, final boolean hasCounts, final boolean hasPositions, final TermProcessor termProcessor,
final String field, final IntBigList sizes, final Properties properties ) {
super( numberOfDocuments, numberOfTerms, numberOfPostings, numberOfOccurrences, maxCount, payload, hasCounts, hasPositions, termProcessor, field, sizes, properties );
this.socketAddress = socketAddress;
}
public IndexReader getReader( final int bufferSize ) throws IOException {
return new RemoteIndexReader( this, bufferSize == -1 ? DEFAULT_BUFFER_SIZE : bufferSize );
}
public IndexIterator documents( final CharSequence prefix, final int limit ) {
// TODO: this must be remoted.
throw new UnsupportedOperationException();
}
}