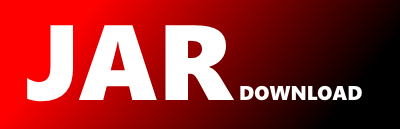
test.it.unimi.dsi.big.mg4j.util.TermMapTest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mg4j-big Show documentation
Show all versions of mg4j-big Show documentation
MG4J (Managing Gigabytes for Java) is a free full-text search engine for large document collections written in Java. The big version is a fork of the original MG4J that can handle more than 2^31 terms and documents.
The newest version!
package it.unimi.dsi.big.mg4j.util;
import junit.framework.TestCase;
import it.unimi.dsi.fastutil.objects.Object2ObjectOpenHashMap;
import it.unimi.dsi.fastutil.objects.ObjectOpenHashSet;
import it.unimi.dsi.lang.MutableString;
import it.unimi.dsi.big.mg4j.io.ByteArrayPostingList;
import it.unimi.dsi.big.mg4j.tool.Scan.Completeness;
public class TermMapTest extends TestCase {
public void testInsertion() {
TermMap termMap = new TermMap();
ByteArrayPostingList byteArrayPostingList = new ByteArrayPostingList( new byte[ 0 ], true, Completeness.COUNTS );
termMap.put( new MutableString( "foo" ), byteArrayPostingList );
assertSame( byteArrayPostingList, termMap.get( new MutableString( "foo" ) ) );
assertEquals( new ObjectOpenHashSet( new ByteArrayPostingList[] { byteArrayPostingList } ),
termMap.values() );
assertEquals( new ObjectOpenHashSet( new MutableString[] { new MutableString( "foo" ) } ),
termMap.keySet() );
}
public void testTwoInsertions() {
TermMap termMap = new TermMap();
ByteArrayPostingList byteArrayPostingList0 = new ByteArrayPostingList( new byte[ 0 ], true, Completeness.COUNTS );
ByteArrayPostingList byteArrayPostingList1 = new ByteArrayPostingList( new byte[ 0 ], true, Completeness.COUNTS );
termMap.put( new MutableString( "foo" ), byteArrayPostingList0 );
termMap.put( new MutableString( "bar" ), byteArrayPostingList1 );
assertSame( byteArrayPostingList0, termMap.get( new MutableString( "foo" ) ) );
assertSame( byteArrayPostingList1, termMap.get( new MutableString( "bar" ) ) );
assertEquals( new ObjectOpenHashSet( new ByteArrayPostingList[] { byteArrayPostingList0, byteArrayPostingList1 } ),
termMap.values() );
assertEquals( new ObjectOpenHashSet( new MutableString[] { new MutableString( "foo" ), new MutableString( "bar" ) } ),
termMap.keySet() );
}
public void testManyInsertions() {
TermMap termMap = new TermMap();
Object2ObjectOpenHashMap map = new Object2ObjectOpenHashMap();
for( int i = 0; i < 10000; i++ ) {
final MutableString mutableString = new MutableString( Integer.toString( i ) );
final ByteArrayPostingList byteArrayPostingList = new ByteArrayPostingList( new byte[ 0 ], true, Completeness.COUNTS );
termMap.put( mutableString, byteArrayPostingList );
map.put( mutableString, byteArrayPostingList );
}
assertEquals( map, termMap );
assertEquals( map.keySet(), termMap.keySet() );
assertEquals( new ObjectOpenHashSet( map.values() ), new ObjectOpenHashSet( termMap.values() ) );
assertEquals( 10000, termMap.size() );
assertEquals( 10000, termMap.keySet().size() );
assertEquals( 10000, termMap.values().size() );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy