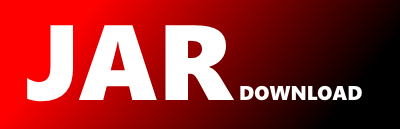
it.uniroma2.art.coda.structures.CODATriple Maven / Gradle / Ivy
package it.uniroma2.art.coda.structures;
import java.util.List;
import it.uniroma2.art.coda.exception.ValueNotPresentDueToConfigurationException;
import org.eclipse.rdf4j.model.IRI;
import org.eclipse.rdf4j.model.Resource;
import org.eclipse.rdf4j.model.Value;
import it.uniroma2.art.coda.pearl.model.annotation.Annotation;
/**
* This class contains a triple of ARTResources representing a RDF Triple
*
* @author Andrea Turbati
*
*/
public class CODATriple {
private Resource subject;
private IRI predicate;
private Value object;
private double confidence;
private List listAnnotations;
private String subjectNameInGraph;
private String predicateNameInGraph;
private String objectNameInGraph;
/**
* @param subject
* the subject of the triple
* @param predicate
* the predicate of the triple
* @param object
* the object of the triple
* @param confidence
* the confidence value for this triple
*/
public CODATriple(Resource subject, IRI predicate, Value object, double confidence) {
this.subject = subject;
this.predicate = predicate;
this.object = object;
this.confidence = confidence;
this.subjectNameInGraph = null;
this.predicateNameInGraph = null;
this.objectNameInGraph = null;
}
public CODATriple(Resource subject, IRI predicate, Value object, double confidence, String subjectNameInGraph,
String predicateNameInGraph, String objectNameInGraph) {
this.subject = subject;
this.predicate = predicate;
this.object = object;
this.confidence = confidence;
this.subjectNameInGraph = subjectNameInGraph;
this.predicateNameInGraph = predicateNameInGraph;
this.objectNameInGraph = objectNameInGraph;
}
/**
* Constructor to set the annotations of the ARTTriple.
* @param subject
* @param predicate
* @param object
* @param listAnnotations
* @param confidence
*/
public CODATriple(Resource subject, IRI predicate, Value object, List listAnnotations, double confidence) {
this.subject = subject;
this.predicate = predicate;
this.object = object;
this.confidence = confidence;
this.listAnnotations = listAnnotations;
this.subjectNameInGraph = null;
this.predicateNameInGraph = null;
this.objectNameInGraph = null;
}
public CODATriple(Resource subject, IRI predicate, Value object, List listAnnotations, double confidence,
String subjectNameInGraph, String predicateNameInGraph, String objectNameInGraph) {
this.subject = subject;
this.predicate = predicate;
this.object = object;
this.confidence = confidence;
this.listAnnotations = listAnnotations;
this.subjectNameInGraph = subjectNameInGraph;
this.predicateNameInGraph = predicateNameInGraph;
this.objectNameInGraph = objectNameInGraph;
}
/**
* Get the subject of the triple
*
* @return the subject of the triple
*/
public Resource getSubject() {
return subject;
}
/**
* Get the predicate of the triple
*
* @return the predicate of the triple
*/
public IRI getPredicate() {
return predicate;
}
/**
* Get the object of the triple.
*
* @return the object of the triple
*/
public Value getObject() {
return object;
}
/**
* Get the confidence value
*
* @return the confidence value
*/
public double getConfidence() {
return confidence;
}
/**
* @return the list of annotations
* @author Gambella Riccardo
*/
public List getListAnnotations(){
return listAnnotations;
}
/**
* return the annotation having the given name or null if no annotation has the given name
* @param annotationName
* @return the annotation having the given name or null if no annotation has the given name
*/
public Annotation getAnnotation(String annotationName) {
for(Annotation annotation : listAnnotations){
if(annotation.getName().equals(annotationName)){
return annotation;
}
}
return null;
}
/**
*
* @return a boolean indicating if there are annotations
* @author Gambella Riccardo
*/
public boolean hasListAnnotations(){
if(listAnnotations == null)
return false;
if(listAnnotations.isEmpty())
return false;
return true;
}
public String getSubjectNameInGraph() throws ValueNotPresentDueToConfigurationException {
if (subjectNameInGraph == null) {
throw new ValueNotPresentDueToConfigurationException("subjectNameInGraph is not present since" +
"the parameter addNodeAssignmentMap was false or not passed");
}
return subjectNameInGraph;
}
public String getPredicateNameInGraph() throws ValueNotPresentDueToConfigurationException {
if (predicateNameInGraph == null) {
throw new ValueNotPresentDueToConfigurationException("predicateNameInGraph is not present since" +
"the parameter addNodeAssignmentMap was false or not passed");
}
return predicateNameInGraph;
}
public String getObjectNameInGraph() throws ValueNotPresentDueToConfigurationException {
if (objectNameInGraph == null) {
throw new ValueNotPresentDueToConfigurationException("objectNameInGraph is not present since" +
"the parameter addNodeAssignmentMap was false or not passed");
}
return objectNameInGraph;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy