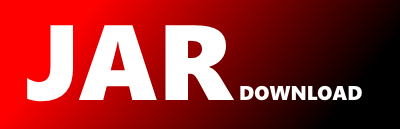
it.uniroma2.art.lime.profiler.ConceptualizationSetStatistics Maven / Gradle / Ivy
The newest version!
package it.uniroma2.art.lime.profiler;
import java.math.BigDecimal;
import java.math.BigInteger;
import org.eclipse.rdf4j.model.IRI;
import org.eclipse.rdf4j.model.Model;
import org.eclipse.rdf4j.model.Resource;
import org.eclipse.rdf4j.model.ValueFactory;
import org.eclipse.rdf4j.model.impl.SimpleValueFactory;
import org.eclipse.rdf4j.model.util.Literals;
import org.eclipse.rdf4j.model.util.Models;
import org.eclipse.rdf4j.model.vocabulary.RDF;
import it.uniroma2.art.lime.model.vocabulary.LIME;
public class ConceptualizationSetStatistics {
private Resource lexiconDataset;
private Resource conceptualDataset;
private BigInteger conceptualizations;
private BigInteger concepts;
private BigInteger lexicalEntries;
private BigDecimal avgSynonymy;
private BigDecimal avgAmbiguity;
public Resource getLexiconDataset() {
return lexiconDataset;
}
public void setLexiconDataset(Resource lexiconDataset) {
this.lexiconDataset = lexiconDataset;
}
public Resource getConceptualDataset() {
return conceptualDataset;
}
public void setConceptualDataset(Resource conceptualDataset) {
this.conceptualDataset = conceptualDataset;
}
public BigInteger getConceptualizations() {
return conceptualizations;
}
public void setConceptualizations(BigInteger conceptualizations) {
this.conceptualizations = conceptualizations;
}
public BigInteger getConcepts() {
return concepts;
}
public void setConcepts(BigInteger concepts) {
this.concepts = concepts;
}
public BigInteger getLexicalEntries() {
return lexicalEntries;
}
public void setLexicalEntries(BigInteger lexicalEntries) {
this.lexicalEntries = lexicalEntries;
}
public BigDecimal getAvgAmbiguity() {
return avgAmbiguity;
}
public void setAvgAmbiguity(BigDecimal avgAmbiguity) {
this.avgAmbiguity = avgAmbiguity;
}
public BigDecimal getAvgSynonymy() {
return avgSynonymy;
}
public void setAvgSynonymy(BigDecimal avgSynonymy) {
this.avgSynonymy = avgSynonymy;
}
public void serialize(Model graph, Resource dataset) {
ValueFactory vf = SimpleValueFactory.getInstance();
graph.add(dataset, RDF.TYPE, LIME.CONCEPTUALIZATION_SET);
if (lexiconDataset != null) {
graph.add(dataset, LIME.LEXICON_DATASET, lexiconDataset);
}
if (conceptualDataset != null) {
graph.add(dataset, LIME.CONCEPTUAL_DATASET, conceptualDataset);
}
if (conceptualizations != null) {
graph.add(dataset, LIME.CONCEPTUALIZATIONS, vf.createLiteral(conceptualizations));
}
if (concepts != null) {
graph.add(dataset, LIME.CONCEPTS, vf.createLiteral(concepts));
}
if (lexicalEntries != null) {
graph.add(dataset, LIME.LEXICAL_ENTRIES, vf.createLiteral(lexicalEntries));
}
if (avgAmbiguity != null) {
graph.add(dataset, LIME.AVG_AMBIGUITY, vf.createLiteral(avgAmbiguity));
}
if (avgSynonymy != null) {
graph.add(dataset, LIME.AVG_SYNONYMY, vf.createLiteral(avgSynonymy));
}
}
public void parse(Model model, IRI conceptualizationSet) throws NullPointerException {
this.setLexiconDataset(Models.getPropertyResource(model, conceptualizationSet, LIME.LEXICON_DATASET)
.orElseThrow(() -> new NullPointerException()));
this.setConceptualDataset(Models.getPropertyResource(model, conceptualizationSet, LIME.CONCEPTUAL_DATASET)
.orElseThrow(() -> new NullPointerException()));
this.setConceptualizations(Models.getPropertyLiteral(model, conceptualizationSet, LIME.CONCEPTUALIZATIONS)
.map(l -> Literals.getIntegerValue(l, BigInteger.ZERO))
.orElseThrow(() -> new NullPointerException()));
this.setLexicalEntries(Models.getPropertyLiteral(model, conceptualizationSet, LIME.LEXICAL_ENTRIES)
.map(l -> Literals.getIntegerValue(l, BigInteger.ZERO))
.orElseThrow(() -> new NullPointerException()));
this.setConcepts(Models.getPropertyLiteral(model, conceptualizationSet, LIME.CONCEPTS)
.map(l -> Literals.getIntegerValue(l, BigInteger.ZERO))
.orElseThrow(() -> new NullPointerException()));
this.setAvgAmbiguity(Models.getPropertyLiteral(model, conceptualizationSet, LIME.AVG_AMBIGUITY)
.map(l -> Literals.getDecimalValue(l, BigDecimal.ZERO))
.orElseThrow(() -> new NullPointerException()));
this.setAvgSynonymy(Models.getPropertyLiteral(model, conceptualizationSet, LIME.AVG_SYNONYMY)
.map(l -> Literals.getDecimalValue(l, BigDecimal.ZERO))
.orElseThrow(() -> new NullPointerException()));
}
public static ConceptualizationSetStatistics of(Resource lexiconDataset, Resource conceptualDataset,
BigInteger conceptualizations, BigInteger concepts, BigInteger lexicalEntries,
BigDecimal avgSynonymy, BigDecimal avgAmbiguity) {
ConceptualizationSetStatistics stats = new ConceptualizationSetStatistics();
stats.setLexiconDataset(lexiconDataset);
stats.setConceptualDataset(conceptualDataset);
stats.setConceptualizations(conceptualizations);
stats.setConcepts(concepts);
stats.setLexicalEntries(lexicalEntries);
stats.setAvgSynonymy(avgSynonymy);
stats.setAvgAmbiguity(avgAmbiguity);
return stats;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy