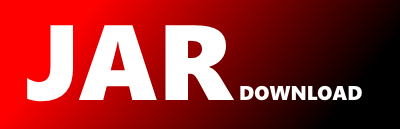
it.uniroma2.art.lime.profiler.impl.ResourceLocationUtilsInternal Maven / Gradle / Ivy
The newest version!
package it.uniroma2.art.lime.profiler.impl;
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
import it.uniroma2.art.lime.profiler.utils.ResourceLocationUtils;
import org.eclipse.rdf4j.common.iteration.Iterations;
import org.eclipse.rdf4j.model.IRI;
import org.eclipse.rdf4j.model.Resource;
import org.eclipse.rdf4j.model.Statement;
import org.eclipse.rdf4j.model.impl.SimpleValueFactory;
import org.eclipse.rdf4j.model.vocabulary.VOID;
import org.eclipse.rdf4j.query.QueryResults;
import org.eclipse.rdf4j.queryrender.RenderUtils;
import org.eclipse.rdf4j.repository.RepositoryResult;
import org.eclipse.rdf4j.rio.ntriples.NTriplesUtil;
import it.uniroma2.art.lime.model.repo.LIMERepositoryConnectionWrapper;
import it.uniroma2.art.lime.profiler.ProfilerContext;
import it.uniroma2.art.lime.profiler.ProfilerOptions;
public abstract class ResourceLocationUtilsInternal {
public static Map retrieveDatasetsByUriSpace(ProfilerOptions options,
LIMERepositoryConnectionWrapper metadataConnection) {
try (RepositoryResult repositoryResult = metadataConnection.getStatements(null, VOID.URI_SPACE, null,
options.isIncludeInferred(), options.getContexts());
Stream stream = QueryResults.stream(repositoryResult)) {
return stream.collect(Collectors.toMap(s -> s.getObject().stringValue(), Statement::getSubject, (v1, v2) -> v1));
}
}
public static void appendUriSpaceLogic(ProfilerOptions options, IRI dataGraph, StringBuilder sb,
LIMERepositoryConnectionWrapper metadataConnection, String varPrefix, String referenceVar) {
Map datasetsByUriSpace = retrieveDatasetsByUriSpace(options, metadataConnection);
if (!datasetsByUriSpace.isEmpty() || !options.isDefaultToLocalReference()) {
sb.append(
// @formatter:off
" OPTIONAL { \n" +
" VALUES(?" + varPrefix + "referenceDataset ?" + varPrefix + "referenceDatasetUriSpaceT) { \n"
// @formatter:on
);
for (Map.Entry entry : datasetsByUriSpace.entrySet()) {
sb.append("(").append(NTriplesUtil.toNTriplesString(entry.getValue())).append(" ")
.append(NTriplesUtil.toNTriplesString(
SimpleValueFactory.getInstance().createLiteral(entry.getKey())))
.append(")");
}
sb.append(
// @formatter:off
" } \n" +
" FILTER(STRSTARTS(STR(?" + referenceVar + "), ?" + varPrefix + "referenceDatasetUriSpaceT)) \n" +
" } \n" +
" bind(IF(BOUND(?" + varPrefix + "referenceDatasetUriSpaceT), ?" + varPrefix +"referenceDatasetUriSpaceT, \n" +
" IF(not exists {graph "+ RenderUtils.toSPARQL(dataGraph)+" {?" + referenceVar + " a []}}, \n" +
" REPLACE(STR(?" + referenceVar + "), \"" + RenderUtils.escape(ResourceLocationUtils.RESOURCE_MENTION_NAMESPACE_PATTERN.pattern()) + "\", \"$1\"), \n" +
" ?unboundVariable)) as ?" + varPrefix + "referenceDatasetUriSpace) \n"
// @formatter:on
);
}
}
public static Resource getDatasetOrMintNew(ProfilerContext profilerContext, Map additionalDatasets,
Resource mainDataset, IRI resourceDataset, String uriSpace) {
if (resourceDataset != null) {
return resourceDataset;
} else {
if (uriSpace == null) {
return mainDataset;
} else {
Resource newDataset = additionalDatasets.get(uriSpace);
if (newDataset == null) {
newDataset = profilerContext.mintDatasetResource();
additionalDatasets.put(uriSpace, newDataset);
}
return newDataset;
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy