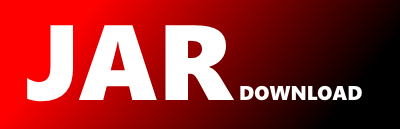
it.uniroma2.art.semanticturkey.changetracking.vocabulary.CHANGELOG Maven / Gradle / Ivy
package it.uniroma2.art.semanticturkey.changetracking.vocabulary;
import org.eclipse.rdf4j.model.IRI;
import org.eclipse.rdf4j.model.Namespace;
import org.eclipse.rdf4j.model.Value;
import org.eclipse.rdf4j.model.impl.SimpleNamespace;
import org.eclipse.rdf4j.model.impl.SimpleValueFactory;
import org.eclipse.rdf4j.model.vocabulary.SESAME;
import java.util.Objects;
/**
* Constant for the Changelog vocabulary used to represent the history of a repository. After the first
* commit, the history repository is expected to have a shape like the following:
*
*
*
* {@code
*
*
* a cl:Commit ;
* prov:startedAtTime "2017-05-09T22:39:51.410+02:00"^^ ;
* prov:endedAtTime "2017-05-09T22:39:51.420+02:00"^^ ;
* prov:generated ;
* prov:qualifiedAssociation [
* prov:agent ;
* prov:hadRole .
* ] ;
* cl:status "committed" ;
* cl:revisionNumber "0"^^xsd:integer .
*
* cl:addedStatement ;
* a prov:Entity ;
* prov:generatedAtTime "2017-05-09T22:39:51.419+02:00"^^ ;
* prov:wasGeneratedBy .
*
* a cl:Quadruple ;
* cl:context ;
* cl:object ;
* cl:predicate ;
* cl:subject .
*
* a cl:Commit ;
* prov:startedAtTime "2017-05-09T22:39:51.760+02:00"^^ ;
* prov:endedAtTime "2017-05-09T22:39:51.762+02:00"^^ ;
* prov:generated ;
* prov:qualifiedAssociation [
* prov:agent ;
* prov:hadRole .
* ] ;
* cl:status "committed" ;
* cl:revisionNumber "1"^^xsd:integer ;
* cl:parentCommit .
*
* a prov:Entity ;
* prov:generatedAtTime "2017-05-09T22:39:51.761+02:00"^^ ;
* prov:wasGeneratedBy ;
* cl:removedStatement .
*
* a cl:Quadruple ;
* cl:context ;
* cl:object ;
* cl:predicate ;
* cl:subject .
*
* cl:MASTER cl:tip .
* }
*
*
* The resource cl:MASTER
conventionally holds a reference to the tip of the history: i.e. the
* latest commit. Commits themselves are chained together via the property cl:parentCommit
: it
* connects a commit to the commit that was the tip when the update of the data repository was perfomed.
*
* Each commit may be described via a number of metadata properties, and above all it is connected to the
* triples effectively added or removed. In the history, the null context is represented via the resource
* {@link SESAME#NIL}.
*
* Usually, the metadata about changes to the data repository are recorded before of the actual change to the
* data. This approach guarantees that even in face of failures of the history repository no change can be
* lost in the history. The property cl:status
is used to indicate that a change described by a
* cl:Commit
has been effectively committed to the data repository.
*
* Usually, only the tip of the MASTER can be in an uncommitted state, because new commits are forbidden until
* the tip is committed. An uncommitted tip may indicate a change on fly against the data repository, or that
* there as been a failure:
*
* - the data repository has been updated, but the history repository could not update the status of the
* commit
* - the data repository failed to commit, but the history repository failed to undo the changes to the
* history
*
*
* Therefore, if we exclude an operation on fly, an "uncommitted" indicates an uncertainty on
* whether or not data have been effectively recorded.
*
*
* @author Manuel Fiorelli
*/
public abstract class CHANGELOG {
/** http://semanticturkey.uniroma2.it/ns/changelog# */
public static final String NAMESPACE = "http://semanticturkey.uniroma2.it/ns/changelog#";
/**
* Recommended prefix for the CHANGETRACKER namespace: "cl"
*/
public static final String PREFIX = "cl";
/**
* An immutable {@link Namespace} constant that represents the CHANGELOG namespace.
*/
public static final Namespace NS = new SimpleNamespace(PREFIX, NAMESPACE);
public static final IRI QUADRUPLE;
public static final IRI SUBJECT;
public static final IRI PREDICATE;
public static final IRI OBJECT;
public static final IRI CONTEXT;
public static final IRI REMOVED_STATEMENT;
public static final IRI ADDED_STATEMENT;
public static final IRI COMMIT;
public static final IRI PARENT_COMMIT;
public static final IRI STATUS;
public static final IRI MASTER;
public static final IRI TIP;
public static final IRI NULL;
public static final IRI REVISION_NUMBER;
public static boolean isNull(Value v) {
return Objects.equals(v, SESAME.NIL) || Objects.equals(v, NULL);
}
static {
SimpleValueFactory vf = SimpleValueFactory.getInstance();
QUADRUPLE = vf.createIRI(NAMESPACE, "Quadruple");
SUBJECT = vf.createIRI(NAMESPACE, "subject");
PREDICATE = vf.createIRI(NAMESPACE, "predicate");
OBJECT = vf.createIRI(NAMESPACE, "object");
CONTEXT = vf.createIRI(NAMESPACE, "context");
REMOVED_STATEMENT = vf.createIRI(NAMESPACE, "removedStatement");
ADDED_STATEMENT = vf.createIRI(NAMESPACE, "addedStatement");
COMMIT = vf.createIRI(NAMESPACE, "Commit");
PARENT_COMMIT = vf.createIRI(NAMESPACE, "parentCommit");
STATUS = vf.createIRI(NAMESPACE, "status");
MASTER = vf.createIRI(NAMESPACE, "MASTER");
TIP = vf.createIRI(NAMESPACE, "tip");
NULL = vf.createIRI(NAMESPACE, "null");
REVISION_NUMBER = vf.createIRI(NAMESPACE, "revisionNumber");
}
}