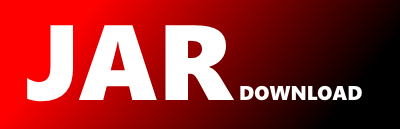
it.uniroma2.art.sheet2rdf.header.NodeSanitization Maven / Gradle / Ivy
The newest version!
package it.uniroma2.art.sheet2rdf.header;
public class NodeSanitization {
private Boolean trim;
private Boolean removeDuplicateSpaces;
private RemovePunctuation removePunctuation;
private Boolean lowerCase;
private Boolean upperCase;
public static NodeSanitization CODA_DEFAULT_SANITIZATION = new NodeSanitization(true, true, new RemovePunctuation(false), false, false);
public NodeSanitization() {
this.removePunctuation = new RemovePunctuation();
}
public NodeSanitization(Boolean trim, Boolean removeDuplicateSpaces, RemovePunctuation removePunctuation, Boolean lowerCase, Boolean upperCase) {
this.trim = trim;
this.removeDuplicateSpaces = removeDuplicateSpaces;
this.removePunctuation = removePunctuation;
this.lowerCase = lowerCase;
this.upperCase = upperCase;
}
public Boolean getTrim() {
return trim;
}
public void setTrim(Boolean trim) {
this.trim = trim;
}
public Boolean getRemoveDuplicateSpaces() {
return removeDuplicateSpaces;
}
public void setRemoveDuplicateSpaces(Boolean removeDuplicateSpaces) {
this.removeDuplicateSpaces = removeDuplicateSpaces;
}
public RemovePunctuation getRemovePunctuation() {
return removePunctuation;
}
public void setRemovePunctuation(RemovePunctuation removePunctuation) {
this.removePunctuation = removePunctuation;
}
public Boolean getLowerCase() {
return lowerCase;
}
public void setLowerCase(Boolean lowerCase) {
this.lowerCase = lowerCase;
}
public Boolean getUpperCase() {
return upperCase;
}
public void setUpperCase(Boolean upperCase) {
this.upperCase = upperCase;
}
public String serializeTrim() {
String s = "@Trim";
if (!trim) {
s += "(" + trim + ")";
}
return s;
}
public String serializeRemoveDuplicateSpaces() {
String s = "@RemoveDuplicateSpaces";
if (!removeDuplicateSpaces) {
s += "(" + removeDuplicateSpaces + ")";
}
return s;
}
public String serializeRemovePunctuation() {
String s = "@RemovePunctuation";
if (removePunctuation.getChars() != null) {
s += "(\"" + removePunctuation.getChars() + "\")";
} else if (!removePunctuation.getEnabled()) {
s += "(" + removePunctuation.getEnabled() + ")";
}
return s;
}
public String serializeLowerCase() {
String s = "@LowerCase";
if (lowerCase) {
s += "(" + lowerCase + ")";
}
return s;
}
public String serializeUpperCase() {
String s = "@UpperCase";
if (upperCase) {
s += "(" + upperCase + ")";
}
return s;
}
/**
* Returns a new sanitization structure with the values of the first one provided with
* fallback of the second one for those attributes not provided
* @param sanitization
* @param fallback
* @return
*/
public static NodeSanitization merge(NodeSanitization sanitization, NodeSanitization fallback) {
NodeSanitization merged = new NodeSanitization();
if (sanitization.getTrim() != null) {
merged.setTrim(sanitization.getTrim());
} else {
merged.setTrim(fallback.getTrim());
}
if (sanitization.getRemoveDuplicateSpaces() != null) {
merged.setRemoveDuplicateSpaces(sanitization.getRemoveDuplicateSpaces());
} else {
merged.setRemoveDuplicateSpaces(fallback.getRemoveDuplicateSpaces());
}
if (sanitization.getRemovePunctuation().getEnabled() != null) {
merged.setRemovePunctuation(sanitization.getRemovePunctuation());
} else {
merged.setRemovePunctuation(fallback.getRemovePunctuation());
}
if (sanitization.getLowerCase() != null) {
merged.setLowerCase(sanitization.getLowerCase());
} else {
merged.setLowerCase(fallback.getLowerCase());
}
if (sanitization.getUpperCase() != null) {
merged.setUpperCase(sanitization.getUpperCase());
} else {
merged.setUpperCase(fallback.getUpperCase());
}
return merged;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy