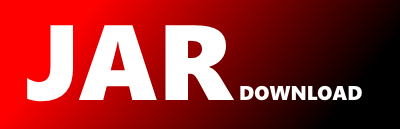
it.uniroma2.art.sheet2rdf.pearl.PearlBuilder Maven / Gradle / Ivy
The newest version!
package it.uniroma2.art.sheet2rdf.pearl;
import it.uniroma2.art.sheet2rdf.header.NodeSanitization;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Set;
public class PearlBuilder {
private final Map prefixMapping;
private final NodeSanitization ruleSanitization;
private final String rule;
private final List nodeSection;
private final List graphSection;
public PearlBuilder(Map prefixMapping, String annotationType,
NodeSanitization ruleSanitization, List nodeSection, List graphSection) {
this.prefixMapping = prefixMapping;
this.ruleSanitization = ruleSanitization;
this.rule = "rule " + annotationType + " id:row";
this.nodeSection = nodeSection;
this.graphSection = graphSection;
}
/**
* Serializes the pearl code.
*
* @return
*/
public String serialize() {
int indent = 0;//increases right after { opening, decreases right before } closing.
String tabs = getTabs(indent);
String pearl = "";
//serialize prefix namespace mapping
Set prefs = prefixMapping.keySet();
for (String pref : prefs) {
pearl += tabs + "prefix " + pref + ": <" + prefixMapping.get(pref) + ">\n";
}
pearl += tabs + "\n";
if (ruleSanitization.getTrim() != null && !ruleSanitization.getTrim().equals(NodeSanitization.CODA_DEFAULT_SANITIZATION.getTrim())) {
//Trim specified in rule and different from the default behaviour => write it
pearl += tabs + ruleSanitization.serializeTrim() + "\n";
}
if (ruleSanitization.getRemoveDuplicateSpaces() != null && !ruleSanitization.getRemoveDuplicateSpaces().equals(NodeSanitization.CODA_DEFAULT_SANITIZATION.getRemoveDuplicateSpaces())) {
//RemoveDuplicateSpaces specified in rule and different from the default behaviour => write it
pearl += tabs + ruleSanitization.serializeRemoveDuplicateSpaces() + "\n";
}
if (ruleSanitization.getRemovePunctuation().getEnabled() != null && ruleSanitization.getRemovePunctuation().getEnabled()) {
pearl += tabs + ruleSanitization.serializeRemovePunctuation() + "\n";
}
if (ruleSanitization.getUpperCase() != null && !ruleSanitization.getUpperCase().equals(NodeSanitization.CODA_DEFAULT_SANITIZATION.getUpperCase())) {
//LowerCase specified in rule and different from the default behaviour => write it
pearl += tabs + ruleSanitization.serializeUpperCase() + "\n";
}
if (ruleSanitization.getLowerCase() != null && !ruleSanitization.getLowerCase().equals(NodeSanitization.CODA_DEFAULT_SANITIZATION.getLowerCase())) {
//LowerCase specified in rule and different from the default behaviour => write it
pearl += tabs + ruleSanitization.serializeLowerCase() + "\n";
}
//serialize rule
pearl += tabs + rule + " {\n";
indent++;
tabs = getTabs(indent);
//serialize node section
pearl += tabs + "nodes = {\n";
indent++;
tabs = getTabs(indent);
for (NodePearlElement n : nodeSection) {
pearl += n.serialize(tabs, prefixMapping);
}
indent--;
tabs = getTabs(indent);
pearl += tabs + "}\n";//closing node section
//serialize graph section (or insert - delete)
List insertElement = new ArrayList<>();
List deleteElement = new ArrayList<>();
for (GraphPearlElement g : graphSection) {
if (g.isDelete()) {
deleteElement.add(g);
} else {
insertElement.add(g);
}
}
//graph/insert
String insertSectionName = deleteElement.isEmpty() ? "graph" : "insert";
pearl += tabs + insertSectionName + " = {\n"; //open graph/insert section
indent++;
tabs = getTabs(indent);
for (GraphPearlElement g : insertElement) {
pearl += g.serialize(tabs, prefixMapping) + "\n";
}
indent--;
tabs = getTabs(indent);
pearl += tabs + "}\n";//close graph/insert section
//delete (only if present)
if (!deleteElement.isEmpty()) {
pearl += tabs + "delete = {\n"; //open delete section
indent++;
tabs = getTabs(indent);
for (GraphPearlElement g : deleteElement) {
pearl += g.serialize(tabs, prefixMapping) + "\n";
}
indent--;
tabs = getTabs(indent);
pearl += tabs + "}\n";//close delete section
}
indent--;
tabs = getTabs(indent);
pearl += tabs + "}\n";//closing rule
return pearl;
}
private String getTabs(int indent) {
String tabs = "";
for (int i = 0; i < indent; ++i)
tabs += "\t";
return tabs;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy