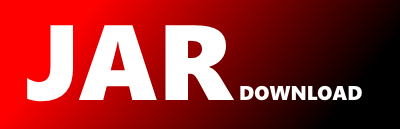
jakarta.faces.application.StateManager Maven / Gradle / Ivy
Show all versions of jakarta.faces-api Show documentation
/*
* Copyright (c) 1997, 2021 Oracle and/or its affiliates. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v. 2.0, which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* This Source Code may also be made available under the following Secondary
* Licenses when the conditions for such availability set forth in the
* Eclipse Public License v. 2.0 are satisfied: GNU General Public License,
* version 2 with the GNU Classpath Exception, which is available at
* https://www.gnu.org/software/classpath/license.html.
*
* SPDX-License-Identifier: EPL-2.0 OR GPL-2.0 WITH Classpath-exception-2.0
*/
package jakarta.faces.application;
import static java.lang.Boolean.TRUE;
import java.io.IOException;
import java.util.Map;
import jakarta.faces.context.FacesContext;
import jakarta.faces.context.ResponseWriter;
import jakarta.faces.render.RenderKit;
import jakarta.faces.render.ResponseStateManager;
import jakarta.faces.view.StateManagementStrategy;
import jakarta.faces.view.ViewDeclarationLanguage;
/**
*
* StateManager directs the process of saving and restoring the view between requests.
* An implementation of this class must be thread-safe. The {@link StateManager}
* instance for an application is retrieved from the {@link Application} instance, and thus cannot know any details of
* the markup language created by the {@link RenderKit} being used to render a view.
*
* The {@link StateManager} utilizes a helper object ({@link ResponseStateManager}), that is provided by the
* {@link RenderKit} implementation and is therefore aware of the markup language details.
*
*/
public abstract class StateManager {
// ------------------------------------------------------ Manifest Constants
/**
*
* The ServletContext
init parameter consulted by the StateManager
to tell where the state
* should be saved. Valid values are given as the values of the constants: {@link #STATE_SAVING_METHOD_CLIENT} or
* {@link #STATE_SAVING_METHOD_SERVER}.
*
*
*
* If this parameter is not specified, the default value is the value of the constant
* {@link #STATE_SAVING_METHOD_CLIENT}.
*
*/
public static final String STATE_SAVING_METHOD_PARAM_NAME = "jakarta.faces.STATE_SAVING_METHOD";
/**
*
* The ServletContext
init parameter consulted by the runtime to determine if the partial state saving
* mechanism should be used.
*
*
*
*
*
* If undefined, the runtime must determine the version level of the application.
*
*
*
*
* -
*
* For applications versioned at 1.2 and under, the runtime must not use the partial state saving mechanism.
*
*
*
* -
*
* For applications versioned at 2.0 and above, the runtime must use the partial state saving mechanism.
*
*
*
*
*
*
* If this parameter is defined, and the application is versioned at 1.2 and under, the runtime must not use the partial
* state saving mechanism. Otherwise, If this param is defined, and calling toLowerCase().equals("true")
on
* a String
representation of its value returns true
, the runtime must use partial state
* mechanism. Otherwise the partial state saving mechanism must not be used.
*
*
*
*
* @since 2.0
* @deprecated Full state saving will be removed in favor of partial state saving in order to keep the spec simple.
* Therefore disabling partial state saving via this context parameter will not anymore be an option.
*/
@Deprecated(forRemoval = true, since = "4.1")
public static final String PARTIAL_STATE_SAVING_PARAM_NAME = "jakarta.faces.PARTIAL_STATE_SAVING";
/**
*
* The runtime must interpret the value of this parameter as a comma separated list of view IDs, each of which must have
* their state saved using the state saving mechanism specified in Jakarta Faces 1.2.
*
*
* @deprecated Full state saving will be removed in favor of partial state saving in order to keep the spec simple.
* Therefore specifying full state saving view IDs via this context parameter will not anymore be an option.
*/
@Deprecated(forRemoval = true, since = "4.1")
public static final String FULL_STATE_SAVING_VIEW_IDS_PARAM_NAME = "jakarta.faces.FULL_STATE_SAVING_VIEW_IDS";
/**
*
* Marker within the FacesContext
attributes map to indicate we are saving state. The implementation must
* set this marker into the map before starting the state saving traversal and the marker must be cleared, in a
* finally block, after the traversal is complete.
*
*/
public static final String IS_SAVING_STATE = "jakarta.faces.IS_SAVING_STATE";
/**
*
* Marker within the FacesContext
attributes map to indicate we are marking initial state, so the
* markInitialState()
method of iterating components such as {@link jakarta.faces.component.UIData} could
* recognize this fact and save the initial state of descendents.
*
*
* @since 2.1
*
*/
public final static String IS_BUILDING_INITIAL_STATE = "jakarta.faces.IS_BUILDING_INITIAL_STATE";
/**
*
* If this param is set, and calling toLowerCase().equals("true") on a String representation of its value returns true,
* and the jakarta.faces.STATE_SAVING_METHOD is set to "server" (as indicated below), the server state must be
* guaranteed to be Serializable such that the aggregate state implements java.io.Serializable. The intent of this
* parameter is to ensure that the act of writing out the state to an ObjectOutputStream would not throw a
* NotSerializableException, but the runtime is not required verify this before saving the state.
*
*
* @since 2.2
*/
public static final String SERIALIZE_SERVER_STATE_PARAM_NAME = "jakarta.faces.SERIALIZE_SERVER_STATE";
/**
*
* Constant value for the initialization parameter named by the STATE_SAVING_METHOD_PARAM_NAME
that
* indicates state saving should take place on the client.
*
*/
public static final String STATE_SAVING_METHOD_CLIENT = "client";
/**
*
* Constant value for the initialization parameter named by the STATE_SAVING_METHOD_PARAM_NAME
that
* indicates state saving should take place on the server.
*
*/
public static final String STATE_SAVING_METHOD_SERVER = "server";
private Boolean savingStateInClient;
// ---------------------------------------------------- State Saving Methods
/**
*
* Save the state represented in the specified state Object
instance, in an implementation dependent
* manner.
*
*
*
* This method will typically simply delegate the actual writing to the writeState()
method of the
* {@link ResponseStateManager} instance provided by the {@link RenderKit} being used to render this view. This method
* assumes that the caller has positioned the {@link ResponseWriter} at the correct position for the saved state to be
* written.
*
*
* @param context {@link FacesContext} for the current request
* @param state the state to be written
* @throws IOException when an I/O error occurs.
* @since 1.2
*/
public void writeState(FacesContext context, Object state) throws IOException {
}
// ------------------------------------------------- State Restoring Methods
/**
*
* Method to determine if the state is saved on the client.
*
*
* @param context the Faces context.
* @return true
if and only if the value of the ServletContext
init parameter named by the
* value of the constant {@link #STATE_SAVING_METHOD_PARAM_NAME} is equal (ignoring
* case) to the value of the constant {@link #STATE_SAVING_METHOD_CLIENT}. false
otherwise.
*
* @throws NullPointerException if context
is null
.
*/
public boolean isSavingStateInClient(FacesContext context) {
if (savingStateInClient != null) {
return savingStateInClient;
}
savingStateInClient = false;
String saveStateParam = context.getExternalContext().getInitParameter(STATE_SAVING_METHOD_PARAM_NAME);
if (saveStateParam != null && saveStateParam.equalsIgnoreCase(STATE_SAVING_METHOD_CLIENT)) {
savingStateInClient = true;
}
return savingStateInClient;
}
/**
*
* Convenience method to return the view state as a String
with no RenderKit
specific markup.
*
* This default implementation of this method will call {@link StateManagementStrategy#saveView(FacesContext)} and
* passing the result to and returning the resulting value from
* {@link ResponseStateManager#getViewState(jakarta.faces.context.FacesContext, Object)}.
*
*
* @param context {@link FacesContext} for the current request
* @return the view state.
* @since 2.0
*/
public String getViewState(FacesContext context) {
Object savedView = null;
if (context != null && !context.getViewRoot().isTransient()) {
String viewId = context.getViewRoot().getViewId();
ViewDeclarationLanguage vdl = context.getApplication().getViewHandler().getViewDeclarationLanguage(context, viewId);
if (vdl != null) {
Map