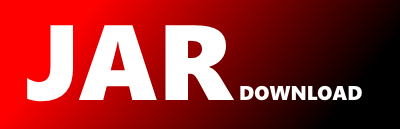
jakarta.faces.model.SelectItem Maven / Gradle / Ivy
Show all versions of jakarta.faces-api Show documentation
/*
* Copyright (c) 1997, 2020 Oracle and/or its affiliates. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v. 2.0, which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* This Source Code may also be made available under the following Secondary
* Licenses when the conditions for such availability set forth in the
* Eclipse Public License v. 2.0 are satisfied: GNU General Public License,
* version 2 with the GNU Classpath Exception, which is available at
* https://www.gnu.org/software/classpath/license.html.
*
* SPDX-License-Identifier: EPL-2.0 OR GPL-2.0 WITH Classpath-exception-2.0
*/
package jakarta.faces.model;
import java.io.Serializable;
import jakarta.faces.component.UISelectMany;
import jakarta.faces.component.UISelectOne;
/**
*
* SelectItem represents a single item
* in the list of supported items associated with a {@link UISelectMany} or {@link UISelectOne} component.
*
*/
public class SelectItem implements Serializable {
private static final long serialVersionUID = 876782311414654999L;
// ------------------------------------------------------------ Constructors
/**
*
* Construct a SelectItem
with no initialized property values.
*
*/
public SelectItem() {
super();
}
/**
*
* Construct a SelectItem
with the specified value. The label
property will be set to the
* value (converted to a String, if necessary), the description
property will be set to null
,
* the disabled
property will be set to false
, and the escape
property will be
* set to ( true
.
*
*
* @param value Value to be delivered to the model if this item is selected by the user
*/
public SelectItem(Object value) {
this(value, value == null ? null : value.toString(), null, false, true, false);
}
/**
*
* Construct a SelectItem
with the specified value and label. The description
property will be
* set to null
, the disabled
property will be set to false
, and the
* escape
property will be set to true
.
*
*
* @param value Value to be delivered to the model if this item is selected by the user
* @param label Label to be rendered for this item in the response
*/
public SelectItem(Object value, String label) {
this(value, label, null, false, true, false);
}
/**
*
* Construct a SelectItem
instance with the specified value, label and description. This
* disabled
property will be set to false
, and the escape
property will be set to
* true
.
*
*
* @param value Value to be delivered to the model if this item is selected by the user
* @param label Label to be rendered for this item in the response
* @param description Description of this item, for use in tools
*/
public SelectItem(Object value, String label, String description) {
this(value, label, description, false, true, false);
}
/**
*
* Construct a SelectItem
instance with the specified property values. The escape
property
* will be set to true
.
*
*
* @param value Value to be delivered to the model if this item is selected by the user
* @param label Label to be rendered for this item in the response
* @param description Description of this item, for use in tools
* @param disabled Flag indicating that this option is disabled
*/
public SelectItem(Object value, String label, String description, boolean disabled) {
this(value, label, description, disabled, true, false);
}
/**
*
* Construct a SelectItem
instance with the specified property values.
*
*
* @param value Value to be delivered to the model if this item is selected by the user
* @param label Label to be rendered for this item in the response
* @param description Description of this item, for use in tools
* @param disabled Flag indicating that this option is disabled
* @param escape Flag indicating that the text of this option should be escaped when rendered.
* @since 1.2
*/
public SelectItem(Object value, String label, String description, boolean disabled, boolean escape) {
this(value, label, description, disabled, escape, false);
}
/**
*
* Construct a SelectItem
instance with the specified property values.
*
*
* @param value Value to be delivered to the model if this item is selected by the user
* @param label Label to be rendered for this item in the response
* @param description Description of this item, for use in tools
* @param disabled Flag indicating that this option is disabled
* @param escape Flag indicating that the text of this option should be escaped when rendered.
* @param noSelectionOption Flag indicating that the current option is a "no selection" option
* @since 1.2
*/
public SelectItem(Object value, String label, String description, boolean disabled, boolean escape, boolean noSelectionOption) {
super();
setValue(value);
setLabel(label);
setDescription(description);
setDisabled(disabled);
setEscape(escape);
setNoSelectionOption(noSelectionOption);
}
// ------------------------------------------------------ Instance Variables
private String description = null;
private boolean disabled = false;
private String label = null;
@SuppressWarnings({ "NonSerializableFieldInSerializableClass" })
private Object value = null;
// -------------------------------------------------------------- Properties
/**
*
* Return a description of this item, for use in development tools.
*
* @return a description of this item, for use in development tools
*/
public String getDescription() {
return description;
}
/**
*
* Set the description of this item, for use in development tools.
*
*
* @param description The new description
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* Return the disabled flag for this item, which should modify the rendered output to make this item unavailable for
* selection by the user if set to true
.
*
*
* @return the disabled flag for this item
*/
public boolean isDisabled() {
return disabled;
}
/**
*
* Set the disabled flag for this item, which should modify the rendered output to make this item unavailable for
* selection by the user if set to true
.
*
*
* @param disabled The new disabled flag
*/
public void setDisabled(boolean disabled) {
this.disabled = disabled;
}
/**
*
* Return the label of this item, to be rendered visibly for the user.
*
* @return the label of this item
*/
public String getLabel() {
return label;
}
/**
*
* Set the label of this item, to be rendered visibly for the user.
*
* @param label The new label
*/
public void setLabel(String label) {
this.label = label;
}
/**
*
* Return the value of this item, to be delivered to the model if this item is selected by the user.
*
* @return the value of this item
*/
public Object getValue() {
return value;
}
/**
*
* Set the value of this item, to be delivered to the model if this item is selected by this user.
*
* @param value The new value
*
*/
public void setValue(Object value) {
this.value = value;
}
private boolean escape;
/**
*
* If and only if this returns true
, the code that renders this select item must escape the label using
* escaping syntax appropriate to the content type being rendered.
*
*
* @return the escape value.
* @since 2.0
*/
public boolean isEscape() {
return escape;
}
/**
*
* Set the value of the escape property. See {@link #isEscape}.
*
*
* @param escape the new value of the escape property
*
* @since 2.0
*/
public void setEscape(boolean escape) {
this.escape = escape;
}
private boolean noSelectionOption = false;
/**
*
* Return the value of the noSelectionOption
property. If the value of this property is true
,
* the system interprets the option represented by this SelectItem
instance as representing a "no
* selection" option. See {@link UISelectOne#validateValue} and {@link UISelectMany#validateValue} for usage.
*
*
* @return the value of the noSelectionOption
property
*
* @since 2.0
*/
public boolean isNoSelectionOption() {
return noSelectionOption;
}
/**
*
* Set the value of the noSelectionOption
property.
*
*
* @param noSelectionOption the new value of the {@code noSelectionOption} property
*
* @since 2.0
*/
public void setNoSelectionOption(boolean noSelectionOption) {
this.noSelectionOption = noSelectionOption;
}
}