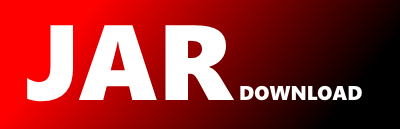
jakarta.ws.rs.core.UriInfo Maven / Gradle / Ivy
/*
* Copyright (c) 2010, 2019 Oracle and/or its affiliates. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v. 2.0, which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* This Source Code may also be made available under the following Secondary
* Licenses when the conditions for such availability set forth in the
* Eclipse Public License v. 2.0 are satisfied: GNU General Public License,
* version 2 with the GNU Classpath Exception, which is available at
* https://www.gnu.org/software/classpath/license.html.
*
* SPDX-License-Identifier: EPL-2.0 OR GPL-2.0 WITH Classpath-exception-2.0
*/
package jakarta.ws.rs.core;
import java.net.URI;
import java.util.List;
/**
* An injectable interface that provides access to application and request URI information. Relative URIs are relative
* to the base URI of the application, see {@link #getBaseUri}.
*
*
* All methods throw {@code java.lang.IllegalStateException} if called outside the scope of a request (e.g. from a
* provider constructor).
*
*
* @author Paul Sandoz
* @author Marc Hadley
* @see Context
* @since 1.0
*/
public interface UriInfo {
/**
* Get the path of the current request relative to the base URI as a string. All sequences of escaped octets are
* decoded, equivalent to {@link #getPath(boolean) getPath(true)}.
*
* @return the relative URI path.
* @throws java.lang.IllegalStateException if called outside the scope of a request.
*/
public String getPath();
/**
* Get the path of the current request relative to the base URI as a string.
*
* @param decode controls whether sequences of escaped octets are decoded ({@code true}) or not ({@code false}).
* @return the relative URI path
* @throws java.lang.IllegalStateException if called outside the scope of a request.
*/
public String getPath(boolean decode);
/**
* Get the path of the current request relative to the base URI as a list of {@link PathSegment}. This method is useful
* when the path needs to be parsed, particularly when matrix parameters may be present in the path. All sequences of
* escaped octets in path segments and matrix parameter values are decoded, equivalent to {@code getPathSegments(true)}.
*
* @return an unmodifiable list of {@link PathSegment}. The matrix parameter map of each path segment is also
* unmodifiable.
* @throws IllegalStateException if called outside the scope of a request
* @see PathSegment
* @see Matrix URIs
*/
public List getPathSegments();
/**
* Get the path of the current request relative to the base URI as a list of {@link PathSegment}. This method is useful
* when the path needs to be parsed, particularly when matrix parameters may be present in the path.
*
* @param decode controls whether sequences of escaped octets in path segments and matrix parameter values are decoded
* ({@code true}) or not ({@code false}).
* @return an unmodifiable list of {@link PathSegment}. The matrix parameter map of each path segment is also
* unmodifiable.
* @throws java.lang.IllegalStateException if called outside the scope of a request
* @see PathSegment
* @see Matrix URIs
*/
public List getPathSegments(boolean decode);
/**
* Get the absolute request URI including any query parameters.
*
* @return the absolute request URI
* @throws java.lang.IllegalStateException if called outside the scope of a request
*/
public URI getRequestUri();
/**
* Get the absolute request URI in the form of a UriBuilder.
*
* @return a UriBuilder initialized with the absolute request URI.
* @throws java.lang.IllegalStateException if called outside the scope of a request.
*/
public UriBuilder getRequestUriBuilder();
/**
* Get the absolute path of the request. This includes everything preceding the path (host, port etc) but excludes query
* parameters. This is a shortcut for {@code uriInfo.getBaseUri().resolve(uriInfo.getPath(false))}.
*
* @return the absolute path of the request.
* @throws java.lang.IllegalStateException if called outside the scope of a request.
*/
public URI getAbsolutePath();
/**
* Get the absolute path of the request in the form of a UriBuilder. This includes everything preceding the path (host,
* port etc) but excludes query parameters.
*
* @return a UriBuilder initialized with the absolute path of the request.
* @throws java.lang.IllegalStateException if called outside the scope of a request.
*/
public UriBuilder getAbsolutePathBuilder();
/**
* Get the base URI of the application. URIs of root resource classes are all relative to this base URI.
*
* @return the base URI of the application.
*/
public URI getBaseUri();
/**
* Get the base URI of the application in the form of a UriBuilder.
*
* @return a UriBuilder initialized with the base URI of the application.
*/
public UriBuilder getBaseUriBuilder();
/**
* Get the values of any embedded URI template parameters. All sequences of escaped octets are decoded, equivalent to
* {@link #getPathParameters(boolean) getPathParameters(true)}.
*
* @return an unmodifiable map of parameter names and values.
* @throws java.lang.IllegalStateException if called outside the scope of a request.
* @see jakarta.ws.rs.Path
* @see jakarta.ws.rs.PathParam
*/
public MultivaluedMap getPathParameters();
/**
* Get the values of any embedded URI template parameters.
*
* @param decode controls whether sequences of escaped octets are decoded ({@code true}) or not ({@code false}).
* @return an unmodifiable map of parameter names and values
* @throws java.lang.IllegalStateException if called outside the scope of a request.
* @see jakarta.ws.rs.Path
* @see jakarta.ws.rs.PathParam
*/
public MultivaluedMap getPathParameters(boolean decode);
/**
* Get the URI query parameters of the current request. The map keys are the names of the query parameters with any
* escaped characters decoded. All sequences of escaped octets in parameter names and values are decoded, equivalent to
* {@link #getQueryParameters(boolean) getQueryParameters(true)}.
*
* @return an unmodifiable map of query parameter names and values.
* @throws java.lang.IllegalStateException if called outside the scope of a request.
*/
public MultivaluedMap getQueryParameters();
/**
* Get the URI query parameters of the current request. The map keys are the names of the query parameters with any
* escaped characters decoded.
*
* @param decode controls whether sequences of escaped octets in parameter names and values are decoded ({@code true})
* or not ({@code false}).
* @return an unmodifiable map of query parameter names and values.
* @throws java.lang.IllegalStateException if called outside the scope of a request.
*/
public MultivaluedMap getQueryParameters(boolean decode);
/**
* Get a read-only list of URIs for matched resources.
*
* Each entry is a relative URI that matched a resource class, a sub-resource method or a sub-resource locator. All
* sequences of escaped octets are decoded, equivalent to {@code getMatchedURIs(true)}. Entries do not include query
* parameters but do include matrix parameters if present in the request URI. Entries are ordered in reverse request URI
* matching order, with the current resource URI first. E.g. given the following resource classes:
*
*
* @Path("foo")
* public class FooResource {
* @GET
* public String getFoo() {...}
*
* @Path("bar")
* public BarResource getBarResource() {...}
* }
*
* public class BarResource {
* @GET
* public String getBar() {...}
* }
*
*
*
* The values returned by this method based on request uri and where the method is called from are:
*
*
*
* Matched URIs from requests
*
* Request
* Called from
* Value(s)
*
*
* GET /foo
* FooResource.getFoo
* foo
*
*
* GET /foo/bar
* FooResource.getBarResource
* foo/bar, foo
*
*
* GET /foo/bar
* BarResource.getBar
* foo/bar, foo
*
*
*
* In case the method is invoked prior to the request matching (e.g. from a pre-matching filter), the method returns an
* empty list.
*
* @return a read-only list of URI paths for matched resources.
*/
public List getMatchedURIs();
/**
* Get a read-only list of URIs for matched resources.
*
* Each entry is a relative URI that matched a resource class, a sub-resource method or a sub-resource locator. Entries
* do not include query parameters but do include matrix parameters if present in the request URI. Entries are ordered
* in reverse request URI matching order, with the current resource URI first. See {@link #getMatchedURIs()} for an
* example.
*
* In case the method is invoked prior to the request matching (e.g. from a pre-matching filter), the method returns an
* empty list.
*
* @param decode controls whether sequences of escaped octets are decoded ({@code true}) or not ({@code false}).
* @return a read-only list of URI paths for matched resources.
*/
public List getMatchedURIs(boolean decode);
/**
* Get a read-only list of the currently matched resource class instances.
*
* Each entry is a resource class instance that matched the request URI either directly or via a sub-resource method or
* a sub-resource locator. Entries are ordered according to reverse request URI matching order, with the current
* resource first. E.g. given the following resource classes:
*
*
* @Path("foo")
* public class FooResource {
* @GET
* public String getFoo() {...}
*
* @Path("bar")
* public BarResource getBarResource() {...}
* }
*
* public class BarResource {
* @GET
* public String getBar() {...}
* }
*
*
*
* The values returned by this method based on request uri and where the method is called from are:
*
*
*
* Matched resources from requests
*
* Request
* Called from
* Value(s)
*
*
* GET /foo
* FooResource.getFoo
* FooResource
*
*
* GET /foo/bar
* FooResource.getBarResource
* FooResource
*
*
* GET /foo/bar
* BarResource.getBar
* BarResource, FooResource
*
*
*
* In case the method is invoked prior to the request matching (e.g. from a pre-matching filter), the method returns an
* empty list.
*
* @return a read-only list of matched resource class instances.
*/
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy