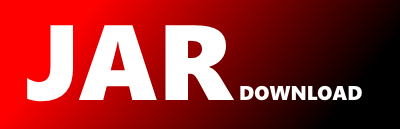
javax.faces.component.ValueHolder Maven / Gradle / Ivy
/*
* $Id: ValueHolder.java,v 1.19 2007/01/29 07:56:02 rlubke Exp $
*/
/*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the License). You may not use this file except in
* compliance with the License.
*
* You can obtain a copy of the License at
* https://javaserverfaces.dev.java.net/CDDL.html or
* legal/CDDLv1.0.txt.
* See the License for the specific language governing
* permission and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* Header Notice in each file and include the License file
* at legal/CDDLv1.0.txt.
* If applicable, add the following below the CDDL Header,
* with the fields enclosed by brackets [] replaced by
* your own identifying information:
* "Portions Copyrighted [year] [name of copyright owner]"
*
* [Name of File] [ver.__] [Date]
*
* Copyright 2005 Sun Microsystems Inc. All Rights Reserved
*/
package javax.faces.component;
import javax.faces.convert.Converter;
import javax.el.ValueExpression;
/**
* ValueHolder is an interface that may be implemented
* by any concrete {@link UIComponent} that wishes to support a local
* value, as well as access data in the model tier via a value
* expression, and support conversion
* between String and the model tier data's native data type.
*/
public interface ValueHolder {
// -------------------------------------------------------------- Properties
/**
*
Return the local value of this {@link UIComponent} (if any),
* without evaluating any associated {@link ValueExpression}.
*/
public Object getLocalValue();
/**
* Gets the value of this {@link UIComponent}. First, consult
* the local value property of this component. If
* non-null
return it. If null
, see if we have a
* {@link ValueExpression} for the value
property. If
* so, return the result of evaluating the property, otherwise
* return null
. Note that because the specification for {@link
* UIComponent#setValueBinding} requires a
* call through to {@link UIComponent#setValueExpression}, legacy
* tags will continue to work.
*/
public Object getValue();
/**
* Set the value of this {@link UIComponent} (if any).
*
* @param value The new local value
*/
public void setValue(Object value);
/**
* Return the {@link Converter} (if any)
* that is registered for this {@link UIComponent}.
*/
public Converter getConverter();
/**
* Set the {@link Converter} (if any)
* that is registered for this {@link UIComponent}.
*
* @param converter New {@link Converter} (or null
)
*/
public void setConverter(Converter converter);
}