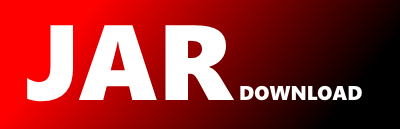
javax.jms.JMSConsumer Maven / Gradle / Ivy
/*
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS HEADER.
*
* Copyright (c) 2011-2012 Oracle and/or its affiliates. All rights reserved.
*
* The contents of this file are subject to the terms of either the GNU
* General Public License Version 2 only ("GPL") or the Common Development
* and Distribution License("CDDL") (collectively, the "License"). You
* may not use this file except in compliance with the License. You can
* obtain a copy of the License at
* https://glassfish.dev.java.net/public/CDDL+GPL_1_1.html
* or packager/legal/LICENSE.txt. See the License for the specific
* language governing permissions and limitations under the License.
*
* When distributing the software, include this License Header Notice in each
* file and include the License file at packager/legal/LICENSE.txt.
*
* GPL Classpath Exception:
* Oracle designates this particular file as subject to the "Classpath"
* exception as provided by Oracle in the GPL Version 2 section of the License
* file that accompanied this code.
*
* Modifications:
* If applicable, add the following below the License Header, with the fields
* enclosed by brackets [] replaced by your own identifying information:
* "Portions Copyright [year] [name of copyright owner]"
*
* Contributor(s):
* If you wish your version of this file to be governed by only the CDDL or
* only the GPL Version 2, indicate your decision by adding "[Contributor]
* elects to include this software in this distribution under the [CDDL or GPL
* Version 2] license." If you don't indicate a single choice of license, a
* recipient has the option to distribute your version of this file under
* either the CDDL, the GPL Version 2 or to extend the choice of license to
* its licensees as provided above. However, if you add GPL Version 2 code
* and therefore, elected the GPL Version 2 license, then the option applies
* only if the new code is made subject to such option by the copyright
* holder.
*/
package javax.jms;
/**
* A client using the simplified JMS API introduced for JMS 2.0 uses a JMSConsumer
* object to receive messages from a destination. A JMSConsumer
* object is created by passing a Destination
object to one of the
* createConsumer
or createDurableConsumer
methods on
* a JMSContext
.
*
* A JMSConsumer
can be created with a message selector. A
* message selector allows the client to restrict the messages delivered to the
* JMSConsumer
to those that match the selector.
*
* A client may either synchronously receive a JMSConsumer
's
* messages or have the JMSConsumer
asynchronously deliver them
* as they arrive.
*
* For synchronous receipt, a client can request the next message from a
* JMSConsumer
using one of its receive
methods. There are several
* variations of receive
that allow a client to poll or wait for the next message.
*
* For asynchronous delivery, a client can register a MessageListener
object
* with a JMSConsumer
.
* As messages arrive at the JMSConsumer
, it delivers them by calling
* the MessageListener
's onMessage
method.
*
* It is a client programming error for a MessageListener
to throw an exception.
*
* @version 2.0
*
* @see javax.jms.JMSContext
*/
public interface JMSConsumer {
/** Gets this JMSConsumer
's message selector expression.
*
* @return this JMSConsumer
's message selector, or null if no
* message selector exists for the JMSConsumer
(that is, if
* the message selector was not set or was set to null or the
* empty string)
*
* @exception JMSRuntimeException if the JMS provider fails to get the message
* selector due to some internal error.
*/
String getMessageSelector();
/** Gets the JMSConsumer
's MessageListener
.
*
* This method must not be used in a Java EE web or EJB application.
* Doing so may cause a JMSRuntimeException
to be thrown though this is not guaranteed.
*
* @return the JMSConsumer
's MessageListener
, or null if one was not set
*
* @exception JMSRuntimeException if the JMS provider fails to get the MessageListener
* for one of the following reasons:
*
* - an internal error has occurred or
*
- this method has been called in a Java EE web or EJB application
* (though it is not guaranteed that an exception is thrown in this case)
*
*
* @see javax.jms.JMSConsumer#setMessageListener(javax.jms.MessageListener)
*/
MessageListener getMessageListener() throws JMSRuntimeException;
/** Sets the JMSConsumer
's MessageListener
.
*
* Setting the MessageListener
to null is the equivalent of
* unsetting the MessageListener
for the JMSConsumer
.
*
* The effect of calling this method
* while messages are being consumed by an existing listener
* or the JMSConsumer
is being used to consume messages synchronously
* is undefined.
*
* This method must not be used in a Java EE web or EJB application.
* Doing so may cause a JMSRuntimeException
to be thrown though this is not guaranteed.
*
* @param listener the listener to which the messages are to be
* delivered
*
* @exception JMSRuntimeException if the JMS provider fails to set the JMSConsumer
's MessageListener
* for one of the following reasons:
*
* - an internal error has occurred or
*
- this method has been called in a Java EE web or EJB application
* (though it is not guaranteed that an exception is thrown in this case)
*
*
* @see javax.jms.JMSConsumer#getMessageListener()
*/
void setMessageListener(MessageListener listener) throws JMSRuntimeException;
/** Receives the next message produced for this JMSConsumer
.
*
* This call blocks indefinitely until a message is produced
* or until this JMSConsumer
is closed.
*
*
If this receive
is done within a transaction, the
* JMSConsumer retains the message until the transaction commits.
*
* @return the next message produced for this JMSConsumer
, or
* null if this JMSConsumer
is concurrently closed
*
* @exception JMSRuntimeException if the JMS provider fails to receive the next
* message due to some internal error.
*
*/
Message receive();
/** Receives the next message that arrives within the specified
* timeout interval.
*
*
This call blocks until a message arrives, the
* timeout expires, or this JMSConsumer
is closed.
* A timeout
of zero never expires, and the call blocks
* indefinitely.
*
* @param timeout the timeout value (in milliseconds)
*
* @return the next message produced for this JMSConsumer
, or
* null if the timeout expires or this JMSConsumer
is concurrently
* closed
*
* @exception JMSRuntimeException if the JMS provider fails to receive the next
* message due to some internal error.
*/
Message receive(long timeout);
/** Receives the next message if one is immediately available.
*
* @return the next message produced for this JMSConsumer
, or
* null if one is not available
*
* @exception JMSRuntimeException if the JMS provider fails to receive the next
* message due to some internal error.
*/
Message receiveNoWait();
/**
* Closes the JMSConsumer
.
*
*
* Since a provider may allocate some resources on behalf of a
* JMSConsumer
outside the Java virtual machine,
* clients should close them when they are not needed. Relying on garbage
* collection to eventually reclaim these resources may not be timely
* enough.
*
*
* This call blocks until a receive
in progress has completed.
* A blocked message consumer receive
call returns null when
* this JMSConsumer
is closed.
*
* @exception JMSRuntimeException
* if the JMS provider fails to close the JMSConsumer
* due to some internal error.
*/
void close();
/**
* Receives the next message produced for this JMSConsumer
and
* returns its payload, which must be of the specified type
*
*
* This call blocks indefinitely until a message is produced or until this
* JMSConsumer
is closed.
*
*
* If this receivePayload
is done within a transaction, the
* JMSConsumer
retains the message until the transaction commits.
*
* @param c
* The class of the payload of the next message.
* If the next message is expected to be a TextMessage
then
* this should be set to String.class
.
* If the next message is expected to be a ObjectMessage
then
* this should be set to java.io.Serializable.class
.
* If the next message is expected to be a MapMessage
then this
* should be set to java.util.Map.class
.
* If the next message is expected to be a BytesMessage
then this
* should be set to java.util.Map.class
.
* If the next message is expected to be a BytesMessage
then this
* should be set to byte[].class
.
* If the next message is not of the expected type
* a ClassCastException
will be thrown
* and the message will not be delivered.
*
* @return the payload of the next message produced for this
* JMSConsumer
, or null if this JMSConsumer
is
* concurrently closed
*
* @throws JMSRuntimeException
* if the JMS provider fails to receive the next message due
* to some internal error
* @throws ClassCastException
* if the next message is not of the expected type
*/
T receivePayload(Class c);
/**
* Receives the next message produced for this JMSConsumer
and that
* arrives within the specified timeout period, and returns its payload,
* which must be of the specified type
*
*
* This call blocks until a message arrives, the timeout expires, or this
* JMSConsumer
is closed. A timeout of zero never expires, and the
* call blocks indefinitely.
*
*
* If this receivePayload
is done within a transaction, the
* JMSConsumer
retains the message until the transaction commits.
*
* @param c
* The class of the payload of the next message.
* If the next message is expected to be a TextMessage
then
* this should be set to String.class
.
* If the next message is expected to be a ObjectMessage
then
* this should be set to java.io.Serializable.class
.
* If the next message is expected to be a MapMessage
then this
* should be set to java.util.Map.class
.
* If the next message is expected to be a BytesMessage
then this
* should be set to java.util.Map.class
.
* If the next message is expected to be a BytesMessage
then this
* should be set to byte[].class
.
* If the next message is not of the expected type
* a ClassCastException
will be thrown
* and the message will not be delivered.
*
* @return the payload of the next message produced for this
* JMSConsumer
, or null if this JMSConsumer
is
* concurrently closed
*
* @throws JMSRuntimeException
* if the JMS provider fails to receive the next message due
* to some internal error
* @throws ClassCastException
* if the next message is not of the expected type
*/
T receivePayload(Class c, long timeout);
/**
* Receives the next message produced for this JMSConsumer
and
* returns its payload, which must be of the specified type
*
*
* Returns null if a message is not available.
*
*
* If this receivePayload
is done within a transaction, the
* JMSConsumer
retains the message until the transaction commits.
*
* @param c
* The class of the payload of the next message.
* If the next message is expected to be a TextMessage
then
* this should be set to String.class
.
* If the next message is expected to be a ObjectMessage
then
* this should be set to java.io.Serializable.class
.
* If the next message is expected to be a MapMessage
then this
* should be set to java.util.Map.class
.
* If the next message is expected to be a BytesMessage
then this
* should be set to java.util.Map.class
.
* If the next message is expected to be a BytesMessage
then this
* should be set to byte[].class
.
* If the next message is not of the expected type
* a ClassCastException
will be thrown
* and the message will not be delivered.
*
* @return the payload of the next message produced for this
* JMSConsumer
, or null if this JMSConsumer
is
* concurrently closed
*
* @throws JMSRuntimeException
* if the JMS provider fails to receive the next message due
* to some internal error
* @throws ClassCastException
* if the next message is not of the expected type
*/
T receivePayloadNoWait(Class c);
}