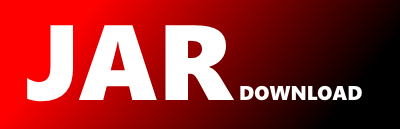
javax.jms.JMSProducer Maven / Gradle / Ivy
/*
* DO NOT ALTER OR REMOVE COPYRIGHT NOTICES OR THIS HEADER.
*
* Copyright (c) 2011-2012 Oracle and/or its affiliates. All rights reserved.
*
* The contents of this file are subject to the terms of either the GNU
* General Public License Version 2 only ("GPL") or the Common Development
* and Distribution License("CDDL") (collectively, the "License"). You
* may not use this file except in compliance with the License. You can
* obtain a copy of the License at
* https://glassfish.dev.java.net/public/CDDL+GPL_1_1.html
* or packager/legal/LICENSE.txt. See the License for the specific
* language governing permissions and limitations under the License.
*
* When distributing the software, include this License Header Notice in each
* file and include the License file at packager/legal/LICENSE.txt.
*
* GPL Classpath Exception:
* Oracle designates this particular file as subject to the "Classpath"
* exception as provided by Oracle in the GPL Version 2 section of the License
* file that accompanied this code.
*
* Modifications:
* If applicable, add the following below the License Header, with the fields
* enclosed by brackets [] replaced by your own identifying information:
* "Portions Copyright [year] [name of copyright owner]"
*
* Contributor(s):
* If you wish your version of this file to be governed by only the CDDL or
* only the GPL Version 2, indicate your decision by adding "[Contributor]
* elects to include this software in this distribution under the [CDDL or GPL
* Version 2] license." If you don't indicate a single choice of license, a
* recipient has the option to distribute your version of this file under
* either the CDDL, the GPL Version 2 or to extend the choice of license to
* its licensees as provided above. However, if you add GPL Version 2 code
* and therefore, elected the GPL Version 2 license, then the option applies
* only if the new code is made subject to such option by the copyright
* holder.
*/
package javax.jms;
import java.io.Serializable;
import java.util.Enumeration;
import java.util.Map;
/**
* A JMSProducer
is a simple object used to send messages on behalf
* of a JMSContext
. An instance of JMSProducer
is
* created by calling the createProducer
method on a
* JMSContext
. It provides various send
methods to
* send a message to a specified destination. It also provides methods to allow
* message send options, message properties and message headers to be specified
* prior to sending a message or set of messages.
*
* Message send options may be specified using one or more of the following
* methods: setDeliveryMode
, setPriority
,
* setTimeToLive
, setDeliveryDelay
,
* setDisableMessageTimestamp
, setDisableMessageID
and
* setAsync
.
*
* Message properties may be may be specified using one or more of nine
* setProperty
methods. Any message properties set using these
* methods will override any message properties that have been set directly on
* the message.
*
* Message headers may be specified using one or more of the following methods:
* setJMSCorrelationID
, setJMSCorrelationIDAsBytes
,
* setJMSType
or setJMSReplyTo
. Any message headers
* set using these methods will override any message headers that have been set
* directly on the message.
*
* All the above methods return the JMSProducer
to allow method
* calls to be chained together, allowing a fluid programming style. For
* example:
*
* context.createProducer().setDeliveryMode(DeliveryMode.NON_PERSISTENT).setTimeToLive(1000).send(destination, message);
*
* Instances of JMSProducer
are intended to be lightweight objects
* which can be created freely and which do not consume significant resources.
* This interface therefore does not provide a close
method.
*
* @version 2.0
* @since 2.0
*
*/
public interface JMSProducer {
/**
* Sends a message to the specified destination, using any send options,
* message properties and message headers that have been defined on this
* JMSProducer
.
*
* @param destination
* the destination to send this message to
* @param message
* the message to send
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to send the message due to some
* internal error.
* @throws MessageFormatRuntimeException
* if an invalid message is specified.
* @throws InvalidDestinationRuntimeException
* if a client uses this method with an invalid destination.
* @throws MessageNotWriteableException
* if this JMSProducer
has been configured to set a
* message property, but the message's properties are read-only
*/
JMSProducer send(Destination destination, Message message);
/**
* Send a TextMessage
with the specified payload to the
* specified destination, using any send options, message properties and
* message headers that have been defined on this JMSProducer
.
*
* @param destination
* the destination to send this message to
* @param payload
* the payload of the TextMessage
that will be sent.
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to send the message due to some
* internal error.
* @throws MessageFormatRuntimeException
* if an invalid message is specified.
* @throws InvalidDestinationRuntimeException
* if a client uses this method with an invalid destination.
*/
JMSProducer send(Destination destination, String payload);
/**
* Send a MapMessage
with the specified payload to the
* specified destination, using any send options, message properties and
* message headers that have been defined on this JMSProducer
.
*
* @param destination
* the destination to send this message to
* @param payload
* the payload of the MapMessage
that will be sent.
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to send the message due to some
* internal error.
* @throws MessageFormatRuntimeException
* if an invalid message is specified.
* @throws InvalidDestinationRuntimeException
* if a client uses this method with an invalid destination.
*/
JMSProducer send(Destination destination, Map payload);
/**
* Send a BytesMessage
with the specified payload to the
* specified destination, using any send options, message properties and
* message headers that have been defined on this JMSProducer
.
*
* @param destination
* the destination to send this message to
* @param payload
* the payload of the BytesMessage
that will be
* sent.
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to send the message due to some
* internal error.
* @throws MessageFormatRuntimeException
* if an invalid message is specified.
* @throws InvalidDestinationRuntimeException
* if a client uses this method with an invalid destination.
*/
JMSProducer send(Destination destination, byte[] payload);
/**
* Send an ObjectMessage
with the specified payload to the
* specified destination, using any send options, message properties and
* message headers that have been defined on this JMSProducer
.
*
* @param destination
* the destination to send this message to
* @param payload
* the payload of the ObjectMessage that will be sent.
* @return this JMSProducer
* @throws JMSRuntimeException
* if JMS provider fails to send the message due to some
* internal error.
* @throws MessageFormatRuntimeException
* if an invalid message is specified.
* @throws InvalidDestinationRuntimeException
* if a client uses this method with an invalid destination.
*/
JMSProducer send(Destination destination, Serializable payload);
/**
* Specifies whether message IDs may be disabled for messages that are sent
* using this JMSProducer
*
* Since message IDs take some effort to create and increase a message's
* size, some JMS providers may be able to optimise message overhead if they
* are given a hint that the message ID is not used by an application. By
* calling this method, a JMS application enables this potential
* optimisation for all messages sent using this JMSProducer
.
* If the JMS provider accepts this hint, these messages must have the
* message ID set to null; if the provider ignores the hint, the message ID
* must be set to its normal unique value.
*
* Message IDs are enabled by default.
*
*
* @param value
* indicates whether message IDs may be disabled
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set message ID to disabled due
* to some internal error.
*
* @see javax.jms.JMSProducer#getDisableMessageID
*/
JMSProducer setDisableMessageID(boolean value);
/**
* Gets an indication of whether message IDs are disabled.
*
* @return an indication of whether message IDs are disabled
*
* @throws JMSRuntimeException
* if the JMS provider fails to determine if message IDs are
* disabled due to some internal error.
*
* @see javax.jms.JMSProducer#setDisableMessageID
*/
boolean getDisableMessageID();
/**
* Specifies whether message timestamps may be disabled for messages that
* are sent using this JMSProducer
. Since timestamps take
* some effort to create and increase a message's size, some JMS providers
* may be able to optimise message overhead if they are given a hint that
* the timestamp is not used by an application. By calling this method, a
* JMS application enables this potential optimisation for all messages sent
* using this JMSProducer
. If the JMS provider accepts this
* hint, these messages must have the timestamp set to zero; if the provider
* ignores the hint, the timestamp must be set to its normal value.
*
* Message timestamps are enabled by default.
*
* @param value
* indicates whether message timestamps may be disabled
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set timestamps to disabled due
* to some internal error.
*
* @see javax.jms.JMSProducer#getDisableMessageTimestamp
*/
JMSProducer setDisableMessageTimestamp(boolean value);
/**
* Gets an indication of whether message timestamps are disabled.
*
* @return an indication of whether message timestamps are disabled
*
* @throws JMSRuntimeException
* if the JMS provider fails to determine if timestamps are
* disabled due to some internal error.
* @see javax.jms.JMSProducer#setDisableMessageTimestamp
*/
boolean getDisableMessageTimestamp();
/**
* Specifies the delivery mode of messages that are sent using this
* JMSProducer
*
* Delivery mode is set to PERSISTENT
by default.
*
* @param deliveryMode
* the message delivery mode to be used; legal values are
* DeliveryMode.NON_PERSISTENT
and
* DeliveryMode.PERSISTENT
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the delivery mode due to
* some internal error.
*
* @see javax.jms.JMSProducer#getDeliveryMode
* @see javax.jms.DeliveryMode#NON_PERSISTENT
* @see javax.jms.DeliveryMode#PERSISTENT
* @see javax.jms.Message#DEFAULT_DELIVERY_MODE
*/
JMSProducer setDeliveryMode(int deliveryMode);
/**
* Returns the delivery mode of messages that are sent using this
* JMSProducer
*
* @return the message delivery mode
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the delivery mode due to
* some internal error.
*
* @see javax.jms.JMSProducer#setDeliveryMode
*/
int getDeliveryMode();
/**
* Specifies the priority of messages that are sent using this
* JMSProducer
*
* The JMS API defines ten levels of priority value, with 0 as the lowest
* priority and 9 as the highest. Clients should consider priorities 0-4 as
* gradations of normal priority and priorities 5-9 as gradations of
* expedited priority. Priority is set to 4 by default.
*
*
* @param defaultPriority
* the message priority to be used; must be a value between 0 and
* 9
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the priority due to some
* internal error.
*
* @see javax.jms.JMSProducer#getPriority
* @see javax.jms.Message#DEFAULT_PRIORITY
*/
JMSProducer setPriority(int defaultPriority);
/**
* Return the priority of messages that are sent using this
* JMSProducer
*
*
* @return the message priority
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the priority due to some
* internal error.
*
* @see javax.jms.JMSProducer#setPriority
*/
int getPriority();
/**
* Specifies the time to live of messages that are sent using this
* JMSProducer
. This is used to determine the expiration time
* of a message.
*
* The expiration time of a message is the sum of the message's time to live
* and the time it is sent. For transacted sends, this is the time the
* client sends the message, not the time the transaction is committed.
*
* Clients should not receive messages that have expired; however, JMS does
* not guarantee that this will not happen.
*
* A JMS provider should do its best to accurately expire messages; however,
* JMS does not define the accuracy provided. It is not acceptable to simply
* ignore time-to-live.
*
* Time to live is set to zero by default, which means a message never
* expires.
*
* @param timeToLive
* the message time to live to be used, in milliseconds; a value
* of zero means that a message never expires.
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the time to live due to some
* internal error.
*
* @see javax.jms.JMSProducer#getTimeToLive
* @see javax.jms.Message#DEFAULT_TIME_TO_LIVE
*/
JMSProducer setTimeToLive(long timeToLive);
/**
* Returns the time to live of messages that are sent using this
* JMSProducer
.
*
* @return the message time to live in milliseconds; a value of zero means
* that a message never expires.
* @throws JMSRuntimeException
* if the JMS provider fails to get the time to live due to some
* internal error.
*
* @see javax.jms.JMSProducer#setTimeToLive
*/
long getTimeToLive();
/**
* Specifies the delivery delay of messages that are sent using this
* JMSProducer
. This is the minimum length of time in
* milliseconds from the time the message was sent before a produced message
* becomes visible on the target destination and available for delivery to
* consumers.
*
* Delivery delay is set to zero by default.
*
* @param deliveryDelay
* the delivery delay in milliseconds.
* @return this JMSProducer
*
* @throws JMSRuntimeException
* if the JMS provider fails to set the delivery delay due to
* some internal error.
*
* @see javax.jms.JMSProducer#getDeliveryDelay
* @see javax.jms.Message#DEFAULT_DELIVERY_DELAY
*/
JMSProducer setDeliveryDelay(long deliveryDelay);
/**
* Returns the delivery delay of messages that are sent using this
* JMSProducer
.
*
* @return the delivery delay in milliseconds.
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the delivery delay due to
* some internal error.
*
* @see javax.jms.JMSProducer#setDeliveryDelay
*/
long getDeliveryDelay();
/**
* Specifies whether subsequent calls to send
on this
* JMSProducer
object should be synchronous or asynchronous. If
* the specified CompletionListener
is not null then subsequent
* calls to send
will be asynchronous. If the specified
* CompletionListener
is null then subsequent calls to
* send
will be synchronous. Calls to send
are
* synchronous by default.
*
* If a call to send
is asynchronous then part of the work
* involved in sending the message will be performed in a separate thread
* and the specified CompletionListener will be notified when
* the operation has completed.
*
* When the message has been successfully sent the JMS provider invokes the
* callback method onCompletion on the CompletionListener
* object. Only when that callback has been invoked can the application be
* sure that the message has been successfully sent with the same degree of
* confidence as if the send had been synchronous. An application which
* requires this degree of confidence must therefore wait for the callback
* to be invoked before continuing.
*
* The following information is intended to give an indication of how an
* asynchronous send would typically be implemented.
*
* In some JMS providers, a normal synchronous send involves sending the
* message to a remote JMS server and then waiting for an acknowledgement to
* be received before returning. It is expected that such a provider would
* implement an asynchronous send by sending the message to the remote JMS
* server and then returning without waiting for an acknowledgement. When
* the acknowledgement is received, the JMS provider would notify the
* application by invoking the onCompletion method on the
* application-specified CompletionListener object. If for some
* reason the acknowledgement is not received the JMS provider would notify
* the application by invoking the CompletionListener's
* onException method.
*
* In those cases where the JMS specification permits a lower level of
* reliability, a normal synchronous send might not wait for an
* acknowledgement. In that case it is expected that an asynchronous send
* would be similar to a synchronous send: the JMS provider would send the
* message to the remote JMS server and then return without waiting for an
* acknowledgement. However the JMS provider would still notify the
* application that the send had completed by invoking the
* onCompletion method on the application-specified
* CompletionListener object.
*
* It is up to the JMS provider to decide exactly what is performed in the
* calling thread and what, if anything, is performed asynchronously, so
* long as it satisfies the requirements given below:
*
* Quality of service: After the send operation is complete, which
* means that the message has been successfully sent with the same degree of
* confidence as if a normal synchronous send had been performed, the JMS
* provider must invoke the CompletionListener. The
* CompletionListener must not be invoked earlier than this.
*
* Message order: If the same JMSContext is used to send
* multiple messages then JMS message ordering requirements must be
* satisfied. This applies even if a combination of synchronous and
* asynchronous sends has been performed. The application is not required to
* wait for an asynchronous send to complete before sending the next
* message.
*
* Close, commit or rollback: If the session is transacted (uses a
* local transaction) then when the JMSContext's commit or
* rollback method is called the JMS provider must block until any
* incomplete send operations have been completed and all callbacks have
* returned before performing the commit or rollback. If the close
* method is called on the JMSContext then the JMS provider must
* block until any incomplete send operations have been completed and all
* callbacks have returned before closing the object and returning.
*
* Restrictions on usage in Java EE An asynchronous send is not
* permitted in a Java EE EJB or web container. If the application component
* violates this restriction this method may throw a JMSRuntimeException.
*
* Message headers JMS defines a number of message header fields and
* message properties which must be set by the "JMS provider on send". If
* the send is asynchronous these fields and properties may be accessed on
* the sending client only after the CompletionListener has been
* invoked. If the CompletionListener's onException method
* is called then the state of these message header fields and properties is
* undefined.
*
* Restrictions on threading: Applications that perform an
* asynchronous send must confirm to the threading restrictions defined in
* JMS. This means that the session may be used by only one thread at a
* time.
*
* Setting a CompletionListener does not cause the session to be
* dedicated to the thread of control which calls the
* CompletionListener. The application thread may therefore
* continue to use the session after performing an asynchronous send.
* However the CompletionListener's callback methods must not use
* the session if an application thread might be using the session at the
* same time.
*
* Use of the CompletionListener by the JMS provider: A
* session will only invoke one CompletionListener callback method
* at a time. For a given JMSContext, callbacks will be performed
* in the same order as the corresponding calls to the send method.
*
* A JMS provider must not invoke the CompletionListener from the
* thread that is calling the send method.
*
* Restrictions on the use of the Message object: Applications which
* perform an asynchronous send must take account of the restriction that a
* Message object is designed to be accessed by one logical thread
* of control at a time and does not support concurrent use.
*
* After the send method has returned, the application must not
* attempt to read the headers, properties or payload of the
* Message object until the CompletionListener's
* onCompletion or onException method has been called.
* This is because the JMS provider may be modifying the Message
* object in another thread during this time. The JMS provider may throw an
* JMSException if the application attempts to access or modify the
* Message object after the send method has returned and
* before the CompletionListener has been invoked. If the JMS
* provider does not throw an exception then the behaviour is undefined.
*
* @param completionListener
* If asynchronous send behaviour is required, this should be set to a CompletionListener
to be notified when the send
* has completed. If synchronous send behaviour is required, this should be set to null
.
* @return this JMSProducer
*
* @throws JMSRuntimeException
* if an internal error occurs
*
* @see javax.jms.JMSProducer#getAsync
* @see javax.jms.CompletionListener
*
*/
JMSProducer setAsync(CompletionListener completionListener);
/**
* If subsequent calls to send
on this
* JMSProducer
object have been configured to be asynchronous
* then this method returns the CompletionListener
* that has previously been configured.
* If subsequent calls to send
have been configured to be synchronous
* then this method returns null
.
*
* @return the CompletionListener
or null
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the required information due
* to some internal error.
*
* @see javax.jms.JMSProducer#setAsync
*/
CompletionListener getAsync();
/**
* Specifies that messages sent using this JMSProducer
should
* have the specified property set to the specified boolean
* value.
*
* This will override any property of the same name that is already set on
* the message being sent.
*
* @param name
* the name of the property
* @param value
* the boolean
value to set
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the property due to some
* internal error.
* @throws IllegalArgumentException
* if the name is null or if the name is an empty string.
*
* @see javax.jms.JMSProducer#getBooleanProperty
*/
JMSProducer setProperty(String name, boolean value);
/**
* Specifies that messages sent using this JMSProducer
should
* have the specified property set to the specified byte
value.
*
* This will override any property of the same name that is already set on
* the message being sent.
*
* @param name
* the name of the property
* @param value
* the byte
value to set
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the property due to some
* internal error.
* @throws IllegalArgumentException
* if the name is null or if the name is an empty string.
*
* @see javax.jms.JMSProducer#getByteProperty
*/
JMSProducer setProperty(String name, byte value);
/**
* Specifies that messages sent using this JMSProducer
should
* have the specified property set to the specified short
* value.
*
* This will override any property of the same name that is already set on
* the message being sent.
*
* @param name
* the name of the property
* @param value
* the short
property value to set
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the property due to some
* internal error.
* @throws IllegalArgumentException
* if the name is null or if the name is an empty string.
*
* @see javax.jms.JMSProducer#getShortProperty
*/
JMSProducer setProperty(String name, short value);
/**
* Specifies that messages sent using this JMSProducer
should
* have the specified property set to the specified int
value.
*
* This will override any property of the same name that is already set on
* the message being sent.
*
* @param name
* the name of the property
* @param value
* the int
property value to set
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the property due to some
* internal error.
* @throws IllegalArgumentException
* if the name is null or if the name is an empty string.
*
* @see javax.jms.JMSProducer#getIntProperty
*/
JMSProducer setProperty(String name, int value);
/**
* Specifies that messages sent using this JMSProducer
should
* have the specified property set to the specified long
value.
*
* This will override any property of the same name that is already set on
* the message being sent.
*
* @param name
* the name of the property
* @param value
* the long
property value to set
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the property due to some
* internal error.
* @throws IllegalArgumentException
* if the name is null or if the name is an empty string.
*
* @see javax.jms.JMSProducer#getLongProperty
*/
JMSProducer setProperty(String name, long value);
/**
* Specifies that messages sent using this JMSProducer
should
* have the specified property set to the specified float
* value.
*
* This will override any property of the same name that is already set on
* the message being sent.
*
* @param name
* the name of the property
* @param value
* the float
property value to set
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the property due to some
* internal error.
* @throws IllegalArgumentException
* if the name is null or if the name is an empty string.
*
* @see javax.jms.JMSProducer#getFloatProperty
*/
JMSProducer setProperty(String name, float value);
/**
* Specifies that messages sent using this JMSProducer
should
* have the specified property set to the specified double
* value.
*
* This will override any property of the same name that is already set on
* the message being sent.
*
* @param name
* the name of the property
* @param value
* the double
property value to set
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the property due to some
* internal error.
* @throws IllegalArgumentException
* if the name is null or if the name is an empty string.
*
* @see javax.jms.JMSProducer#getDoubleProperty
*/
JMSProducer setProperty(String name, double value);
/**
* Specifies that messages sent using this JMSProducer
should
* have the specified property set to the specified String
* value.
*
* This will override any property of the same name that is already set on
* the message being sent.
*
* @param name
* the name of the property
* @param value
* the String
property value to set
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the property due to some
* internal error.
* @throws IllegalArgumentException
* if the name is null or if the name is an empty string.
*
* @see javax.jms.JMSProducer#getStringProperty
*/
JMSProducer setProperty(String name, String value);
/**
* Specifies that messages sent using this JMSProducer
should
* have the specified property set to the specified Java object value.
*
* Note that this method works only for the objectified primitive object
* types (Integer
, Double
, Long
...)
* and String
objects.
*
* This will override any property of the same name that is already set on
* the message being sent.
*
* @param name
* the name of the property
* @param value
* the Java object property value to set
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the property due to some
* internal error.
* @throws IllegalArgumentException
* if the name is null or if the name is an empty string.
* @throws MessageFormatRuntimeException
* if the object is invalid
*
* @see javax.jms.JMSProducer#getObjectProperty
*/
JMSProducer setProperty(String name, Object value);
/**
* Clears any message properties set on this JMSProducer
*
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to clear the message properties due
* to some internal error.
*/
JMSProducer clearProperties();
/**
* Indicates whether a message property with the specified name has been set
* on this JMSProducer
*
* @param name
* the name of the property
*
* @return true whether the property exists
*
* @throws JMSRuntimeException
* if the JMS provider fails to determine whether the property
* exists due to some internal error.
*/
boolean propertyExists(String name);
/**
* Returns the message property with the specified name that has been set on
* this JMSProducer
, converted to a boolean
.
*
* @param name
* the name of the property
*
* @return the property value, converted to a boolean
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the property value due to
* some internal error.
* @throws MessageFormatRuntimeException
* if this type conversion is invalid.
*
* @see javax.jms.JMSProducer#setProperty(java.lang.String,boolean)
*/
boolean getBooleanProperty(String name);
/**
* Returns the message property with the specified name that has been set on
* this JMSProducer
, converted to a String
.
*
* @param name
* the name of the property
*
* @return the property value, converted to a byte
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the property value due to
* some internal error.
* @throws MessageFormatRuntimeException
* if this type conversion is invalid.
*
* @see javax.jms.JMSProducer#setProperty(java.lang.String,byte)
*/
byte getByteProperty(String name);
/**
* Returns the message property with the specified name that has been set on
* this JMSProducer
, converted to a short
.
*
* @param name
* the name of the property
*
* @return the property value, converted to a short
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the property value due to
* some internal error.
* @throws MessageFormatRuntimeException
* if this type conversion is invalid.
*
* @see javax.jms.JMSProducer#setProperty(java.lang.String,short)
*/
short getShortProperty(String name);
/**
* Returns the message property with the specified name that has been set on
* this JMSProducer
, converted to a int
.
*
* @param name
* the name of the property
*
* @return the property value, converted to a int
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the property value due to
* some internal error.
* @throws MessageFormatRuntimeException
* if this type conversion is invalid.
*
* @see javax.jms.JMSProducer#setProperty(java.lang.String,int)
*/
int getIntProperty(String name);
/**
* Returns the message property with the specified name that has been set on
* this JMSProducer
, converted to a long
.
*
* @param name
* the name of the property
*
* @return the property value, converted to a long
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the property value due to
* some internal error.
* @throws MessageFormatRuntimeException
* if this type conversion is invalid.
*
* @see javax.jms.JMSProducer#setProperty(java.lang.String,long)
*/
long getLongProperty(String name);
/**
* Returns the message property with the specified name that has been set on
* this JMSProducer
, converted to a float
.
*
* @param name
* the name of the property
*
* @return the property value, converted to a float
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the property value due to
* some internal error.
* @throws MessageFormatRuntimeException
* if this type conversion is invalid.
*
* @see javax.jms.JMSProducer#setProperty(java.lang.String,float)
*/
float getFloatProperty(String name);
/**
* Returns the message property with the specified name that has been set on
* this JMSProducer
, converted to a double
.
*
* @param name
* the name of the property
*
* @return the property value, converted to a double
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the property value due to
* some internal error.
* @throws MessageFormatRuntimeException
* if this type conversion is invalid.
*
* @see javax.jms.JMSProducer#setProperty(java.lang.String,double)
*/
double getDoubleProperty(String name);
/**
* Returns the message property with the specified name that has been set on
* this JMSProducer
, converted to a String
.
*
* @param name
* the name of the property
*
* @return the property value, converted to a boolean
; if there
* is no property by this name, a null value is returned
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the property value due to
* some internal error.
* @throws MessageFormatRuntimeException
* if this type conversion is invalid.
*
* @see javax.jms.JMSProducer#setProperty(java.lang.String,String)
*/
String getStringProperty(String name);
/**
* Returns the message property with the specified name that has been set on
* this JMSProducer
, converted to objectified format.
*
* This method can be used to return, in objectified format, an object that
* has been stored as a property in the message with the equivalent
* setObjectProperty
method call, or its equivalent primitive
* settypeProperty
method.
*
* @param name
* the name of the property
*
* @return the Java object property value with the specified name, in
* objectified format (for example, if the property was set as an
* int
, an Integer
is returned); if there
* is no property by this name, a null value is returned
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the property value due to
* some internal error.
*
* @see javax.jms.JMSProducer#setProperty(java.lang.String,java.lang.Object)
*/
Object getObjectProperty(String name);
/**
* Returns an Enumeration
of the names of all the message
* properties that have been set on this JMSProducer
.
*
* Note that JMS standard header fields are not considered properties and
* are not returned in this enumeration.
*
* @return an enumeration of all the names of property values
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the property names due to
* some internal error.
*/
Enumeration getPropertyNames();
/**
* Specifies that messages sent using this JMSProducer
should
* have their JMSCorrelationID
header value set to the
* specified correlation ID, where correlation ID is specified as an array
* of bytes.
*
* This will override any JMSCorrelationID
header value that is
* already set on the message being sent.
*
* The array is copied before the method returns, so future modifications to
* the array will not alter the value in this JMSProducer
.
*
* If a provider supports the native concept of correlation ID, a JMS client
* may need to assign specific JMSCorrelationID
values to match
* those expected by native messaging clients. JMS providers without native
* correlation ID values are not required to support this method and its
* corresponding get method; their implementation may throw a
* java.lang.UnsupportedOperationException
.
*
* The use of a byte[]
value for JMSCorrelationID
* is non-portable.
*
* @param correlationID
* the correlation ID value as an array of bytes
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the correlation ID due to
* some internal error.
*
* @see javax.jms.JMSProducer#setJMSCorrelationID(String)
* @see javax.jms.JMSProducer#getJMSCorrelationID()
* @see javax.jms.JMSProducer#getJMSCorrelationIDAsBytes()
*/
JMSProducer setJMSCorrelationIDAsBytes(byte[] correlationID);
/**
* Returns the JMSCorrelationID
header value that has been set
* on this JMSProducer
, as an array of bytes.
*
* The use of a byte[]
value for JMSCorrelationID
* is non-portable.
*
* @return the correlation ID as an array of bytes
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the correlation ID due to
* some internal error.
*
* @see javax.jms.JMSProducer#setJMSCorrelationID(String)
* @see javax.jms.JMSProducer#getJMSCorrelationID()
* @see javax.jms.JMSProducer#setJMSCorrelationIDAsBytes(byte[])
*/
byte[] getJMSCorrelationIDAsBytes();
/**
* Specifies that messages sent using this JMSProducer
should
* have their JMSCorrelationID
header value set to the
* specified correlation ID, where correlation ID is specified as a
* String
.
*
* This will override any JMSCorrelationID
header value that is
* already set on the message being sent.
*
* A client can use the JMSCorrelationID
header field to link
* one message with another. A typical use is to link a response message
* with its request message.
*
* JMSCorrelationID
can hold one of the following:
*
* - A provider-specific message ID
*
- An application-specific
String
* - A provider-native
byte[]
value
*
*
* Since each message sent by a JMS provider is assigned a message ID value,
* it is convenient to link messages via message ID. All message ID values
* must start with the 'ID:'
prefix.
*
* In some cases, an application (made up of several clients) needs to use
* an application-specific value for linking messages. For instance, an
* application may use JMSCorrelationID
to hold a value
* referencing some external information. Application-specified values must
* not start with the 'ID:'
prefix; this is reserved for
* provider-generated message ID values.
*
* If a provider supports the native concept of correlation ID, a JMS client
* may need to assign specific JMSCorrelationID
values to match
* those expected by clients that do not use the JMS API. A
* byte[]
value is used for this purpose. JMS providers without
* native correlation ID values are not required to support
* byte[]
values. The use of a byte[]
value for
* JMSCorrelationID
is non-portable.
*
* @param correlationID
* the message ID of a message being referred to
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the correlation ID due to
* some internal error.
*
* @see javax.jms.JMSProducer#getJMSCorrelationID()
* @see javax.jms.JMSProducer#getJMSCorrelationIDAsBytes()
* @see javax.jms.JMSProducer#setJMSCorrelationIDAsBytes(byte[])
*/
JMSProducer setJMSCorrelationID(String correlationID);
/**
* Returns the JMSCorrelationID
header value that has been set
* on this JMSProducer
, as a String
.
*
* This method is used to return correlation ID values that are either
* provider-specific message IDs or application-specific String
* values.
*
* @return the correlation ID of a message as a String
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the correlation ID due to
* some internal error.
*
* @see javax.jms.JMSProducer#setJMSCorrelationID(String)
* @see javax.jms.JMSProducer#getJMSCorrelationIDAsBytes()
* @see javax.jms.JMSProducer#setJMSCorrelationIDAsBytes(byte[])
*/
String getJMSCorrelationID();
/**
* Specifies that messages sent using this JMSProducer
should
* have their JMSType
header value set to the specified message
* type.
*
* This will override any JMSType
header value that is already
* set on the message being sent.
*
* Some JMS providers use a message repository that contains the definitions
* of messages sent by applications. The JMSType
header field
* may reference a message's definition in the provider's repository.
*
* The JMS API does not define a standard message definition repository, nor
* does it define a naming policy for the definitions it contains.
*
* Some messaging systems require that a message type definition for each
* application message be created and that each message specify its type. In
* order to work with such JMS providers, JMS clients should assign a value
* to JMSType
, whether the application makes use of it or not.
* This ensures that the field is properly set for those providers that
* require it.
*
* To ensure portability, JMS clients should use symbolic values for
* JMSType
that can be configured at installation time to the
* values defined in the current provider's message repository. If string
* literals are used, they may not be valid type names for some JMS
* providers.
*
* @param type
* the message type
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the message type due to some
* internal error.
*
* @see javax.jms.JMSProducer#getJMSType()
*/
JMSProducer setJMSType(String type);
/**
* Returns the JMSType
header value that has been set on this
* JMSProducer
.
*
* @return the message type
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the message type due to some
* internal error.
*
* @see javax.jms.JMSProducer#setJMSType(String)
*/
String getJMSType();
/**
* Specifies that messages sent using this JMSProducer
should
* have their JMSReplyTo
header value set to the specified
* Destination
object.
*
* This will override any JMSReplyTo
header value that is
* already set on the message being sent.
*
* The JMSReplyTo
header field contains the destination where a
* reply to the current message should be sent. If it is null, no reply is
* expected. The destination may be either a Queue
object or a
* Topic
object.
*
* Messages sent with a null JMSReplyTo
value may be a
* notification of some event, or they may just be some data the sender
* thinks is of interest.
*
* Messages with a JMSReplyTo
value typically expect a
* response. A response is optional; it is up to the client to decide. These
* messages are called requests. A message sent in response to a request is
* called a reply.
*
* In some cases a client may wish to match a request it sent earlier with a
* reply it has just received. The client can use the
* JMSCorrelationID
header field for this purpose.
*
* @param replyTo
* Destination
to which to send a response to this
* message
* @return this JMSProducer
* @throws JMSRuntimeException
* if the JMS provider fails to set the JMSReplyTo
* destination due to some internal error.
*
* @see javax.jms.JMSProducer#getJMSReplyTo()
*/
JMSProducer setJMSReplyTo(Destination replyTo);
/**
* Returns the JMSReplyTo
header value that has been set on
* this JMSProducer
.
*
*
* @return Destination
the JMSReplyTo
header value
*
* @throws JMSRuntimeException
* if the JMS provider fails to get the JMSReplyTo
* destination due to some internal error.
*
* @see javax.jms.JMSProducer#setJMSReplyTo(Destination)
*/
Destination getJMSReplyTo();
}