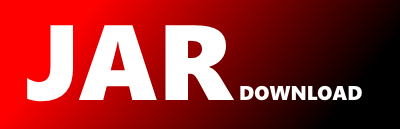
javax.measure.Quantity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of unit-api Show documentation
Show all versions of unit-api Show documentation
Units of Measurement Standard - This JSR specifies Java packages for modeling and working with measurement values, quantities and their corresponding units.
/*
* Units of Measurement API
* Copyright (c) 2014-2016, Jean-Marie Dautelle, Werner Keil, V2COM.
*
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification,
* are permitted provided that the following conditions are met:
*
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
*
* 2. Redistributions in binary form must reproduce the above copyright notice, this list of conditions
* and the following disclaimer in the documentation and/or other materials provided with the distribution.
*
* 3. Neither the name of JSR-363 nor the names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO,
* THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE
* ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED
* AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE,
* EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package javax.measure;
/**
* Represents a quantitative property of a phenomenon, body, or substance, that can be quantified by measurement. {@link javax.measure.quantity.Mass
* Mass}, time, distance, heat, and angular separation are among the familiar examples of quantitative properties.
*
* {@literal Unit} pound = ... {@literal Quantity} size = ... {@literal Sensor}
* thermometer = ... {@literal Vector3D} aircraftSpeed = ...
*
*
* @apiNote This interface places no restrictions on the mutability of implementations, however immutability is strongly recommended. All
* implementations must be {@link Comparable}.
*
* @param
* The type of the quantity.
*
* @author Jean-Marie Dautelle
* @author Martin Desruisseaux
* @author Werner Keil
* @author Otavio Santana
* @see Wikipedia: Quantity
* @see Martin Fowler - Quantity
* @see Wikipedia: Conversion of units
* @version 0.26, Date: 2015-12-23
*/
public interface Quantity> {
/**
* Returns the sum of this {@code Quantity} with the one specified.
*
* @param augend
* the {@code Quantity} to be added.
* @return {@code this + augend}.
*/
Quantity add(Quantity augend);
/**
* Returns the difference between this {@code Quantity} and the one specified.
*
* @param subtrahend
* the {@code Quantity} to be subtracted.
* @return this - that
.
*/
Quantity subtract(Quantity subtrahend);
/**
* Returns the product of this {@code Quantity} divided by the {@code Quantity} specified.
*
* @throws ClassCastException
* if the type of an element in the specified operation is incompatible with this quantity (optional)
*
* @param divisor
* the {@code Quantity} divisor.
* @return this / that
.
*/
Quantity> divide(Quantity> divisor);
/**
* Returns the product of this {@code Quantity} divided by the {@code Number} specified.
*
* @param divisor
* the {@code Number} divisor.
* @return this / that
.
*/
Quantity divide(Number divisor);
/**
* Returns the product of this {@code Quantity} with the one specified.
*
* @throws ClassCastException
* if the type of an element in the specified operation is incompatible with this quantity (optional)
*
* @param multiplier
* the {@code Quantity} multiplier.
* @return this * multiplier
.
*/
Quantity> multiply(Quantity> multiplier);
/**
* Returns the product of this {@code Quantity} with the {@code Number} value specified.
*
* @param multiplier
* the {@code Number} multiplier.
* @return this * multiplier
.
*/
Quantity multiply(Number multiplier);
/**
* Returns a {@code Quantity} whose unit is {@code unit.inverse()}.
*
* @return {@code Quantity with this.getUnit().inverse()}.
*/
Quantity> inverse();
/**
* Returns this {@code Quantity} converted into another (compatible) {@code Unit}.
*
* @param unit
* the {@code Unit} to convert to.
* @return the converted result.
*/
Quantity to(Unit unit);
/**
* Casts this quantity to a parameterized unit of specified nature or throw a ClassCastException
if the dimension of the specified
* quantity and this measure unit's dimension do not match. For example:
*
*
* {@literal Quantity} length = Quantities.getQuantity("2 km").asType(Length.class);
*
or
* {@literal Quantity} C = length.multiply(299792458).divide(second).asType(Speed.class);
*
*
*
* @param
* The type of the quantity.
* @param type
* the quantity class identifying the nature of the quantity.
* @return this quantity parameterized with the specified type.
* @throws ClassCastException
* if the dimension of this unit is different from the specified quantity dimension.
* @throws UnsupportedOperationException
* if the specified quantity class does not have a SI unit for the quantity.
* @see Unit#asType(Class)
*/
> Quantity asType(Class type) throws ClassCastException;
/**
* Returns the value of this {@code Quantity}.
*
* @return a value.
*/
Number getValue();
/**
* Returns the unit of this {@code Quantity}.
*
* @return the unit (shall not be {@code null}).
*/
Unit getUnit();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy