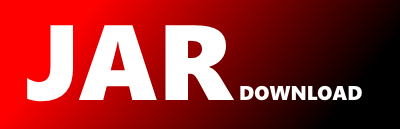
javax.slee.facilities.AlarmFacility Maven / Gradle / Ivy
package javax.slee.facilities;
import javax.slee.ComponentID;
import javax.slee.UnrecognizedComponentException;
/**
* The Alarm Facility is used to indicate to the SLEE requests to raise or clear
* alarms. If a request is made to raise an alarm and the identified alarm has not
* already been raised, the alarm is raised and a corresponding alarm notification
* is generated by the {@link javax.slee.management.AlarmMBean}. If a request is
* made to clear an alarm and the identified alarm is currently raised, the alarm
* is cleared and a corresponding alarm notification is generated by the
* {@link javax.slee.management.AlarmMBean}. Requests to raise an alarm that is
* already raised or to clear an alarm that is not currently raised cause no
* further action in the SLEE, ie. notifications are not generated in this case.
*
* Each AlarmFacility
object is associated with a particular
* {@link javax.slee.management.NotificationSource}, an object that identifies the SLEE
* component or subsystem that the alarm facility belongs to. For example, the
* AlarmFacility
object that a particular SBB obtains from its JNDI environment
* is associated with a notification source that identifies the SBB that that JNDI
* environment was created for and the service that that SBB belongs to.
*
* Each occurrence of an alarm is uniquely identified by an alarm identifier generated
* by the SLEE. Each alarm identifier is associated to an alarm with three identifying
* attributes:
*
* - the
NotificationSource
object associated to the AlarmFacility
* where the alarm was raised. This attribute is implicitly provided by the
* alarm facility when the alarm is raised.
* - an alarm type, specified by the notification source that raised the alarm.
* This attribute identifies the particular type of alarm, e.g. a database
* connection failure.
*
- an instance ID, specified by the notification source that raised the alarm.
* This attribute identifies a particular instance of the alarm type, e.g.
* the name of the host where connection failures are being experienced.
*
*
* All operations invoked on the AlarmFacility
interface only apply
* to alarms belonging to the notification source associated with the AlarmFacility
* object.
*
* The Alarm Facility is non-transactional. The effects of operations invoked on this
* facility occur immediately regardless of the state or outcome of any enclosing
* transaction.
*
* An SBB or profile object obtains access to an AlarmFacility
object via
* its JNDI environment. The Alarm Facility is bound into JNDI using the name specified
* by {@link #JNDI_NAME}. A resource adaptor entity obtains access to an
* AlarmFacility
object via the {@link javax.slee.resource.ResourceAdaptorContext}.
*
* @see javax.slee.management.AlarmMBean
* @see javax.slee.management.AlarmNotification
*/
public interface AlarmFacility {
/**
* Constant declaring the JNDI name where an AlarmFacility
object
* is bound into an SBB, profile object's, or resource adaptor object's JNDI
* environment.
*
* The value of this constant is "java:comp/env/slee/facilities/alarm".
* @since SLEE 1.1
*/
public static final String JNDI_NAME = "java:comp/env/slee/facilities/alarm";
/**
* Emit an alarm notification containing the specified alarm message.
*
* This method is a non-transactional method.
* @param alarmSource the identifier of the component that is emitting the alarm.
* @param alarmLevel the level of the alarm.
* @param alarmType a string denoting the type of the alarm. Refer to the
* SLEE specification for recommended formatting of alarm type strings.
* @param message the alarm message.
* @param timestamp the time (in ms since January 1, 1970 UTC) that the alarm was generated.
* @throws NullPointerException if any parameter is null
.
* @throws IllegalArgumentException if alarmLevel ==
{@link Level#OFF}.
* @throws UnrecognizedComponentException if alarmSource
is not a recognizable
* ComponentID
object for the SLEE or it does not correspond
* with a component installed in the SLEE.
* @throws FacilityException if the alarm could not be generated due to a system-level failure.
* @deprecated This method uses a ComponentID
to identify a notification
* source and thus is not compatible with the changes made to the alarm subsystem
* in SLEE 1.1. In addition the levels provided by the {@link Level} class were
* not practical for stateful alarm use. This method has been replaced with
* {@link #raiseAlarm(String,String,AlarmLevel,String)}.
*/
public void createAlarm(ComponentID alarmSource, Level alarmLevel, String alarmType, String message, long timestamp)
throws NullPointerException, IllegalArgumentException, UnrecognizedComponentException, FacilityException;
/**
* Emit an alarm notification containing the specified alarm message and cause throwable.
*
* This method is a non-transactional method.
* @param alarmSource the identifier of the component that is emitting the alarm.
* @param alarmLevel the level of the alarm.
* @param alarmType a string denoting the type of the alarm. Refer to the
* SLEE specification for recommended formatting of alarm type strings.
* @param message the alarm message.
* @param cause the reason (if any) this alarm was generated.
* @param timestamp the time (in ms since January 1, 1970 UTC) that the alarm was generated.
* @throws NullPointerException if any parameter is null
.
* @throws IllegalArgumentException if alarmLevel ==
{@link Level#OFF}.
* @throws UnrecognizedComponentException if alarmSource
is not a recognizable
* ComponentID
object for the SLEE or it does not correspond
* with a component installed in the SLEE.
* @throws FacilityException if the alarm could not be generated due to a system-level failure.
* @deprecated This method uses a ComponentID
to identify a notification
* source and thus is not compatible with the changes made to the alarm subsystem
* in SLEE 1.1. In addition the levels provided by the {@link Level} class were
* not practical for stateful alarm use. This method has been replaced with
* {@link #raiseAlarm(String,String,AlarmLevel,String,Throwable)}.
*/
public void createAlarm(ComponentID alarmSource, Level alarmLevel, String alarmType, String message, Throwable cause, long timestamp)
throws NullPointerException, IllegalArgumentException, UnrecognizedComponentException, FacilityException;
/**
* Request that an alarm be raised with the specified message if an alarm with
* the same identifying attributes is not currently active. The alarm will
* remain active until it is cleared, either via a call to any of the
* clearAlarm
methods in this interface, or by a management client
* interacting with an {@link javax.slee.management.AlarmMBean} object.
*
* If an alarm with the same identifying attributes (notification source, alarm
* type, and instance ID) is already active in the SLEE, this method has no
* effect and the alarm identifier of the existing active alarm is returned.
* If no such alarm exists, the alarm is raised, the SLEE's AlarmMBean
* object emits an {@link javax.slee.management.AlarmNotification}, and a
* SLEE-generated alarm identifier for the new alarm is returned.
*
* This method is equivalent to:
*
{@link #raiseAlarm(String,String,AlarmLevel,String,Throwable) raiseAlarm(alarmType, instanceID, alarmLevel, message, null);}
*
* This method is a non-transactional method.
* @param alarmType an identifier specifying the type of the alarm.
* @param instanceID an identifier specifying the particular instance of the
* alarm type that is occurring.
* @param alarmLevel the level of the alarm. The level cannot be {@link AlarmLevel#CLEAR}.
* @param message the message to include in the alarm if the alarm is raised
* as a result of this method call.
* @return a unique alarm identifier for the alarm. If the alarm was raised as
* a result of this operation, a new alarm identifier is generated by the
* SLEE and returned from this method. Otherwise the alarm identifier
* which identifies the existing alarm with the same identifying attributes
* is returned.
* @throws NullPointerException if any argument is null
.
* @throws IllegalArgumentException if alarmLevel
is {@link AlarmLevel#CLEAR}.
* @throws FacilityException if the alarm could not be raised due to a system-level failure.
* @since SLEE 1.1
*/
public String raiseAlarm(String alarmType, String instanceID, AlarmLevel alarmLevel, String message)
throws NullPointerException, IllegalArgumentException, FacilityException;
/**
* Request that an alarm be raised with the specified message and cause throwable
* if an alarm with the same identifying attributes is not currently active. The
* alarm will remain active until it is cleared, either via a call to any of the
* clearAlarm
methods in this interface, or by a management client
* interacting with an {@link javax.slee.management.AlarmMBean} object.
*
* If an alarm with the same identifying attributes (notification source, alarm
* type, and instance ID) is already active in the SLEE, this method has no
* effect and the alarm identifier of the existing active alarm is returned.
* If no such alarm exists, the alarm is raised, the SLEE's AlarmMBean
* object emits an {@link javax.slee.management.AlarmNotification}, and a
* SLEE-generated alarm identifier for the new alarm is returned.
*
* This method is a non-transactional method.
* @param alarmType an identifier specifying the type of the alarm.
* @param instanceID an identifier specifying the particular instance of the
* alarm type that is occurring.
* @param alarmLevel the level of the alarm. The level cannot be {@link AlarmLevel#CLEAR}.
* @param message the message to include in the alarm if the alarm is raised
* as a result of this method call.
* @param cause an optional Throwable
object that caused this alarm
* to be raised, included in the alarm descriptor if the alarm is raised
* as a result of this method call.
* @return a unique alarm identifier for the alarm. If the alarm was raised as
* a result of this operation, a new alarm identifier is generated by the
* SLEE and returned from this method. Otherwise the alarm identifier
* which identifies the existing alarm with the same identifying attributes
* is returned.
* @throws NullPointerException if alarmType
, instanceID
,
* alarmLevel
, or message
is null
.
* @throws IllegalArgumentException if alarmLevel
is {@link AlarmLevel#CLEAR}.
* @throws FacilityException if the alarm could not be raised due to a system-level failure.
* @since SLEE 1.1
*/
public String raiseAlarm(String alarmType, String instanceID, AlarmLevel alarmLevel, String message, Throwable cause)
throws NullPointerException, IllegalArgumentException, FacilityException;
/**
* Request that the alarm with the specified alarm identifier be cleared. If
* the alarm has already been cleared, or no such alarm exists in the SLEE, this
* method returns with no effect.
*
* This method only affects alarms belonging to the notification source associated
* with the AlarmFacility
object. If the specified alarm is cleared,
* the SLEE's AlarmMBean
object emits an {@link javax.slee.management.AlarmNotification}
* for the alarm with the alarm level in the notification set to {@link AlarmLevel#CLEAR}.
*
* This method is a non-transactional method.
* @param alarmID the unique alarm identifier for the alarm.
* @return true
if the specified alarm existed and was cleared as a
* result of this method, false
otherwise.
* @throws NullPointerException if alarmID
is null
.
* @throws FacilityException if the alarm could not be cleared due to a system-level
* failure.
* @since SLEE 1.1
*/
public boolean clearAlarm(String alarmID)
throws NullPointerException, FacilityException;
/**
* Request that all alarms of the specified type be cleared. If no active alarms
* of the specified type are found, this method returns with no effect.
*
* This method only affects alarms belonging to the notification source associated
* with the AlarmFacility
object. If one or more alarms are cleared
* by this method, the SLEE's AlarmMBean
object emits an
* {@link javax.slee.management.AlarmNotification} for each alarm with the alarm
* level in each notification set to {@link AlarmLevel#CLEAR}.
*
* This method is a non-transactional method.
* @param alarmType the type of the alarms to clear.
* @return the number of alarms cleared by this method call. May be zero if
* no alarms were cleared.
* @throws NullPointerException if alarmType
is null
.
* @throws FacilityException if the alarms could not be cleared due to a system-level
* failure.
* @since SLEE 1.1
*/
public int clearAlarms(String alarmType)
throws NullPointerException, FacilityException;
/**
* Request that all alarms belonging to the notification source associated with
* the AlarmFacility
object be cleared.
*
* This method only affects alarms belonging to the notification source associated
* with the AlarmFacility
object instance. If one or more alarms are
* cleared by this method, the SLEE's AlarmMBean
object emits an
* {@link javax.slee.management.AlarmNotification} for each alarm with the alarm
* level in each notification set to {@link AlarmLevel#CLEAR}.
*
* This method is a non-transactional method.
* @return the number of alarms cleared by this method call. May be zero if
* no alarms were cleared.
* @throws FacilityException if the alarms could not be cleared due to a system-level
* failure.
* @since SLEE 1.1
*/
public int clearAlarms()
throws FacilityException;
}