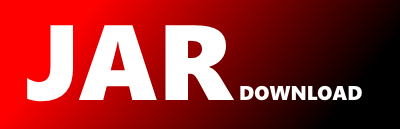
javax.slee.facilities.Level Maven / Gradle / Ivy
package javax.slee.facilities;
import java.io.Serializable;
import java.io.StreamCorruptedException;
/**
* This class defines an enumerated type for the alarm and trace levels supported by
* the Alarm Facility and Trace Facility. The class is based on the Java Logging API
* included with J2SE 1.4.
*
* A singleton instance of each enumerated value is guaranteed (via an implementation
* of readResolve()
- refer {@link java.io.Serializable java.io.Serializable}),
* so that equality tests using ==
are always evaluated correctly. (This
* equality test is only guaranteed if this class is loaded in the application's boot class
* path, rather than dynamically loaded at runtime.)
*
* The levels in descending order are:
*
* - SEVERE (highest value)
*
- WARNING
*
- INFO
*
- CONFIG
*
- FINE
*
- FINER
*
- FINEST (lowest value)
*
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public final class Level implements Serializable {
/**
* An integer representation of {@link #OFF}.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final int LEVEL_OFF = 0;
/**
* An integer representation of {@link #SEVERE}.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final int LEVEL_SEVERE = 1;
/**
* An integer representation of {@link #WARNING}.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final int LEVEL_WARNING = 2;
/**
* An integer representation of {@link #INFO}.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final int LEVEL_INFO = 3;
/**
* An integer representation of {@link #CONFIG}.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final int LEVEL_CONFIG = 4;
/**
* An integer representation of {@link #FINE}.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final int LEVEL_FINE = 5;
/**
* An integer representation of {@link #FINER}.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final int LEVEL_FINER = 6;
/**
* An integer representation of {@link #FINEST}.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final int LEVEL_FINEST = 7;
/**
* Level for maximum filtering. Notifications cannot be generated using this level.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final Level OFF = new Level(LEVEL_OFF);
/**
* Level for messages indicating a severe failure.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final Level SEVERE = new Level(LEVEL_SEVERE);
/**
* Level for messages indicating warning conditions.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final Level WARNING = new Level(LEVEL_WARNING);
/**
* Level for information messages.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final Level INFO = new Level(LEVEL_INFO);
/**
* Level for configuration messages.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final Level CONFIG = new Level(LEVEL_CONFIG);
/**
* Level for basic debug messages.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final Level FINE = new Level(LEVEL_FINE);
/**
* Level for moderately detailed messages.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final Level FINER = new Level(LEVEL_FINER);
/**
* Level for highly detailed messages. This is the lowest level.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static final Level FINEST = new Level(LEVEL_FINEST);
/**
* Get an Level
object from an integer value.
* @param level the level as an integer.
* @return an Level
object corresponding to level
.
* @throws IllegalArgumentException if level
is not a valid
* level value.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public static Level fromInt(int level) throws IllegalArgumentException {
switch (level) {
case LEVEL_OFF: return OFF;
case LEVEL_SEVERE: return SEVERE;
case LEVEL_WARNING: return WARNING;
case LEVEL_INFO: return INFO;
case LEVEL_CONFIG: return CONFIG;
case LEVEL_FINE: return FINE;
case LEVEL_FINER: return FINER;
case LEVEL_FINEST: return FINEST;
default: throw new IllegalArgumentException("Invalid level: " + level);
}
}
/**
* Get an integer value representation for this Level
object.
* @return an integer value representation for this Level
object.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public int toInt() {
return level;
}
/**
* Determine if this Level object represents the OFF level.
*
* This method is effectively equivalent to the conditional test:
* (this == OFF)
, ie. the code:
*
* if (level.isOff()) ...
*
* is interchangeable with the code:
*
* if (level == Level.OFF) ...
*
* @return true
if this object represents the OFF level,
* false
otherwise.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public boolean isOff() {
return level == LEVEL_OFF;
}
/**
* Determine if this Level object represents the SEVERE level.
*
* This method is effectively equivalent to the conditional test:
* (this == SEVERE)
, ie. the code:
*
* if (level.isSevere()) ...
*
* is interchangeable with the code:
*
* if (level == Level.SEVERE) ...
*
* @return true
if this object represents the SEVERE level,
* false
otherwise.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public boolean isSevere() {
return level == LEVEL_SEVERE;
}
/**
* Determine if this Level object represents the WARNING level.
*
* This method is effectively equivalent to the conditional test:
* (this == WARNING)
, ie. the code:
*
* if (level.isWarning()) ...
*
* is interchangeable with the code:
*
* if (level == Level.WARNING) ...
*
* @return true
if this object represents the WARNING level,
* false
otherwise.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public boolean isWarning() {
return level == LEVEL_WARNING;
}
/**
* Determine if this Level object represents the INFO level.
*
* This method is effectively equivalent to the conditional test:
* (this == INFO)
, ie. the code:
*
* if (level.isMinor()) ...
*
* is interchangeable with the code:
*
* if (level == Level.INFO) ...
*
* @return true
if this object represents the INFO level,
* false
otherwise.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public boolean isMinor() {
return level == LEVEL_INFO;
}
/**
* Determine if this Level object represents the CONFIG level.
*
* This method is effectively equivalent to the conditional test:
* (this == CONFIG)
, ie. the code:
*
* if (level.isConfig()) ...
*
* is interchangeable with the code:
*
* if (level == Level.CONFIG) ...
*
* @return true
if this object represents the CONFIG level,
* false
otherwise.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public boolean isConfig() {
return level == LEVEL_CONFIG;
}
/**
* Determine if this Level object represents the FINE level.
*
* This method is effectively equivalent to the conditional test:
* (this == FINE)
, ie. the code:
*
* if (level.isFine()) ...
*
* is interchangeable with the code:
*
* if (level == Level.FINE) ...
*
* @return true
if this object represents the FINE level,
* false
otherwise.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public boolean isFine() {
return level == LEVEL_FINE;
}
/**
* Determine if this Level object represents the FINER level.
*
* This method is effectively equivalent to the conditional test:
* (this == FINER)
, ie. the code:
*
* if (level.isFiner()) ...
*
* is interchangeable with the code:
*
* if (level == Level.FINER) ...
*
* @return true
if this object represents the FINER level,
* false
otherwise.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public boolean isFiner() {
return level == LEVEL_FINER;
}
/**
* Determine if this Level object represents the FINEST level.
*
* This method is effectively equivalent to the conditional test:
* (this == FINEST)
, ie. the code:
*
* if (level.isFinest()) ...
*
* is interchangeable with the code:
*
* if (level == Level.FINEST) ...
*
* @return true
if this object represents the FINEST level,
* false
otherwise.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public boolean isFinest() {
return level == LEVEL_FINEST;
}
/**
* Determine if this Level object represents a level that is higher or more severe
* that some other Level object. For the purposes of the comparison OFF
* is considered a higher level than SEVERE.
* @param other the Level
object to compare this with.
* @return true
if the level represented by this Level
* object is a higher level than the level represented by the specified
* Level
object, false
otherwise.
* @throws NullPointerException if other
is null
.
* @deprecated Trace and alarm levels now defined in {@link TraceLevel} and {@link AlarmLevel}.
*/
public boolean isHigherLevel(Level other) throws NullPointerException {
if (other == null) throw new NullPointerException("other is null");
return this.level < other.level;
}
/**
* Compare this level for equality with another.
* @param obj the object to compare this with.
* @return true
if obj
is an instance of this class
* representing the same level as this, false
otherwise.
*/
public boolean equals(Object obj) {
if (obj == this) return true;
return (obj instanceof Level) && ((Level)obj).level == level;
}
/**
* Get a hash code value for this level.
* @return a hash code value.
*/
public int hashCode() {
return level;
}
/**
* Get the textual representation of the Level object.
* @return the textual representation of the Level object.
*/
public String toString() {
switch (level) {
case LEVEL_OFF: return "Off";
case LEVEL_SEVERE: return "Severe";
case LEVEL_WARNING: return "Warning";
case LEVEL_INFO: return "Info";
case LEVEL_CONFIG: return "Config";
case LEVEL_FINE: return "Fine";
case LEVEL_FINER: return "Finer";
case LEVEL_FINEST: return "Finest";
default: return "Level in Unknown and Invalid State";
}
}
/**
* Private constructor to prevent unauthorized object creation.
*/
private Level(int level) {
this.level = level;
}
/**
* Resolve deserialisation references so that the singleton property of each
* enumerated object is preserved.
*/
private Object readResolve() throws StreamCorruptedException {
if (level == LEVEL_OFF) return OFF;
if (level == LEVEL_SEVERE) return SEVERE;
if (level == LEVEL_WARNING) return WARNING;
if (level == LEVEL_INFO) return INFO;
if (level == LEVEL_CONFIG) return CONFIG;
if (level == LEVEL_FINE) return FINE;
if (level == LEVEL_FINER) return FINER;
if (level == LEVEL_FINEST) return FINEST;
throw new StreamCorruptedException("Invalid internal state found");
}
/**
* The internal state representation of the enumerated type.
*/
private final int level;
}