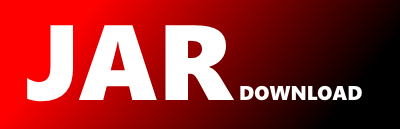
javax.slee.facilities.TraceFacility Maven / Gradle / Ivy
package javax.slee.facilities;
import javax.slee.ComponentID;
import javax.slee.UnrecognizedComponentException;
/**
* The Trace Facility is used by SBBs (and other components as determined by
* the SLEE vendor) to generate trace notifications intended for consumption by
* management clients external to the SLEE. For example, an SCE may attach a trace
* listener in order to deliver trace message output to the Service developer.
* Management clients register register interest in receiving trace notifictions using
* the SLEE's {@link javax.slee.management.TraceMBean} object.
*
* The Trace Facility is non-transactional. The effects of operations invoked on this
* facility occur regardless of the state or outcome of any enclosing transaction.
*
*
* - SBB JNDI Location:
*
java:comp/env/slee/facilities/trace
*
*
* @see javax.slee.management.TraceMBean
* @see javax.slee.management.TraceNotification
* @deprecated Replaced with {@link Tracer} objects that may be obtained from the
* {@link javax.slee.SbbContext} for SBBs, the {@link javax.slee.profile.ProfileContext}
* for profiles, or the {@link javax.slee.resource.ResourceAdaptorContext} for resource adaptor
* entities.
*/
public interface TraceFacility {
/**
* Get the current trace filter level set for a component identifier.
*
* This method is a non-transactional method.
* @param messageSource the component identifier.
* @return the current trace filter level for the identified component.
* @throws NullPointerException if id
is null
.
* @throws UnrecognizedComponentException if id
is not a recognizable
* ComponentID
object for the SLEE or it does not correspond
* with a component installed in the SLEE.
* @throws FacilityException if the trace level could not be obtained due to a
* system-level failure.
* @deprecated This method uses a ComponentID
to identify a notification
* source and thus was not flexible enough to be used by other objects in the
* SLEE. It has been replaced with {@link Tracer#getTraceLevel()}.
*/
public Level getTraceLevel(ComponentID messageSource)
throws NullPointerException, UnrecognizedComponentException, FacilityException;
/**
* Emit a trace notification containing the specified trace message. The trace
* notification will only be generated by the TraceMBean if the trace filter level
* of the specified component identifier does not cause the trace message to be filtered.
*
* This method is a non-transactional method.
* @param messageSource the identifier of the component that is emitting the trace message.
* @param traceLevel the logging level of the trace message.
* @param messageType a string denoting the type of the trace message.
* @param message the trace message.
* @param timestamp the time (in ms since January 1, 1970 UTC) that the message was generated.
* @throws NullPointerException if any parameter is null
.
* @throws IllegalArgumentException if traceLevel ==
{@link Level#OFF}.
* @throws UnrecognizedComponentException if messageSource
is not a recognizable
* ComponentID
object for the SLEE or it does not correspond
* with a component installed in the SLEE.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
* @deprecated This method uses a ComponentID
to identify a notification
* source and thus was not flexible enough to be used by other objects in the
* SLEE. It has been replaced with {@link Tracer#trace(TraceLevel, String)}.
*/
public void createTrace(ComponentID messageSource, Level traceLevel, String messageType, String message, long timestamp)
throws NullPointerException, IllegalArgumentException, UnrecognizedComponentException, FacilityException;
/**
* Emit a trace notification containing the specified trace message and cause throwable.
* The trace notification will only be generated by the TraceMBean if the trace filter level
* of the specified component identifier does not cause the trace message to be filtered.
*
* This method is a non-transactional method.
* @param messageSource the identifier of the component that is emitting the trace message.
* @param traceLevel the logging level of the trace message.
* @param messageType a string denoting the type of the trace message.
* @param message the trace message.
* @param cause the reason (if any) this trace message was generated.
* @param timestamp the time (in ms since January 1, 1970 UTC) that the message was generated.
* @throws NullPointerException if any parameter is null
.
* @throws IllegalArgumentException if traceLevel ==
{@link Level#OFF}.
* @throws UnrecognizedComponentException if messageSource
is not a recognizable
* ComponentID
object for the SLEE or it does not correspond
* with a component installed in the SLEE.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
* @deprecated This method uses a ComponentID
to identify a notification
* source and thus was not flexible enough to be used by other objects in the
* SLEE. It has been replaced with {@link Tracer#trace(TraceLevel, String, Throwable)}.
*/
public void createTrace(ComponentID messageSource, Level traceLevel, String messageType, String message, Throwable cause, long timestamp)
throws NullPointerException, IllegalArgumentException, UnrecognizedComponentException, FacilityException;
}