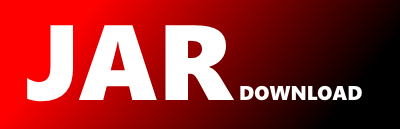
javax.slee.facilities.Tracer Maven / Gradle / Ivy
package javax.slee.facilities;
/**
* The Tracer
interface is used to emit trace messages. Trace messages
* that are emitted and which are not filtered by the effective trace filter level
* setting for the tracer cause a {@link javax.slee.management.TraceNotification}
* to be generated by the {@link javax.slee.management.TraceMBean}.
*
* A tracer is a named entity. Tracer names are case-sensitive and follow the Java
* hierarchical naming conventions. A tracer is considered to be an ancestor of
* another tracer if its name followed by a dot is a prefix of the descendant tracer's
* name. A tracer is considered to be a parent of a child tracer if there are no
* ancestors between itself and the descendant tracer. For example, the tracer named
* "com"
is the parent tracer of the tracer named "com.foo"
* and an ancestor of the tracer named "com.foo.bar"
. Each tracer name
* can be divided into one or more name components. Each name component identifies
* the name of the tracer at a particular level in the hierarchy. For example, the
* tracer named "com.foo.bar"
has three name components, "com"
,
* "foo"
, and "bar"
. Tracer name components must be at
* least one character in length. This means that the tracer name "com.foo"
* is a legal name, whereas "com..foo"
is not, as the middle name component
* of this name is zero-length.
*
* The empty string, ""
, denotes the root tracer. The root tracer sits at
* the top of a tracer hierarchy and is the ancestor of all tracers in the hierarchy.
* The root tracer always exists.
*
* All tracers may be assigned a {@link TraceLevel}. The Administrator uses the
* {@link javax.slee.management.TraceMBean} to change the trace level for a tracer.
* The effective trace level of a tracer is equal to its assigned trace level,
* or if the tracer has not been assigned a trace level then the effective trace level
* is equal to the trace level assigned to the nearest ancestor tracer. The root tracer
* always has an assigned trace level. The default trace level for all root tracers
* is {@link TraceLevel#INFO}.
*
* All tracers are implicitly associated with a {@link javax.slee.management.NotificationSource},
* an object that identifies the SLEE component or subsystem that the tracer was created
* for. For example, tracers created for an SBB via the
* {@link javax.slee.SbbContext#getTracer} method are associated with a notification
* source that identifies the SBB that the SbbContext
was created for and
* the service that that SBB belongs to. A tracer's NotificationSource
is
* included in any trace notifications generated by the TraceMBean
on behalf
* of that tracer.
*
* Tracers may be obtained by SBBs via the {@link javax.slee.SbbContext#getTracer}
* method. Tracers may be obtained by profiles via the {@link javax.slee.profile.ProfileContext#getTracer}
* method. Tracers for a resource adaptor entity may be obtained via the
* {@link javax.slee.resource.ResourceAdaptorContext#getTracer} method.
*
* A Tracer is non-transactional. The effects of operations invoked on it occur
* regardless of the state or outcome of any enclosing transaction.
* @since SLEE 1.1
* @see javax.slee.management.TraceMBean
* @see javax.slee.management.TraceNotification
*/
public interface Tracer {
/**
* Get the tracer name for this tracer.
* @return the tracer name.
*
* This method is a non-transactional method.
*/
public String getTracerName();
/**
* Get the name of this tracer's parent tracer. For example, if {@link #getTracerName()}
* returns , this method will return "com"
*
* This method is a non-transactional method.
* @return the name of the this tracer's parent tracer, or null
if this
* tracer is the root tracer.
*/
public String getParentTracerName();
/**
* Get the current effective trace level for this tracer.
*
* This method is a non-transactional method.
* @return the current effective trace level for this tracer.
* @throws FacilityException if the trace level could not be obtained due to a
* system-level failure.
*/
public TraceLevel getTraceLevel()
throws FacilityException;
/**
* Emit a trace message at the {@link TraceLevel#SEVERE} trace level. This method will only
* cause a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if {@link #isSevereEnabled} returns true
,
* otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String) trace(TraceLevel.SEVERE, message);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace message could not be emitted due to a system-level
* failure.
*/
public void severe(String message)
throws NullPointerException, FacilityException;
/**
* Emit a trace message with a cause throwable at the {@link TraceLevel#SEVERE} trace level.
* This method will only cause a {@link javax.slee.management.TraceNotification} to be generated
* by the {@link javax.slee.management.TraceMBean} if {@link #isSevereEnabled} returns
* true
, otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String, Throwable) trace(TraceLevel.SEVERE, message, cause);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void severe(String message, Throwable cause)
throws NullPointerException, FacilityException;
/**
* Determine if the current effective trace filter level for this tracer is sufficient to
* allow a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if a trace message was emitted at the
* {@link TraceLevel#SEVERE} trace level using this tracer.
*
* This method is a convenience method equivalent to:
*
{@link #isTraceable isTraceable}({@link TraceLevel#SEVERE})
*
* This method is a non-transactional method.
* @return true
if the current effective trace filter level for this tracer
* allows a trace notification to be generated for a trace message emitted at the
* TraceLevel.SEVERE
level, false
otherwise.
* @throws FacilityException if the result could not be obtained due to a system-level failure.
*/
public boolean isSevereEnabled()
throws FacilityException;
/**
* Emit a trace message at the {@link TraceLevel#WARNING} trace level. This method will only
* cause a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if {@link #isWarningEnabled} returns true
,
* otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String) trace(TraceLevel.WARNING, message);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void warning(String message)
throws NullPointerException, FacilityException;
/**
* Emit a trace message with a cause throwable at the {@link TraceLevel#WARNING} trace level.
* This method will only cause a {@link javax.slee.management.TraceNotification} to be generated
* by the {@link javax.slee.management.TraceMBean} if {@link #isWarningEnabled} returns
* true
, otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String, Throwable) trace(TraceLevel.WARNING, message, cause);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void warning(String message, Throwable cause)
throws NullPointerException, FacilityException;
/**
* Determine if the current effective trace filter level for this tracer is sufficient to
* allow a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if a trace message was emitted at the
* {@link TraceLevel#WARNING} trace level using this tracer.
*
* This method is a convenience method equivalent to:
*
{@link #isTraceable isTraceable}({@link TraceLevel#WARNING})
*
* This method is a non-transactional method.
* @return true
if the current effective trace filter level for this tracer
* allows a trace notification to be generated for a trace message emitted at the
* TraceLevel.WARNING
level, false
otherwise.
* @throws FacilityException if the result could not be obtained due to a system-level failure.
*/
public boolean isWarningEnabled()
throws FacilityException;
/**
* Emit a trace message at the {@link TraceLevel#INFO} trace level. This method will only
* cause a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if {@link #isInfoEnabled} returns true
,
* otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String) trace(TraceLevel.INFO, message);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void info(String message)
throws NullPointerException, FacilityException;
/**
* Emit a trace message with a cause throwable at the {@link TraceLevel#INFO} trace level.
* This method will only cause a {@link javax.slee.management.TraceNotification} to be generated
* by the {@link javax.slee.management.TraceMBean} if {@link #isInfoEnabled} returns
* true
, otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String, Throwable) trace(TraceLevel.INFO, message, cause);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void info(String message, Throwable cause)
throws NullPointerException, FacilityException;
/**
* Determine if the current effective trace filter level for this tracer is sufficient to
* allow a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if a trace message was emitted at the
* {@link TraceLevel#INFO} trace level using this tracer.
*
* This method is a convenience method equivalent to:
*
{@link #isTraceable isTraceable}({@link TraceLevel#INFO})
*
* This method is a non-transactional method.
* @return true
if the current effective trace filter level for this tracer
* allows a trace notification to be generated for a trace message emitted at the
* TraceLevel.INFO
level, false
otherwise.
* @throws FacilityException if the result could not be obtained due to a system-level failure.
*/
public boolean isInfoEnabled()
throws FacilityException;
/**
* Emit a trace message at the {@link TraceLevel#CONFIG} trace level. This method will only
* cause a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if {@link #isConfigEnabled} returns true
,
* otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String) trace(TraceLevel.CONFIG, message);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void config(String message)
throws NullPointerException, FacilityException;
/**
* Emit a trace message with a cause throwable at the {@link TraceLevel#CONFIG} trace level.
* This method will only cause a {@link javax.slee.management.TraceNotification} to be generated
* by the {@link javax.slee.management.TraceMBean} if {@link #isConfigEnabled} returns
* true
, otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String, Throwable) trace(TraceLevel.CONFIG, message, cause);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void config(String message, Throwable cause)
throws NullPointerException, FacilityException;
/**
* Determine if the current effective trace filter level for this tracer is sufficient to
* allow a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if a trace message was emitted at the
* {@link TraceLevel#CONFIG} trace level using this tracer.
*
* This method is a convenience method equivalent to:
*
{@link #isTraceable isTraceable}({@link TraceLevel#CONFIG})
*
* This method is a non-transactional method.
* @return true
if the current effective trace filter level for this tracer
* allows a trace notification to be generated for a trace message emitted at the
* TraceLevel.CONFIG
level, false
otherwise.
* @throws FacilityException if the result could not be obtained due to a system-level failure.
*/
public boolean isConfigEnabled()
throws FacilityException;
/**
* Emit a trace message at the {@link TraceLevel#FINE} trace level. This method will only
* cause a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if {@link #isFineEnabled} returns true
,
* otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String) trace(TraceLevel.FINE, message);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void fine(String message)
throws NullPointerException, FacilityException;
/**
* Emit a trace message with a cause throwable at the {@link TraceLevel#FINE} trace level.
* This method will only cause a {@link javax.slee.management.TraceNotification} to be generated
* by the {@link javax.slee.management.TraceMBean} if {@link #isFineEnabled} returns
* true
, otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String, Throwable) trace(TraceLevel.FINE, message, cause);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void fine(String message, Throwable cause)
throws NullPointerException, FacilityException;
/**
* Determine if the current effective trace filter level for this tracer is sufficient to
* allow a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if a trace message was emitted at the
* {@link TraceLevel#FINE} trace level using this tracer.
*
* This method is a convenience method equivalent to:
*
{@link #isTraceable isTraceable}({@link TraceLevel#FINE})
*
* This method is a non-transactional method.
* @return true
if the current effective trace filter level for this tracer
* allows a trace notification to be generated for a trace message emitted at the
* TraceLevel.FINE
level, false
otherwise.
* @throws FacilityException if the result could not be obtained due to a system-level failure.
*/
public boolean isFineEnabled()
throws FacilityException;
/**
* Emit a trace message at the {@link TraceLevel#FINER} trace level. This method will only
* cause a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if {@link #isFinerEnabled} returns true
,
* otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String) trace(TraceLevel.FINER, message);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void finer(String message)
throws NullPointerException, FacilityException;
/**
* Emit a trace message with a cause throwable at the {@link TraceLevel#FINER} trace level.
* This method will only cause a {@link javax.slee.management.TraceNotification} to be generated
* by the {@link javax.slee.management.TraceMBean} if {@link #isFinerEnabled} returns
* true
, otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String, Throwable) trace(TraceLevel.FINER, message, cause);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void finer(String message, Throwable cause)
throws NullPointerException, FacilityException;
/**
* Determine if the current effective trace filter level for this tracer is sufficient to
* allow a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if a trace message was emitted at the
* {@link TraceLevel#FINER} trace level using this tracer.
*
* This method is a convenience method equivalent to:
*
{@link #isTraceable isTraceable}({@link TraceLevel#FINER})
*
* This method is a non-transactional method.
* @return true
if the current effective trace filter level for this tracer
* allows a trace notification to be generated for a trace message emitted at the
* TraceLevel.FINER
level, false
otherwise.
* @throws FacilityException if the result could not be obtained due to a system-level failure.
*/
public boolean isFinerEnabled()
throws FacilityException;
/**
* Emit a trace message at the {@link TraceLevel#FINEST} trace level. This method will only
* cause a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if {@link #isFinestEnabled} returns true
,
* otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String) trace(TraceLevel.FINEST, message);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void finest(String message)
throws NullPointerException, FacilityException;
/**
* Emit a trace message with a cause throwable at the {@link TraceLevel#FINEST} trace level.
* This method will only cause a {@link javax.slee.management.TraceNotification} to be generated
* by the {@link javax.slee.management.TraceMBean} if {@link #isFinestEnabled} returns
* true
, otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String, Throwable) trace(TraceLevel.FINEST, message, cause);}
*
* This method is a non-transactional method.
* @param message the trace message.
* @throws NullPointerException if message
is null.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void finest(String message, Throwable cause)
throws NullPointerException, FacilityException;
/**
* Determine if the current effective trace filter level for this tracer is sufficient to
* allow a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if a trace message was emitted at the
* {@link TraceLevel#FINEST} trace level using this tracer.
*
* This method is a convenience method equivalent to:
*
{@link #isTraceable isTraceable}({@link TraceLevel#FINEST})
*
* This method is a non-transactional method.
* @return true
if the current effective trace filter level for this tracer
* allows a trace notification to be generated for a trace message emitted at the
* TraceLevel.FINEST
level, false
otherwise.
* @throws FacilityException if the result could not be obtained due to a system-level failure.
*/
public boolean isFinestEnabled()
throws FacilityException;
/**
* Determine if the current effective trace level for this tracer is sufficient to allow
* a {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if a trace message was emitted at the specified
* trace level using this tracer.
*
* An invocation of this method can be used as a guard around a trace invocation to avoid
* unnecessary computation of a trace message string that will ultimately be discarded.
*
* This method is a convenience method equivalent to:
*
!{@link #getTraceLevel()}.{@link TraceLevel#isHigherLevel isHigherLevel(level)}
*
* This method is a non-transactional method.
* @param level the trace level to test.
* @return true
if the current effective trace filter level for this tracer
* allows a trace notification to be generated for a trace message emitted at the
* specified trace level, false
otherwise.
* @throws NullPointerException if level
is null
.
* @throws FacilityException if the result could not be obtained due to a system-level failure.
*/
public boolean isTraceable(TraceLevel level)
throws NullPointerException, FacilityException;
/**
* Emit a trace message at the specified trace level. This method will only cause a
* {@link javax.slee.management.TraceNotification} to be generated by the
* {@link javax.slee.management.TraceMBean} if {@link #isTraceable isTraceable(level)}
* returns true
, otherwise it has no effect and returns silently.
*
* This method is equivalent to:
*
{@link #trace(TraceLevel, String, Throwable) trace(level, message, null);}
*
* This method is a non-transactional method.
* @param level the trace level of the message.
* @param message the trace message.
* @throws NullPointerException if either level
or message
is null.
* @throws IllegalArgumentException if level ==
{@link TraceLevel#OFF}.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void trace(TraceLevel level, String message)
throws NullPointerException, IllegalArgumentException, FacilityException;
/**
* Emit a trace message with a cause throwable at the specified trace level. This method
* will only cause a {@link javax.slee.management.TraceNotification} to be generated by
* the {@link javax.slee.management.TraceMBean} if {@link #isTraceable isTraceable(level)}
* returns true
, otherwise it has no effect and returns silently.
*
* This method is a non-transactional method.
* @param level the trace level of the message.
* @param message the trace message.
* @param cause the reason (if any) this trace messages was generated. May be null.
* @throws NullPointerException if either level
or message
is null.
* @throws IllegalArgumentException if level ==
{@link TraceLevel#OFF}.
* @throws FacilityException if the trace could not be generated due to a system-level failure.
*/
public void trace(TraceLevel level, String message, Throwable cause)
throws NullPointerException, IllegalArgumentException, FacilityException;
}