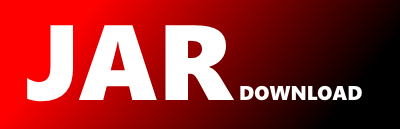
javax.slee.management.ServiceManagementMBean Maven / Gradle / Ivy
package javax.slee.management;
import javax.slee.ServiceID;
import javax.slee.InvalidStateException;
import javax.slee.InvalidArgumentException;
import javax.slee.UnrecognizedServiceException;
import javax.management.ObjectName;
/**
* The ServiceManagementMBean
interface defines Service-related
* management operations. Using the ServiceManagementMBean
a management
* client may get or change the operational states of Services, and get the set of
* services that are in a particular state.
*
* The JMX Object Name of a ServiceManagementMBean
object is specified by
* the {@link #OBJECT_NAME} constant. The Object Name can also be obtained by
* a management client via the {@link SleeManagementMBean#getServiceManagementMBean()} method.
*/
public interface ServiceManagementMBean {
/**
* The JMX Object Name string of the SLEE Service Management MBean, equal to the
* string "javax.slee.management:name=ServiceManagement".
* @since SLEE 1.1
*/
public static final String OBJECT_NAME = "javax.slee.management:name=ServiceManagement";
/**
* The notification type of {@link ServiceStateChangeNotification ServiceStateChange}
* notifications emitted by this MBean. The notification type is equal to the
* string "javax.slee.management.servicestatechange
".
* @since SLEE 1.1
*/
public static final String SERVICE_STATE_CHANGE_NOTIFICATION_TYPE =
"javax.slee.management.servicestatechange";
/**
* Get the current state of a Service.
* @param id the component identifier of the Service.
* @return the current state of the Service.
* @throws NullPointerException if id
is null
.
* @throws UnrecognizedServiceException if id
is not a
* recognizable ServiceID
for the SLEE or it does not
* correspond with a Service installed in the SLEE.
* @throws ManagementException if the state of the Service could not be
* obtained due to a system-level failure.
*/
public ServiceState getState(ServiceID id)
throws NullPointerException, UnrecognizedServiceException, ManagementException;
/**
* Get the set of services that are in a particular state.
* @param state the required state.
* @return an array of ServiceID
objects identifying the services
* that are in the specified state.
* @throws NullPointerException if state
is null
.
* @throws ManagementException if the set of services could not be obtained due
* to a system-level failure.
*/
public ServiceID[] getServices(ServiceState state)
throws NullPointerException, ManagementException;
/**
* Activate a Service. The Service must currently be in the
* {@link ServiceState#INACTIVE} state, and transitions to
* {@link ServiceState#ACTIVE} state during this method invocation.
* @param id the component identifier of the Service.
* @throws NullPointerException if id
is null
.
* @throws UnrecognizedServiceException if id
is not a
* recognizable ServiceID
for the SLEE or it does not
* correspond with a Service installed in the SLEE.
* @throws InvalidStateException if the current state of the Service is not
* ServiceState.INACTIVE
.
* @throws InvalidLinkNameBindingStateException if a resource adaptor entity
* link name binding required by an SBB in the Service does not exist or
* is bound to a resource adaptor entity that does not implement the
* resource adaptor type expected by the SBB.
* @throws ManagementException if the state of the Service could not be
* changed due to a system-level failure.
*/
public void activate(ServiceID id)
throws NullPointerException, UnrecognizedServiceException,
InvalidStateException, InvalidLinkNameBindingStateException,
ManagementException;
/**
* Activate a set of Services. All Services in the set must currently be in the
* {@link ServiceState#INACTIVE} state, and transition to
* {@link ServiceState#ACTIVE} state during this method invocation.
* @param ids a set of component identifiers of the Services to be activated.
* @throws NullPointerException if ids
is null
.
* @throws InvalidArgumentException if ids
is zero-length, or contains
* null
or duplicate elements.
* @throws UnrecognizedServiceException if any member of ids
is not a
* recognizable ServiceID
for the SLEE or does not correspond
* with a Service installed in the SLEE.
* @throws InvalidStateException if the current state of any of the Services
* identified by ids
is not ServiceState.INACTIVE
.
* @throws InvalidLinkNameBindingStateException if a resource adaptor entity
* link name binding required by an SBB in any of the Services does not exist or
* is bound to a resource adaptor entity that does not implement the
* resource adaptor type expected by the SBB.
* @throws ManagementException if the state of any Service could not be
* changed due to a system-level failure. In the case of such an error
* the state of all Services identified in ids
remains unchanged.
*/
public void activate(ServiceID[] ids)
throws NullPointerException, InvalidArgumentException,
UnrecognizedServiceException, InvalidStateException,
InvalidLinkNameBindingStateException, ManagementException;
/**
* Deactivate a Service. The Service must currently be in the
* {@link ServiceState#ACTIVE} state, and transitions to
* {@link ServiceState#STOPPING} state during this method invocation.
* @param id the component identifier of the Service.
* @throws NullPointerException if id
is null
.
* @throws UnrecognizedServiceException if id
is not a
* recognizable ServiceID
for the SLEE or it does not
* correspond with a Service installed in the SLEE.
* @throws InvalidStateException if the current state of the Service is not
* ServiceState.ACTIVE
.
* @throws ManagementException if the state of the Service could not be
* changed due to a system-level failure.
*/
public void deactivate(ServiceID id)
throws NullPointerException, UnrecognizedServiceException,
InvalidStateException, ManagementException;
/**
* Deactivate a set of Services. All Services in the set must currently be in the
* {@link ServiceState#ACTIVE} state, and transition to
* {@link ServiceState#STOPPING} state during this method invocation.
* @param ids a set of component identifiers of Services to be deactivated.
* @throws NullPointerException if ids
is null
.
* @throws InvalidArgumentException if ids
is zero-length, or contains
* null
or duplicate elements.
* @throws UnrecognizedServiceException if any member of ids
is not a
* recognizable ServiceID
for the SLEE or does not correspond
* with a Service installed in the SLEE.
* @throws InvalidStateException if the current state of any of the Services
* identified by ids
is not ServiceState.ACTIVE
.
* @throws ManagementException if the state of any Service could not be
* changed due to a system-level failure. In the case of such an error
* the state of all Services identified in ids
remains unchanged.
*/
public void deactivate(ServiceID[] ids)
throws NullPointerException, InvalidArgumentException,
UnrecognizedServiceException, InvalidStateException,
ManagementException;
/**
* Deactivate one Service and activate another Service. The first Service
* must currently be in the {@link ServiceState#ACTIVE} state, and transitions
* to {@link ServiceState#STOPPING} state during this method invocation. The
* second Service must be in the {@link ServiceState#INACTIVE} state and
* transitions to the {@link ServiceState#ACTIVE} state during this method
* invocation.
* @param deactivateID the component identifier of the Service to be deactivated.
* @param activateID the component identifier of the Service to be activated.
* @throws NullPointerException if either argument is null
.
* @throws InvalidArgumentException if deactivateID
and
* activateID
identify the same Service.
* @throws UnrecognizedServiceException if either argument is not a recognizable
* ServiceID
for the SLEE or does not correspond with a Service
* installed in the SLEE.
* @throws InvalidStateException if the current state of the Service identified
* by deactivateID
is not ServiceState.ACTIVE
or the
* the current state of the Service identified by activateID
is
* not ServiceState.INACTIVE
.
* @throws InvalidLinkNameBindingStateException if a resource adaptor entity
* link name binding required by an SBB in the activating Service does not exist
* or is bound to a resource adaptor entity that does not implement the
* resource adaptor type expected by the SBB.
* @throws ManagementException if the state of either Service could not be
* changed due to a system-level failure. In the case of such an error
* the state of both Services remains unchanged.
*/
public void deactivateAndActivate(ServiceID deactivateID, ServiceID activateID)
throws NullPointerException, InvalidArgumentException,
UnrecognizedServiceException, InvalidStateException,
InvalidLinkNameBindingStateException, ManagementException;
/**
* Deactivate one set of Services and activate another set of Services.
* All Services in the first set must currently be in the {@link ServiceState#ACTIVE}
* state, and transition to {@link ServiceState#STOPPING} state during this method
* invocation. All Services in the second set must be in the {@link ServiceState#INACTIVE}
* state and transition to the {@link ServiceState#ACTIVE} state during this method
* invocation.
* @param deactivateIDs a set of component identifiers of Services to be deactivated.
* @param activateIDs a set of component identifiers of Services to be activated.
* @throws NullPointerException if either argument is null
.
* @throws InvalidArgumentException if either argument is zero-length, contains
* null
or duplicate elements, or a Service identified by a
* a component identifier in deactivateIDs
is the same as a Service
* identified by a component identifier in activateIDs
.
* @throws UnrecognizedServiceException if any member of deactivateIDs
* or activateIDs
is not a recognizable ServiceID
* for the SLEE or does not correspond with a Service installed in the SLEE.
* @throws InvalidStateException if the current state of any of the Services
* identified by deactivateIDs
is not ServiceState.ACTIVE
* or the the current state of any of the Services identified by
* activateIDs
is not ServiceState.INACTIVE
.
* @throws InvalidLinkNameBindingStateException if a resource adaptor entity
* link name binding required by an SBB in any of the activating Services does not
* exist or is bound to a resource adaptor entity that does not implement the
* resource adaptor type expected by the SBB.
* @throws ManagementException if the state of any of the Services could not be
* changed due to a system-level failure. In the case of such an error
* the state of all Services identified in deactivateIDs
and
* activateIDs
remains unchanged.
*/
public void deactivateAndActivate(ServiceID[] deactivateIDs, ServiceID[] activateIDs)
throws NullPointerException, InvalidArgumentException,
UnrecognizedServiceException, InvalidStateException,
InvalidLinkNameBindingStateException, ManagementException;
/**
* Get the JMX Object Name of a {@link ServiceUsageMBean} object for a Service.
*
* The JMX Object name of the Service Usage MBean is composed of at least:
*
* - the {@link ServiceUsageMBean#BASE_OBJECT_NAME base name} which specifies the
* domain and type of the MBean
*
- the {@link ServiceUsageMBean#SERVICE_NAME_KEY} property, with a value equal
* to
id.getName()
* - the {@link ServiceUsageMBean#SERVICE_VENDOR_KEY} property, with a value equal
* to
id.getVendor()
* - the {@link ServiceUsageMBean#SERVICE_VERSION_KEY} property, with a value equal
* to
id.getVersion()
*
* @param id the component identifier of the Service.
* @return the Object Name of a ServiceUsageMBean
object for the
* specified Service.
* @throws NullPointerException if id
is null
.
* @throws UnrecognizedServiceException if id
is not a
* recognizable ServiceID
for the SLEE or it does not
* correspond with a Service installed in the SLEE.
* @throws ManagementException if the Object Name could not be obtained due to a
* system-level failure.
*/
public ObjectName getServiceUsageMBean(ServiceID id)
throws NullPointerException, UnrecognizedServiceException, ManagementException;
}