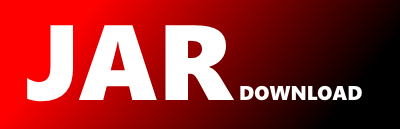
javax.slee.profile.ProfileTable Maven / Gradle / Ivy
package javax.slee.profile;
import java.util.Collection;
import javax.slee.SLEEException;
import javax.slee.TransactionRequiredLocalException;
import javax.slee.CreateException;
/**
* The ProfileTable
interface defines common operations that may be performed
* by SLEE components on profile tables. This interface is extended by a profile
* specification that defines static query methods on its Profile Table Interface.
*
* Static query methods have the following design pattern:
*
public java.util.Collection query<query-name>(<query-args...>);
*
* where:
*
* query-name
is the name of a static query defined in the profile
* specification's deployment descriptor, with the first letter uppercased.
*
* query-args
are zero or more arguments that match the quantity,
* ordering, and type of the query parameters defined for the query in the profile
* specification's deployment descriptor.
*
* Static query methods are mandatory transactional, and will throw a
* javax.slee.TransactionRequiredLocalException
if invoked without a valid
* transaction context. These methods may also throw a javax.slee.SLEEException
* if the query could not be successfully executed due to a system-level failure.
* @since SLEE 1.1
*/
public interface ProfileTable {
/**
* Create a new profile with the specified name in the profile table represented by this
* ProfileTable
object. The ProfileLocalObject
returned by this
* method may be safely typecast to the Profile Local Interface defined by the profile
* specification of the profile table. The invoking client may use the
* ProfileLocalObject
to interact with the new profile in the same transaction
* context as it was created, for example, to set the values of any profile CMP fields
* prior to the profile creation being committed.
*
* This method is a mandatory transactional method.
* @param profileName the name of the new profile. The name must be unique within the
* scope of the profile table.
* @return a Profile Local Interface object.
* @throws NullPointerException if profileName
is null
.
* @throws IllegalArgumentException if profileName
is zero-length or contains
* illegal characters.
* @throws TransactionRequiredLocalException if this method is invoked without a valid
* transaction context.
* @throws ReadOnlyProfileException if the profile table's profile specification has
* enforced a read-only SLEE component view of profiles.
* @throws ProfileAlreadyExistsException if a profile with the same name already exists
* in the profile table.
* @throws CreateException if the {@link javax.slee.profile.Profile#profilePostCreate()}
* method of the profile object invoked to create the profile throws this
* exception, it is propagated to the caller of this method.
* @throws SLEEException if the profile could not be created due to a system-level failure.
*/
public ProfileLocalObject create(String profileName)
throws NullPointerException, IllegalArgumentException, TransactionRequiredLocalException,
ReadOnlyProfileException, ProfileAlreadyExistsException, CreateException,
SLEEException;
/**
* Find an existing profile with the specified profile name within the profile table
* represented by this ProfileTable
object. The ProfileLocalObject
* returned by this method may be safely typecast to the Profile Local Interface defined
* by the profile specification of the profile table.
*
* This method is a mandatory transactional method.
* @param profileName the name of the profile to find.
* @return a Profile Local Interface object for the found profile, or null
* if a profile with the specified name does not exist in the profile table.
* @throws NullPointerException if profileName
is null
.
* @throws TransactionRequiredLocalException if this method is invoked without a valid
* transaction context.
* @throws SLEEException if the profile could not be found due to a system-level failure.
*/
public ProfileLocalObject find(String profileName)
throws NullPointerException, TransactionRequiredLocalException, SLEEException;
/**
* Find all profiles within the profile table represented by this ProfileTable
* object. The profile table's default profile is not included in the collection returned
* by this method.
*
* The ProfileLocalObject
s contained in the collection returned by this method may
* be safely typecast to the Profile Local Interface defined by the profile specification of
* the profile table.
*
* The collection returned by this method is immutable, i.e. elements may not be added to or
* removed from the collection. Any attempt to modify it, either directly or indirectly,
* will result in a java.lang.UnsupportedOperationException
being thrown.
*
* This method is a mandatory transactional method.
* @return a read-only collection of {@link ProfileLocalObject} objects, one for each profile
* that exists in the profile table.
* @throws TransactionRequiredLocalException if this method is invoked without a valid
* transaction context.
* @throws SLEEException if the profiles could not be found due to a system-level failure.
*/
public Collection findAll()
throws TransactionRequiredLocalException, SLEEException;
/**
* Find all profiles within the profile table represented by this ProfileTable
* object where the specified profile attribute is set to the specified value. The type of
* the attribute must be a primitive type, an object wrapper of a primitive type,
* java.lang.String
, or javax.slee.Address
.
*
* The profile table's default profile is not considered when determining matching profiles,
* and the returned collection will never contain a Profile Local Interface object for the
* default profile.
*
* The ProfileLocalObject
s contained in the collection returned by this method may
* be safely typecast to the Profile Local Interface defined by the profile specification of
* this profile table.
*
* The collection returned by this method is immutable, i.e. elements may not be added to or
* removed from the collection. Any attempt to modify it, either directly or indirectly,
* will result in a java.lang.UnsupportedOperationException
being thrown.
*
* This method is a mandatory transactional method.
*
* This method can only be invoked on profile tables created from SLEE 1.1 profile
* specifications. Attempting to invoke it on a profile table created from a SLEE 1.0
* profile specification causes a SLEEException
to be thrown.
* @param attributeName the name of the profile's attribute to check.
* @param attributeValue the value to compare the attribute with.
* @return a read-only collection of {@link ProfileLocalObject} objects, one for each profile
* that exists in the profile table where the specified attribute of the profile equals
* the specified value.
* @throws NullPointerException if either argument is null
.
* @throws IllegalArgumentException if attributeName
does not identify a profile
* attribute defined by the profile specification of the profile table or if the
* type of the attribute is not one of the permitted types, or if the type of
* attributeValue
does not match the type of the attribute.
* @throws TransactionRequiredLocalException if this method is invoked without a valid
* transaction context.
* @throws SLEEException if the profiles could not be found due to a system-level failure,
* or if an attempt is made to invoke this method on a profile table created from a
* SLEE 1.0 profile specification.
*/
public Collection findProfilesByAttribute(String attributeName, Object attributeValue)
throws NullPointerException, IllegalArgumentException,
TransactionRequiredLocalException, SLEEException;
/**
* Find a profile contained in the profile table represented by this ProfileTable
* object where the specified profile attribute is set to the specified value. The type of
* the attribute must be a primitive type, an object wrapper of a primitive type,
* java.lang.String
, or javax.slee.Address
.
*
* If this method finds more than one matching profile, the SLEE may arbitrarily return any
* one of the matching profiles as the result. The profile table's default profile is not
* considered when determining matching profiles.
*
* The ProfileLocalObject
returned by this method may be safely typecast to the
* Profile Local Interface defined by the profile specification of this profile table.
*
* This method is a mandatory transactional method.
*
* This method can only be invoked on profile tables created from SLEE 1.1 profile
* specifications. Attempting to invoke it on a profile table created from a SLEE 1.0
* profile specification causes a SLEEException
to be thrown.
* @param attributeName the name of the profile's attribute to check.
* @param attributeValue the value to compare the attribute with.
* @return a {@link ProfileLocalObject} for a profile where the specified attribute equals
* the specified value, or null
if no matching profile was found.
* @throws NullPointerException if either argument is null
.
* @throws IllegalArgumentException if attributeName
does not identify a profile
* attribute defined by the profile specification of the profile table or if the
* type of the attribute is not one of the permitted types, or if the type of
* attributeValue
does not match the type of the attribute.
* @throws TransactionRequiredLocalException if this method is invoked without a valid
* transaction context.
* @throws SLEEException if the profiles could not be found due to a system-level failure,
* or if an attempt is made to invoke this method on a profile table created from a
* SLEE 1.0 profile specification.
*/
public ProfileLocalObject findProfileByAttribute(String attributeName, Object attributeValue)
throws NullPointerException, IllegalArgumentException,
TransactionRequiredLocalException, SLEEException;
/**
* Remove an existing profile with the specified profile name from the profile table
* represented by this ProfileTable
object.
*
* This method is a mandatory transactional method.
* @param profileName the name of the profile to remove.
* @return true
if the specified profile existed in the profile table and
* was removed, false
if the specified profile was not found in the
* profile table.
* @throws NullPointerException if profileName
is null
.
* @throws ReadOnlyProfileException if the profile table's profile specification has
* enforced a read-only SLEE component view of profiles.
* @throws TransactionRequiredLocalException if this method is invoked without a valid
* transaction context.
* @throws SLEEException if the profile could not be removed due to a system-level failure.
*/
public boolean remove(String profileName)
throws NullPointerException, ReadOnlyProfileException,
TransactionRequiredLocalException, SLEEException;
}